Working with colours, Listing 8 – ETC Unison Mosaic Designer v1.11.0 User Manual
Page 231
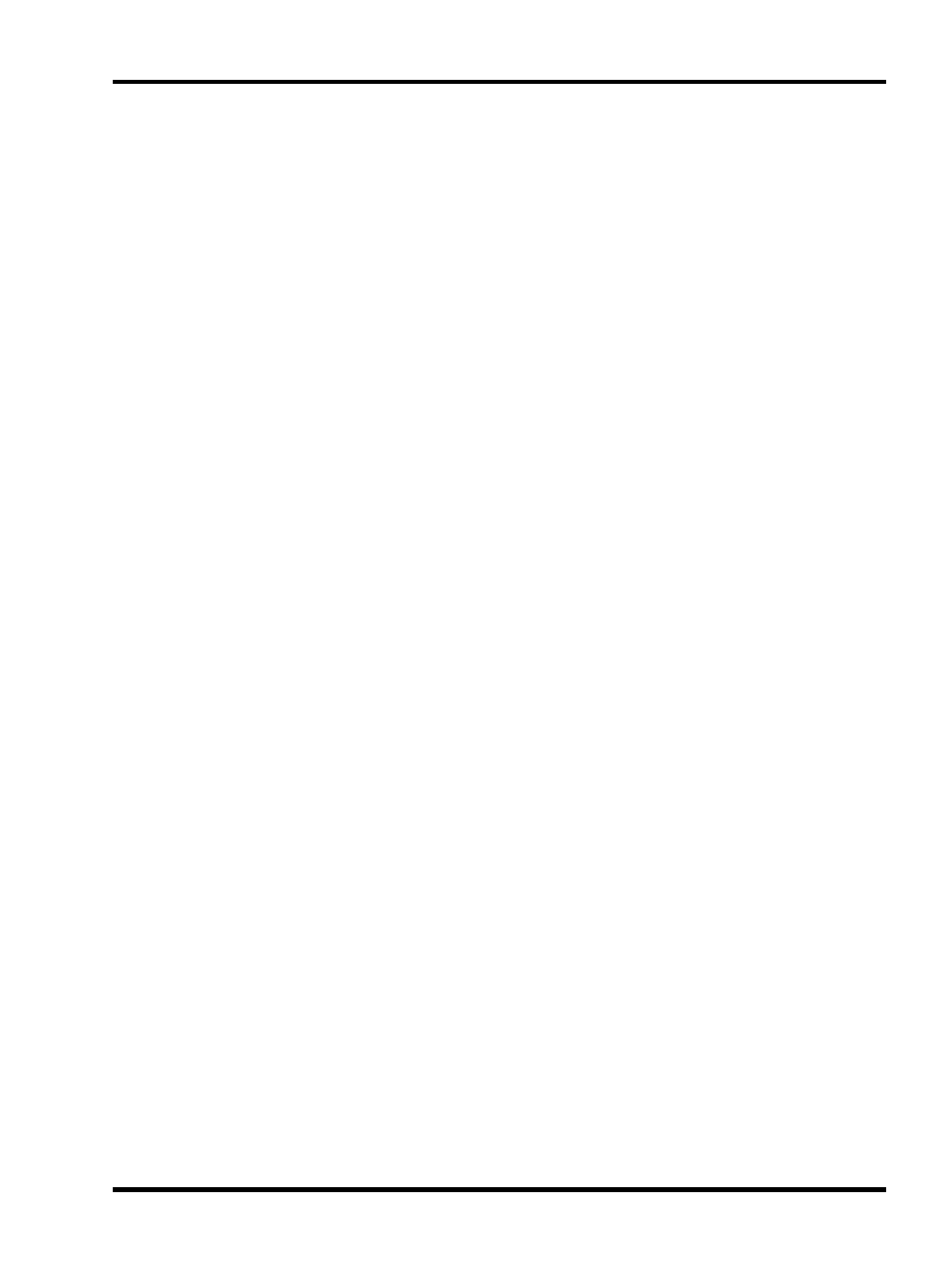
Custom Preset Programming Guide
-- get the band in which this pixel falls
local band = math.floor(x/total_band_width)
-- get the fraction through the effect
local t = frame/frames
-- get the height of the band in which this pixel falls
local band_height = (math.sin((band/bands+t)*math.pi*2)+1)/2
-- adjust y to be relative to the center of the effect
y = y-(height/2)+0.5
-- decide if this pixel is inside the band
local h = math.abs(y)/(height/2)
if (h <= band_height) then
return rainbow(band/bands+t)
else
-- offset hue by quarter
return rainbow((band/bands+t)+0.25)
end
end
We have defined a new function, rainbow, which returns a fully saturated r,g,b value for a given hue. This func-
tion is then called with different arguments depending on whether on not a pixel falls inside or outside of a band.
User-defined functions can be used whenever you want to use a similar piece of code in multiple places with dif-
fering arguments.
Running this script, you will see that the bands are now coloured with a rainbow which changes over time, and
the area above and below the band is filled with a colour that is pi/2 radians out of phase with the band's colour.
Working with colours
Working with colours as 3 separate components can produce a wide variety of effects, but sometimes it is more
convenient to treat a colour as a single entity. We can do that with the colour library.
To create a variable of type colour, call colour.new(), passing in three values between 0 and 255 which rep-
resent the red, green and blue components of the colour, i.e:
local c = colour.new(255,0,0)
The variable c has the type colour and represents red. Colours have three properties, red, green and blue,
which can be used to access and alter that colour. Here is a simple example using the colour type:
Listing 8
function pixel(frame,x,y)
local c = colour.new(255,0,0)
return c.red,c.green,c.blue
end
This fills every pixel of every frame with red.
- 231 -