Functions – ETC Unison Mosaic Designer v1.11.0 User Manual
Page 217
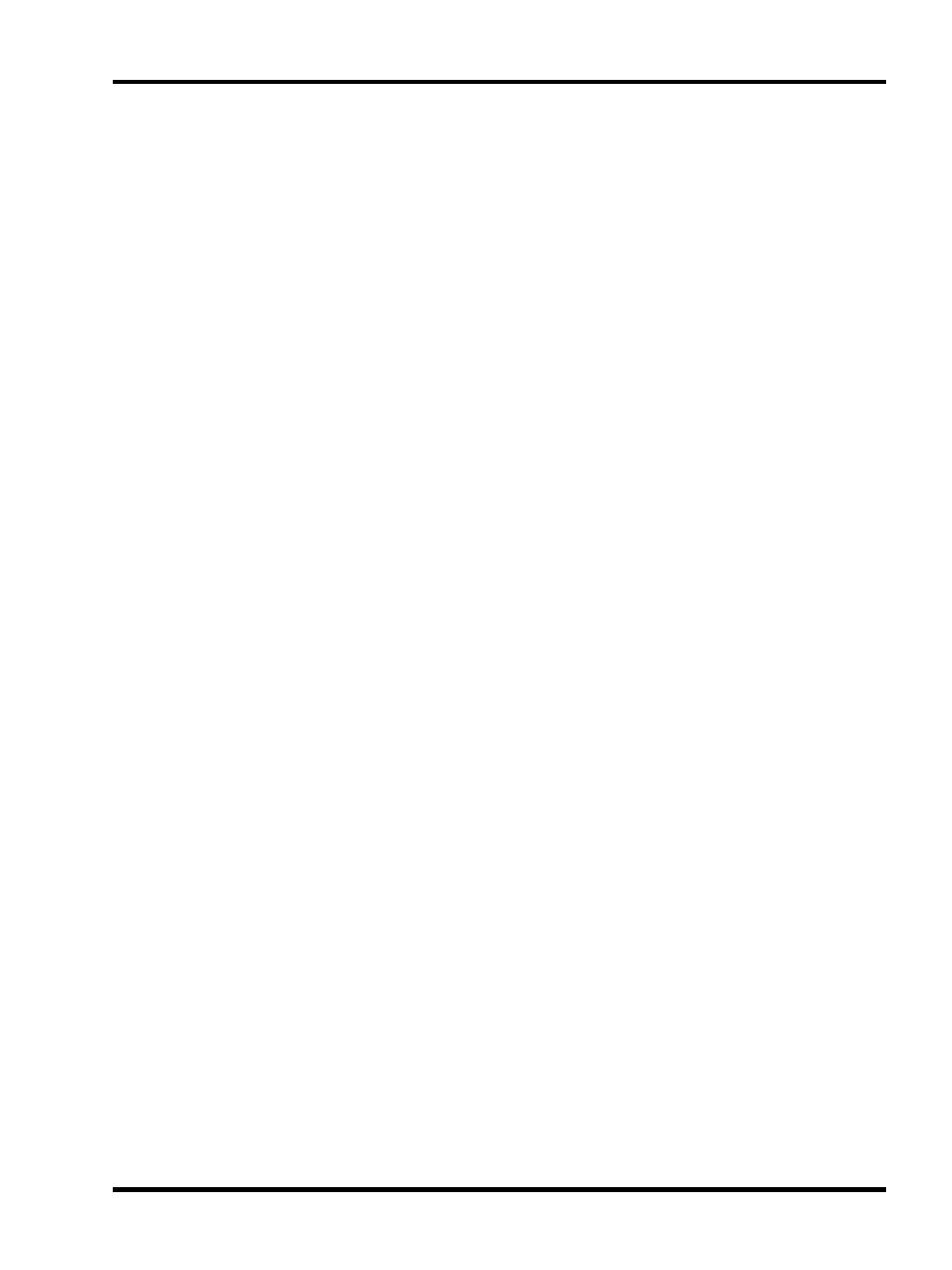
Trigger Programming Guide
firstTable = {} -- creates an empty table
secondTable = { 5,3,9,7 } -- a table with 4 entries
You can then access entries within the table by indexing into it - signified by square brackets. The number within
the square brackets identified which entry within the table you want to use or modify.
x = secondTable[3] -- x now equals 9 (3rd entry)
firstTable[1] = 5 -- entry 1 now has value 5
firstTable[7] = 3 -- entry 7 now has value 3
x = firstTable[1] + firstTable[7] -- x now equals 5 + 3
Note that we are allowed to assign values to entries within the table without doing anything special to change the
size of the table. We can keep adding elements to the table as needed and Lua will take care of it for us. This
makes it possible to write scripts using tables that will work regardless of how many entries there are in the table
(e.g. a list of 4 timeline numbers or of 40).
Tables are particularly powerful when used together with the loops we looked at in the previous section. For
example if I have a table of numbers and I wanted to find the smallest then I could use the following script:
numbers = { 71,93,22,45,16,33,84 }
smallest = 10000 -- initialise with large number
i = 1 -- use to count loops
while numbers[i] do
if numbers[i] < smallest then
smallest = numbers[i]
end
i = i+1
end
This is our first really functional piece of script and there are a couple of things worth noting.
l
The first entry in a table is accessed using the number one (i.e. myTable[1]). This may seem obvious -
but some other programming languages start counting from zero.
l
As we increment the variable i each time around the loop this means we will be looking at a different entry
in the table each time around. The test at the start of my while loop is written to work regardless of how
many entries there are in the table. When you use a table entry in a test like this then it will be true as long
as the entry has some value (even if the value is zero) and false if there is no value there at all.
Functions
Within script there are a whole range of pre-defined operations that you can call when writing your own scripts.
Some of these are provided by the Lua language and are fully described in its documentation. Others have been
provided by ETC to allow you to interact with the Controller from script and are fully described in the manual.
They are all called functions and accessed using a similar syntax. For example:
x = math.random(1,100)
This will assign variable x a value that is a random number between 1 and 100. The function math.random() is
a standard function provided by Lua and we can control its behaviour by passing in an argument - in this case the
values 1 and 100 to tell it the range within which we want our random number to fall.
t = 5
- 217 -