Comments, Variables, Arithmetic – ETC Unison Mosaic Designer v1.11.0 User Manual
Page 215: Flow of control
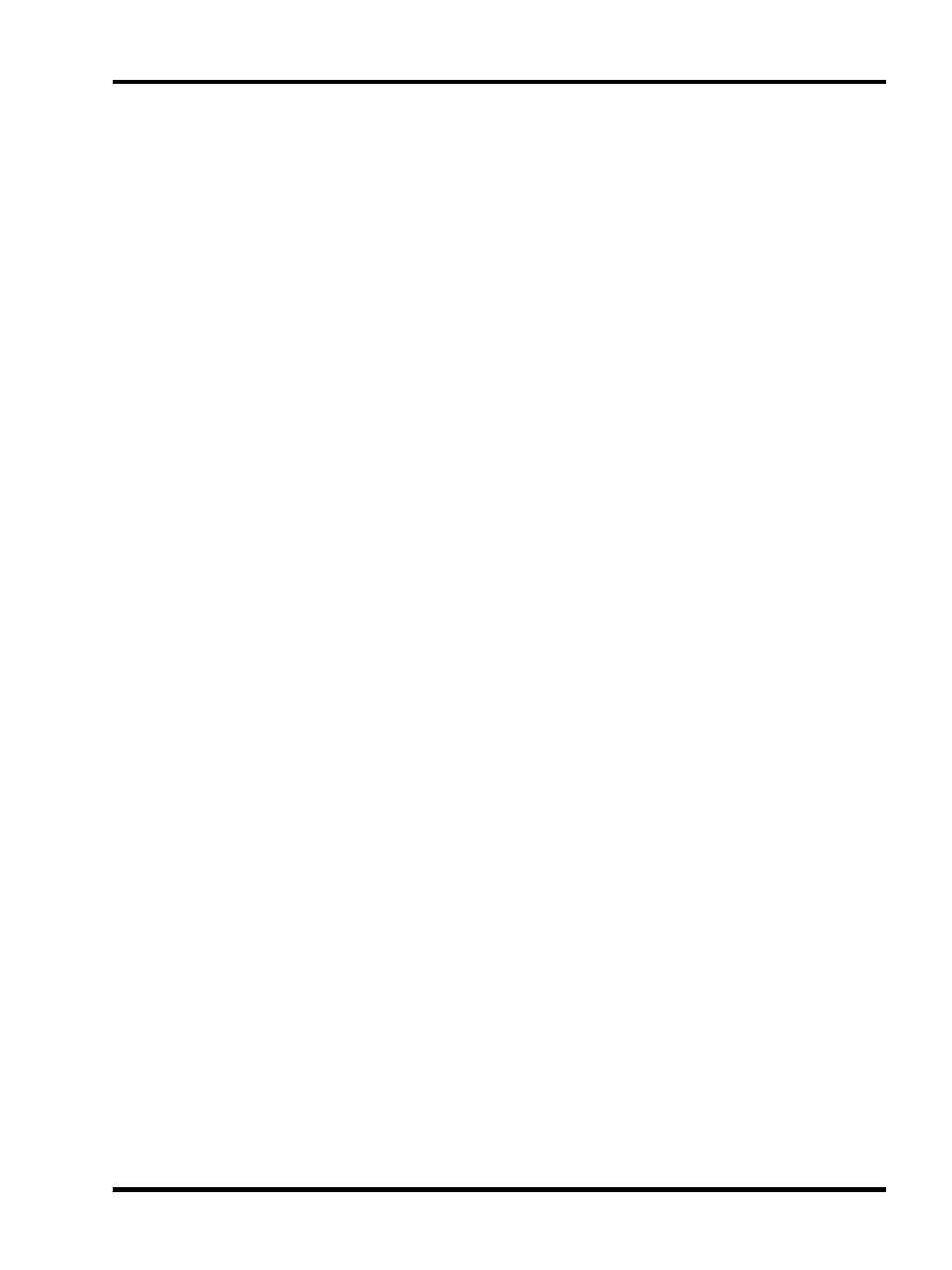
Trigger Programming Guide
Comments
It is good practice to include readable comments in your scripts so that you (or anyone else) will be able to easily
tell what you were aiming to achieve. In Lua everything after two dashes on a line is treated as a comment.
-- This is a comment
This = is + not - a * comment -- but this is!
The whole point of comments is that they have no effect on the behaviour of the script. But I am introducing them
first so that I can use them within the examples that follow.
Variables
If you want to store a piece of data - whether it is a number, some text or just true or false - then you use a vari-
able. You create a variable simply by giving it a name and using it in your script. A variable can store any type of
data just by assigning it.
firstVariable = 10 -- assign a number
anotherVariable = "Some text" -- assign a string
When you next use these names then they will have the values that you assigned to them:
nextVariable = firstVariable + 5 -- value of nextVariable will be 15
Note that names are case-sensitive (i.e. capitals matter!), and once you have named a variable once then any
time you use the same name you will be referring to the same variable - in programming terms it is global. This
even applies across different scripts - so you can assign a number to a variable called bob in one script and then
use the number in another script by referencing bob.
One of the most common errors when writing scripts is trying to use a named variable before it has been assigned
a value - this will result in an error when the script is run. It is also very easy to use the same name in two different
places and not realise that you are actually reusing a single variable. (There is a way of dealing with this for
names you want to reuse that we will touch on later.)
Arithmetic
Scripts will often need to do some arithmetic - even if it is something very basic like keeping a counter of how
many times it is run:
myCount = myCount + 1
All of the standard arithmetic operations are available. There is also a library of mathematical functions available
should it be required, which includes things like random number generators.
Flow of Control
In most scripts there will be one or more points where you want to make choices. Lua provides four useful struc-
tures for this. The most common is if, where you can choose which path to take through the script by performing
tests.
if myNumber < 5 then
- 215 -