AMETEK Lx Series II Programming Manual User Manual
Page 52
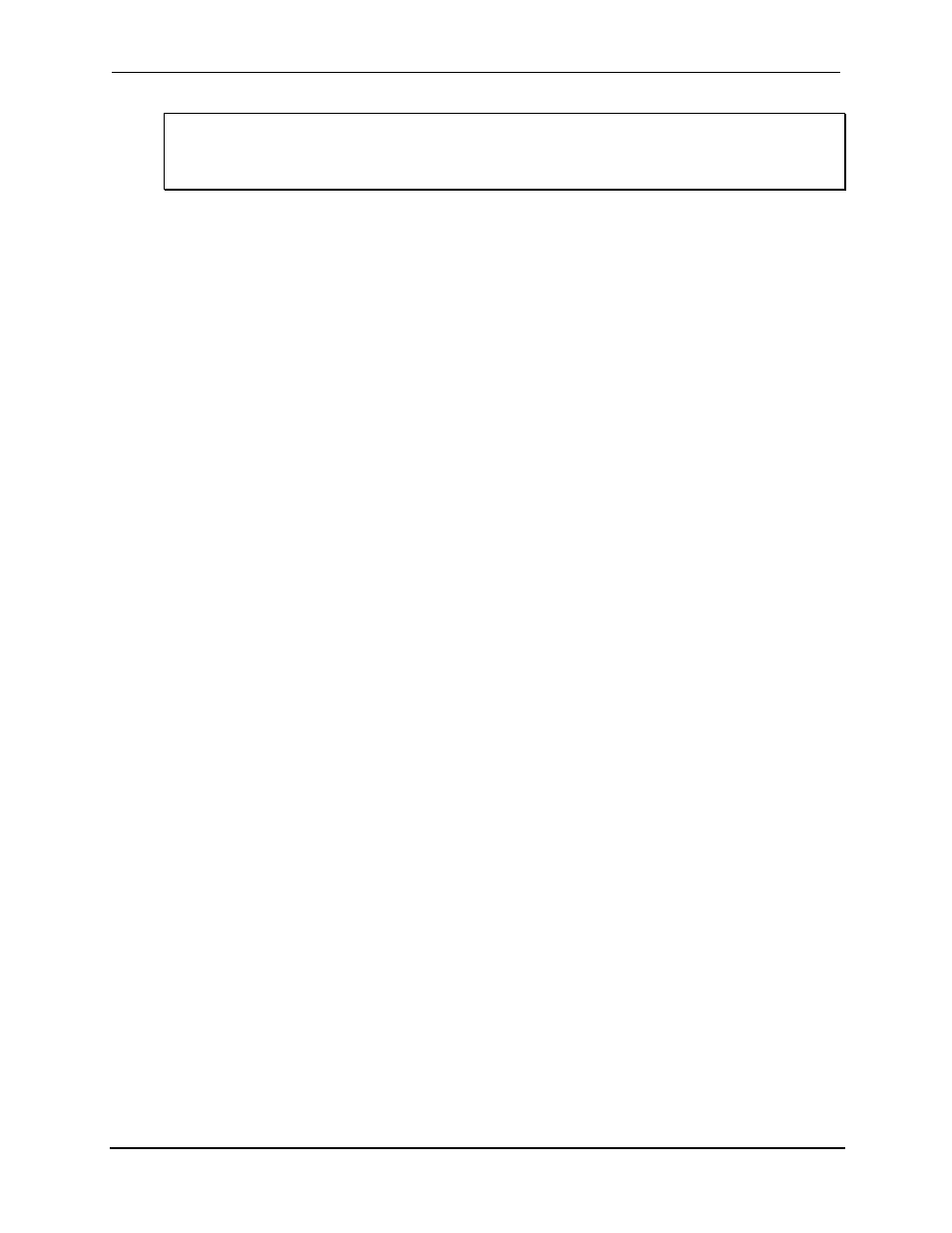
Programming Manual
Lx \ Ls Series II
48
Syntax
MEASure:ARRay:MODe
Parameters
BIN | ASCii
Examples
MEAS:ARR:MOD ASC
Related Commands
MEAS:ARR:VOLT
MEAS:ARR:CURR
Note: The MEAS:ARR:MOD command is provided to allow waveform data transfers in ASCII on
DBCS versions of MS Windows. Examples of DBCS versions are Chinese, Korean, Japanese
etc. On most Windows versions, the binary mode can be used as it reduces the amount of data
transferred and thus provides better throughput.
The ASCII mode will double the number of characters transferred so provisions for a larger
receive buffer on the PC may have to be made. On the Lx/Ls, the full acquisition data size that
can be sent with one command in BIN mode is 16KB, in ASC mode 32KB.
The binary data must be converted to a single precision floating point notation. Sample VB6 code
is shown on the next page.
Conversion function sample VB6. Converting waveform data from either transfer mode to a single
precision value can be accomplished using the following sample routine:
Public Function StringToIEEEFloat(ByVal sData As String, ByVal bAsciiMode As Boolean) As
Single
'=============================================================
'bAsciiMode flag is used if data is received as 8 ascii chars
'representing Hex 0-9,A-F. If bAsciiMode flag is false, then
'data is process as 4 char representing a byte each. Ascii
'mode is needed for DCBS windows
'=============================================================
Dim i As Integer
Dim j As Integer
Dim iChar As Integer
Dim expo As Long
Dim mantisse As Long
Dim expo_val As Variant
Dim mant_f As Single
Dim c(3) As Long 'Must use 32 bit integers to allow for
'intermediate result of 24 bit shift
Dim sign As Boolean
'=============================================================
Const MANT_MAX = &H7FFFFF
Const EXPO_MAX = 2 ^ 126
'=============================================================
On Error GoTo FloatConvError
If bAsciiMode Then
'Retrieve ASC values from eight hex byte input data
sData = UCase(sData)
For i = 0 To 3
c(i) = 0
For j = 0 To 1
iChar = AscB(Mid$(sData, i * 2 + j + 1, 1)) - 48
If iChar > 9 Then iChar = iChar - 7
c(i) = c(i) * 16 * j + iChar
Next j
Next i
Else
'Retrieve ASC values from four byte input data
'Note: Don't use ASCB or ASCW functions as results will differ
'based on character sets, even on non DCBS Windows
'Retrieve ASC values from four byte input data
For i = 0 To 3
c(i) = Asc(Mid$(sData, i + 1, 1))
Next i
End If
'Get sign bit
sign = ((c(0) And &H80) = &H80)
'Get exponent value less sign bit
expo = (c(0) And &H7F) * 2
'Pick up exponent sign
If (c(1) And &H80) = &H80 Then expo = expo Or 1