Sample program, Sample program -11 – Sensaphone SCADA 3000 Users manual User Manual
Page 197
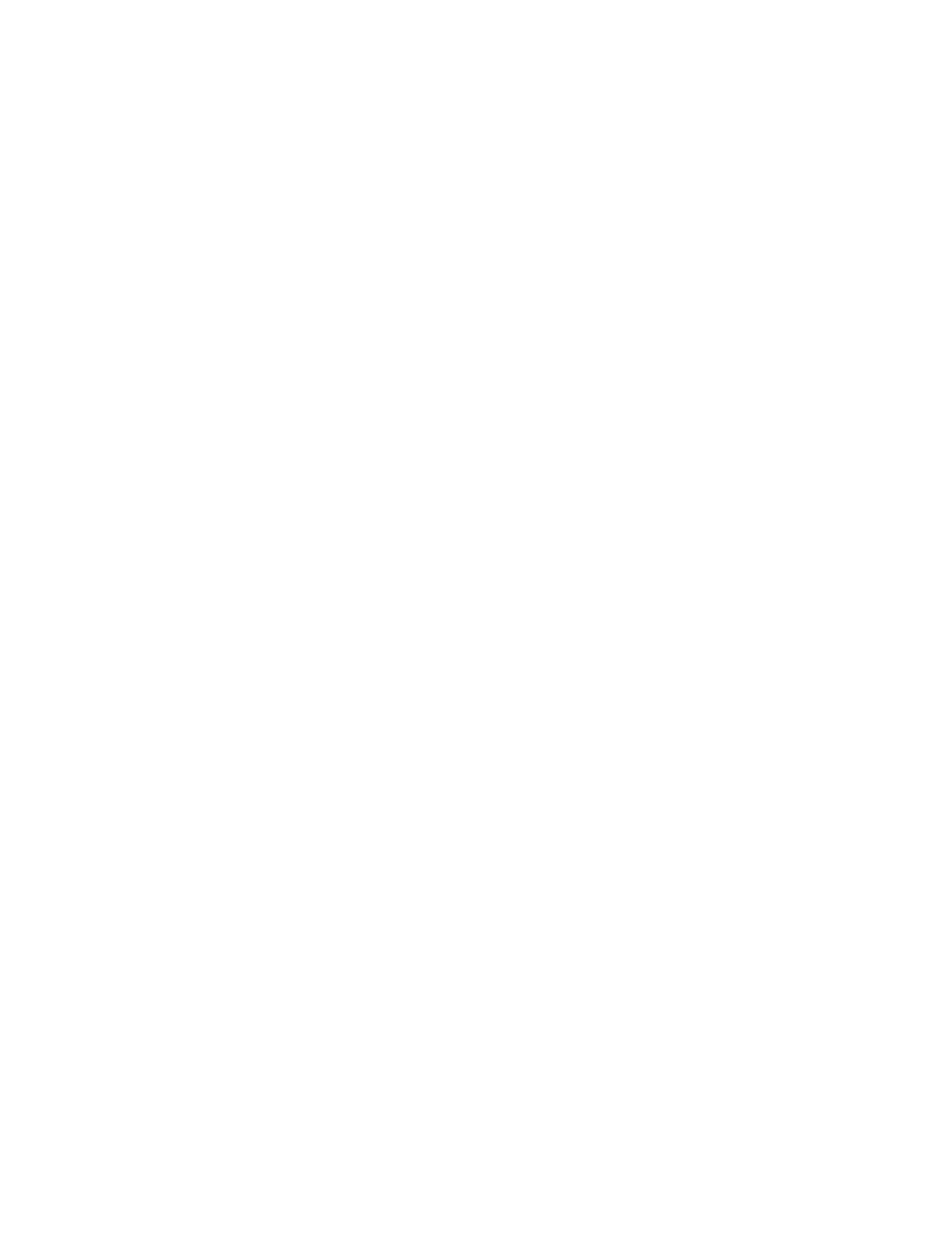
16-11
Chapter 16: Programming in C
SAmPLe PROGRAm
This program calculates a one-hour average temperature. The array named “numbers” sets
up a series of variables from 0 to 60 to hold a value for input 0 for each minute in an hour.
The 60 values are totaled, then averaged. The average can then viewed from the C & Ladder
Variables form, stored in the datalogger, or displayed on the Real-time screen.
By using an array, the program becomes substantially more concise. The program is first listed,
then a section by section explanation on how the program works is provided.
float numbers[60]; /* array: input 0 value for each minute */
int x;
/* index to the array */
float total;
/* total of the input 0 values */
float average;
/* total/60 */
int oldminute;
/* minute counter */
main()
{
if (oldminute != minutes)
{
oldminute=minutes;
numbers[minutes] = read_uaf(input,0,0);
total = 0;
for (x=0;x<60;x=x+1)
{
total = total + numbers[x];
}
average = total/60;
}
}
The following is a step by step explanation of the program.
1)
if (oldminute != minutes)
{
oldminute=minutes;
This checks the value of minutes to see if a minute has passed. If a minute has passed, the
value of oldminute is reset to the value of the new minute.
2)
numbers[minutes] = read_uaf(input,0,0);
This line sets the value of the variable “numbers” for a particular minute to the current value of
input 0. For example,
It is 3 minutes into the hour.
The value of input 0 is 72.
Therefore,
numbers[minutes] = read_uaf(input,0,0);
is equal to
numbers[3] = 72
3)
total = 0;
This line initializes the variable “total” to zero.