B&B Electronics VFG3000 - Manual User Manual
Page 165
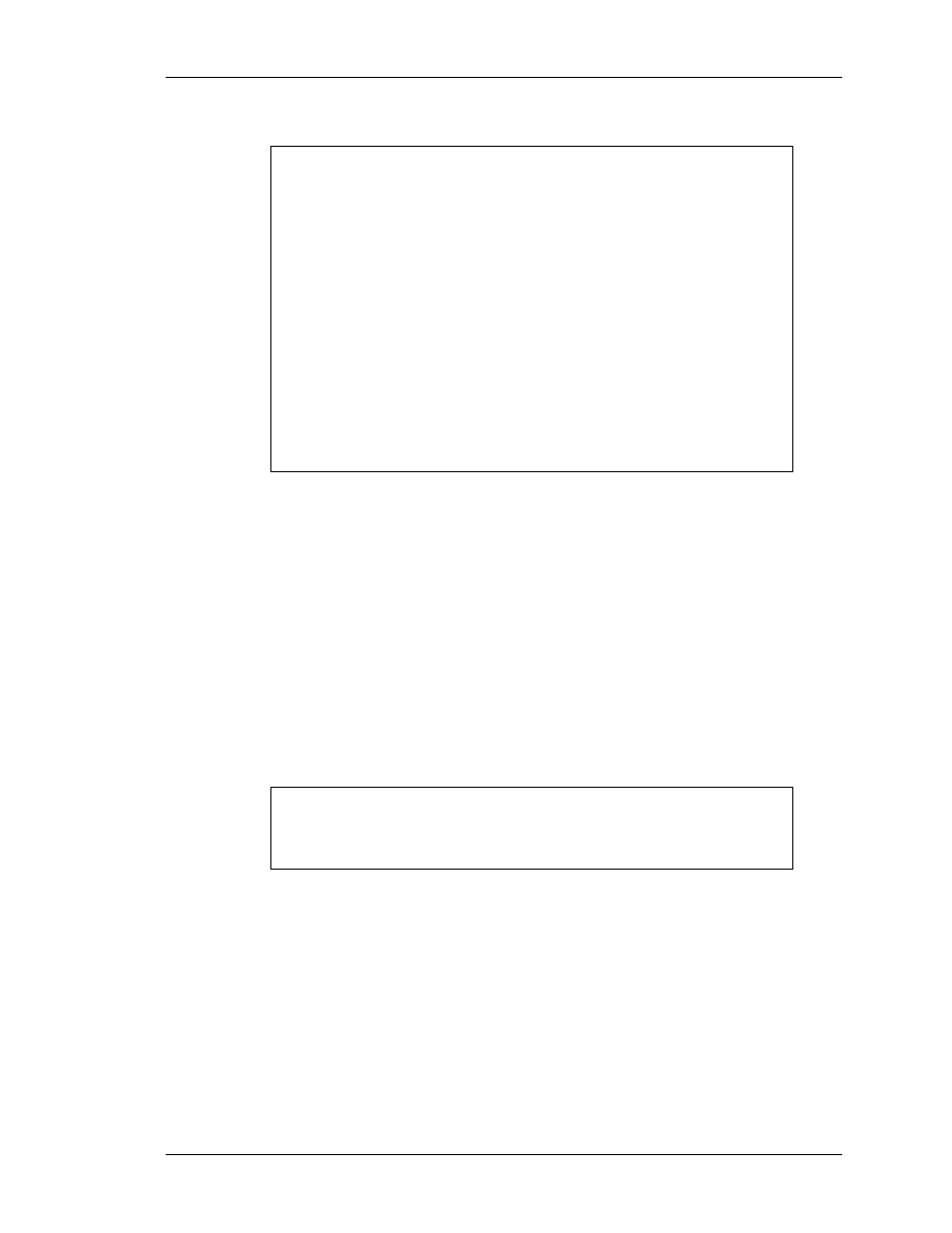
C
ONFIGURING
P
ROGRAMS
P
ROGRAMMING
T
IPS
R
EVISION
1
P
AGE
149
This example below will start a motor selected by the value in the
MotorIndex
tag...
switch( MotorIndex ) {
case 1:
MotorA := 1;
break;
case 2:
case 3:
MotorB := 1;
break;
case 4:
MotorC := 1;
break;
default:
MotorD := 1;
break;
}
A value of 1 will start motor A, a value of 2 or 3 will start motor B, and a value of 4 will start
motor C. Any value which is not explicitly listed will start motor D. Things to note about the
syntax are the use of curly-brackets around the
case
statements, the use of
break
to end each
conditional block, the use of two sequential
case
statements to match more than one value,
and the use of the optional
default
statement to indicate an action to perform if none of the
specified values is matched by the value in the controlling expression. (If this syntax looks
too intimidating, a series of
if
statements can be used instead to produce the same results, but
with marginally lower performance, and somewhat less readability.)
L
OCAL
V
ARIABLES
Some programs use variables to store intermediate results, or to control one of the various
loop constructs described below. Rather than defining a tag to hold these values, you can
declare what are known as local variables using the syntax shown below…
int a;
// Declare local integer ‘a’
float b;
// Declare local real ‘b’
cstring c;
// Declare local string ‘c’
Local variables may optionally be initialized when they are declared by following the variable
name with
:=
and the value to be assigned. Variables that are not initialized in this manner
are set to zero, or an empty string, as appropriate.
Note that local variables are truly local in both scope and lifetime. This means that they
cannot be referenced outside the program, and they do not retain their values between
function invocations. If a function is called recursively, each invocation has its own variables.
L
OOP
C
ONSTRUCTS
The three different loop constructs can be used to perform a given section of code while a
certain condition is true. The
while
loop tests its condition before the code is executed, while