Avago Technologies LSI53C1010 User Manual
Page 168
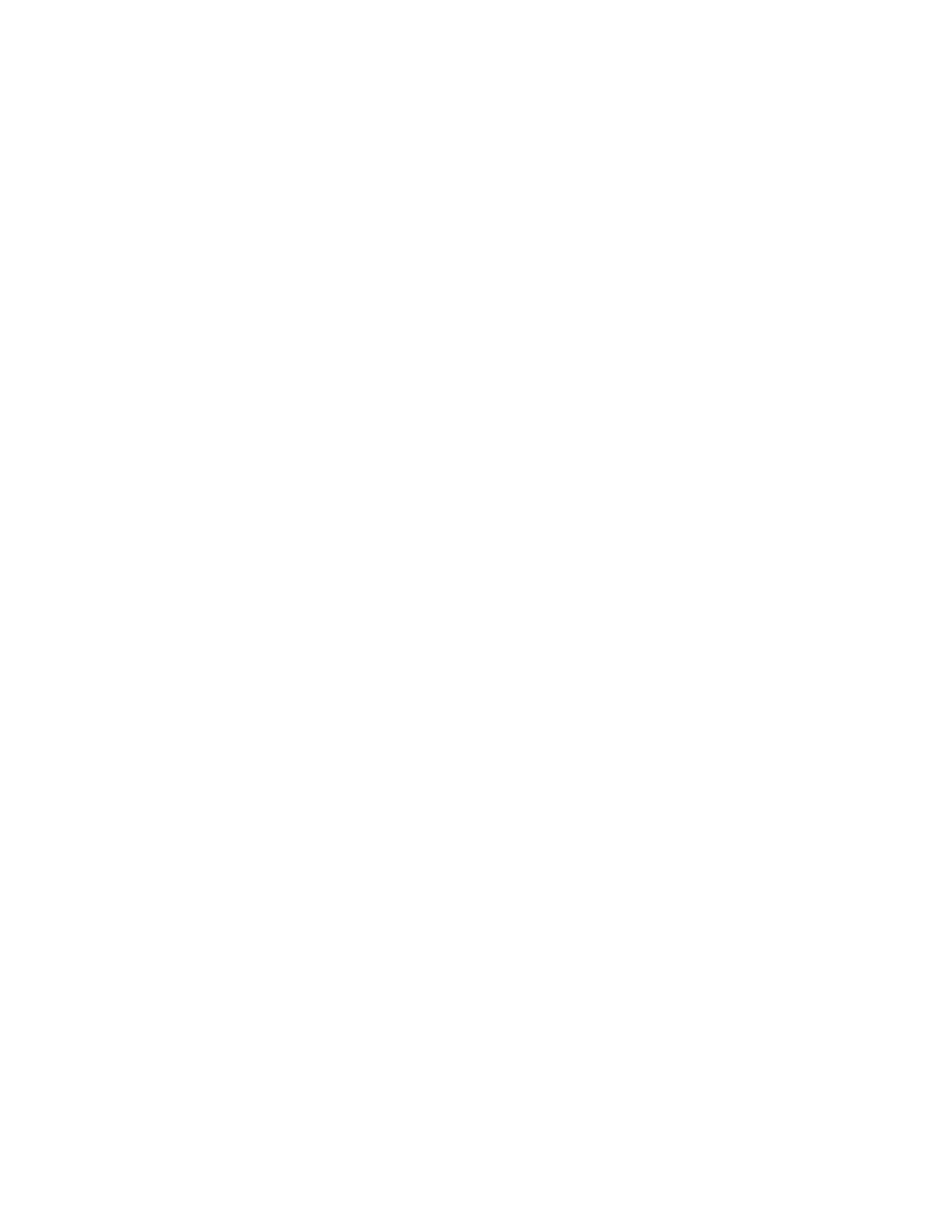
7-2
Integrating SCRIPTS Programs into “C” Language Drivers
}
/******************************************************
Function: IOWrite8
Purpose: To write a byte out to an IO port
Input: Value to be written and IO port address
Output: None
Assumptions: That the IO port actually exists
Restrictions: Although IO_Addr is defined as a
ULONG it must not exceed 16 bits
in length as this is the maximum IO
address the X86 architecture can produce
Other functions called: outportb to write to the io port
******************************************************/
void IOWrite8(ULONG IO_Addr, UBYTE value)
{
outportb((UINT) IO_Addr, value);
}
/******************************************************
Function: IORead32
Purpose: To read a dword (32 bits) from an io port
Input: IO address of dword to be read
Output: dword read from io port
Assumptions: That the IO port actually exists
Restrictions: Although IO_Addr is defined as a
ULONG it must not exceed 16 bits in
length as this is the maximum IO
address the X86 architecture can produce
Other functions called: none
******************************************************/
ULONG IORead32(ULONG IO_Addr)
{
ULONG result;
asm
{
.386
mov dx, [IO_Addr]
in eax, dx
mov [result], eax
}
return(result);
}
/******************************************************
Function: IOWrite32
Purpose: To write a dword (32 bits) out to an IO port
Input: Value to be written and IO port address
Output: None
Assumptions: That the IO port actually exists
Restrictions: Although IO_Addr is defined as a