Writing the fpm application shutdown() routine – Echelon i.LON SmartServer 2.0 User Manual
Page 99
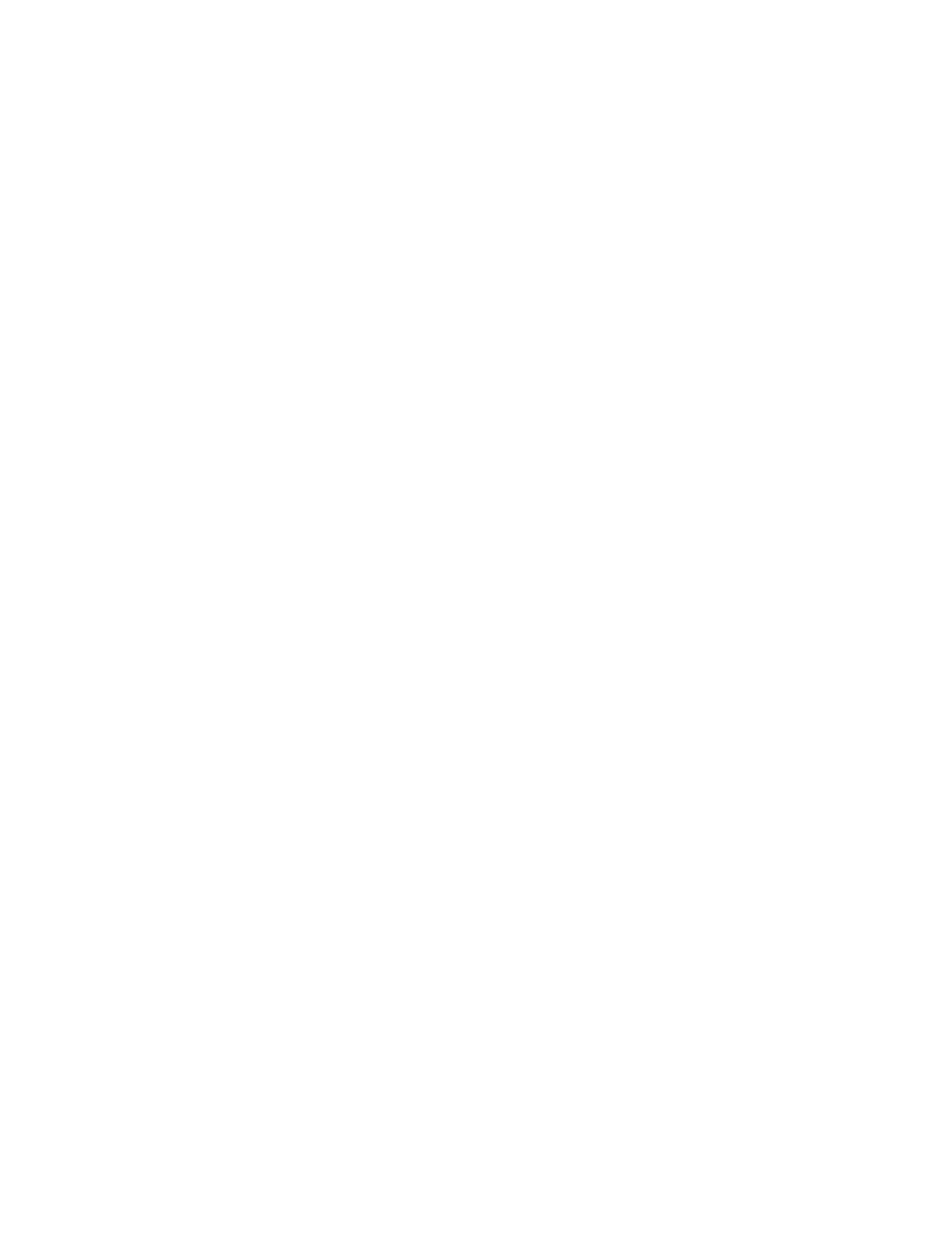
i.LON SmartServer 2.0 Programming Tools User’s Guide
85
The following code demonstrates how to create an OnTimer()routine that handles the expiration of
multiple timers started with the Start() method of the CFPM_Timer class. Observe that the
m_oTimer.Expired()
method is called first to determine which of the two timers started in the
Initialize()
routine expired.
void CUFPTHVACController::Initialize()
{
m_oTimer1.Start(FPM_TF_REPEAT, 2000);
m_oTimer2.Start(FPM_TF_ONETIME, 3000);
}
void CUFPTHVACController::OnTimer()
{
if
(m_oTimer1.Expired())
{
// do some task
}
if
(m_oTimer2.Expired())
{
// do some task
}
}
You must create custom OnMyTimer() routines for each user-defined timer you started with the
START_TIMER()
macro in the Initialize() routine. The following code demonstrates how to
create OnMyTimer()routines that handle the expiration of their respective timers started with the
START_TIMER()
macro.
void CUFPTHVACController::Initialize()
{
START_TIMER(m_oTimer3, FPM_TF_REPEAT, 2000, OnMyTimer3);
START_TIMER(m_oTimer4, FPM_TF_REPEAT, 2000, MyTimerHandler4);
}
void CUFPTHVACController::OnMyTimer3()
{
//do some task to handle expiration of the m_oTimer3 timer
}
void CUFPTHVACController::MyTimerHandler4()
{
//do some task to handle expiration of the m_oTimer4 timer
}
See the Programmer’s Reference in Appendix A for more information on starting timers and using
timer handler methods.
Writing the FPM Application Shutdown() Routine
The Shutdown() routine is executed when the FPM application is stopped or disabled. In the
Shutdown()
routine, you stop timers and perform any required cleanup. You can stop a timer using
use the Stop()and StopAllTimers()methods of the CFPM_Timer class.
• The StopTimer()method causes the system to stop the referenced timer.
• The StopAllTimers()method causes the system to stop all timers.
The following code demonstrates how you can stop timers in the Shutdown() routine: