Writing the fpm driver shutdown() routine – Echelon i.LON SmartServer 2.0 User Manual
Page 102
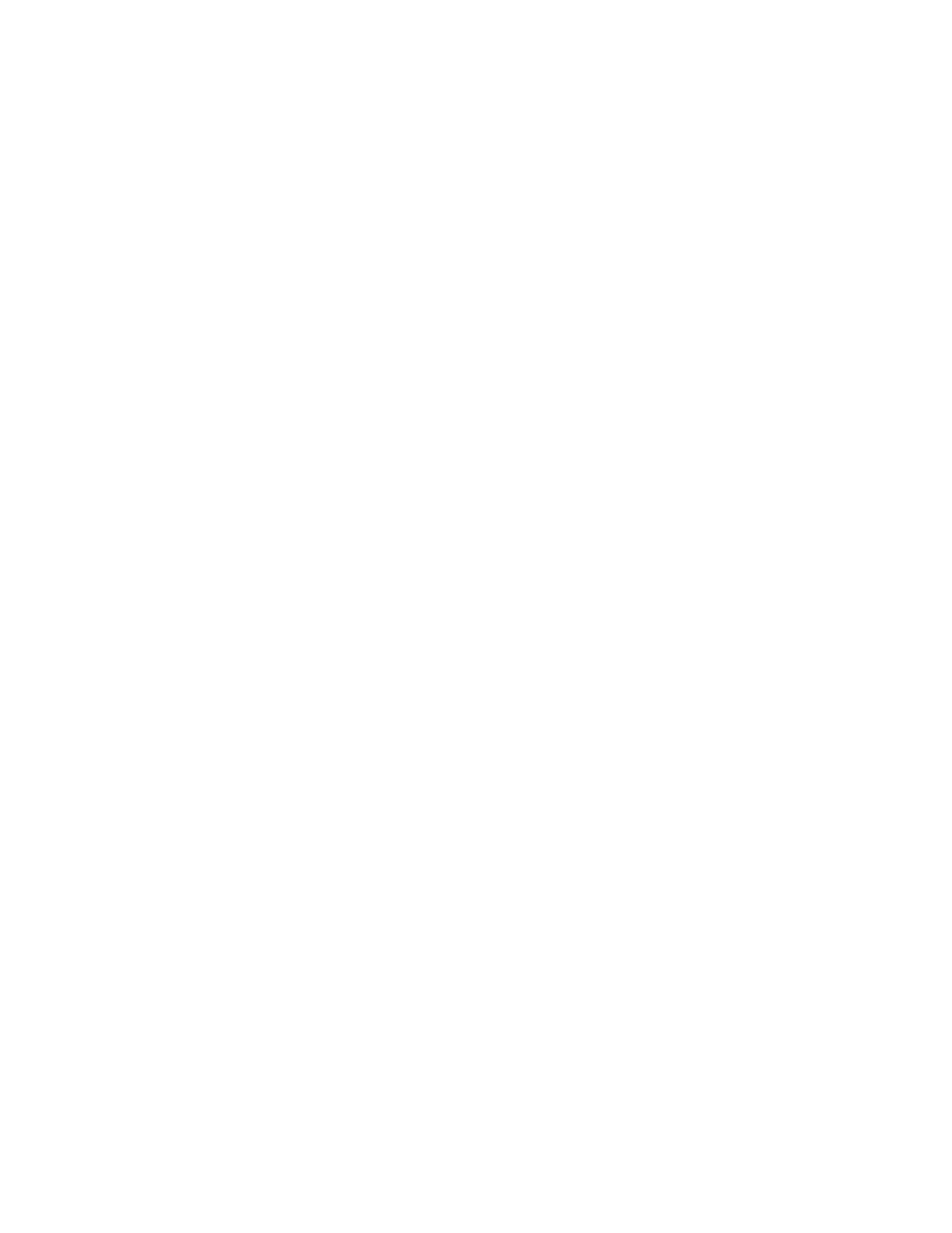
88
Creating Freely Programmable Modules
FPM and the devices connected to the RS-232 and RS-485 serial interfaces, read data from the RS-
232 or RS-485 interface, write updated values to data points, and read data point properties.
If you started more than one timer using the Start()method of the CFPM_Timer class, you must
first identify the timer that expired using the m_oTimer.Expired() method. In addition, you
must create custom OnMyTimer() routines for each user-defined timers you started with the
START_TIMER()
macro in the Initialize() routine.
You can initialize communication with the RS-232 and RS-485 serial interfaces using the methods
described in the previous section, Writing the FPM Driver Work() Routine. You can write data point
values and read data point properties using the methods described in Writing the FPM Application
Work() Routine. See rs232_read() in Appendix A for more information on using the ReadBytes()
function to read data from the RS-232 interface.
The following code demonstrates how to create an OnTimer()routine for an FPM driver.
//define buffer size in header file
#define MAX_RXBUFLEN 512
Byte rxBuf [MAX_RXBUFLEN];
…
void CRs232Driver::OnTimer()
{
if
(m_oDisplay_InputTimer.Expired())
{
memset(rxBuf,
0,
MAX_RXBUFLEN);
int nBytesRead = ReadBytes(RS232_fd, rxBuf, MAX_RX_BYTE_SIZE);
//check whether something has been read
if (nBytesRead >= 1)
{
printf ("Read %c from RS232\n", Line1);
//if something has been read, write it to display device
rs232_write(RS232_fd,
(Byte
*)rxBuf,
nBytesRead);
}
}
}
Writing the FPM Driver Shutdown() Routine
The Shutdown() routine is executed when the FPM driver is stopped or disabled. In the
Shutdown()
routine, you close the RS-232 or RS-485 interface, stop timers, and perform any
required cleanup.
To delete a timer, you can use the StopTimer() or StopAllTimers() methods. To close the
RS-232 interface, you use the rs232_close() method. To close the RS-485 interface, you use the
rs485_close()
method.
The following code demonstrates how you can close an RS-232 interface and stop timers in the
Shutdown()
routine:
void CUFPT_Display::Shutdown()
{
m_oDisplay_InputTimer.Stop();
rs232_close(RS232_fd);
}
See the Programmer’s Reference in Appendix A for more information on stopping timers and closing
the RS-232 and RS-485 interfaces.