Rs232_read() – Echelon i.LON SmartServer 2.0 User Manual
Page 241
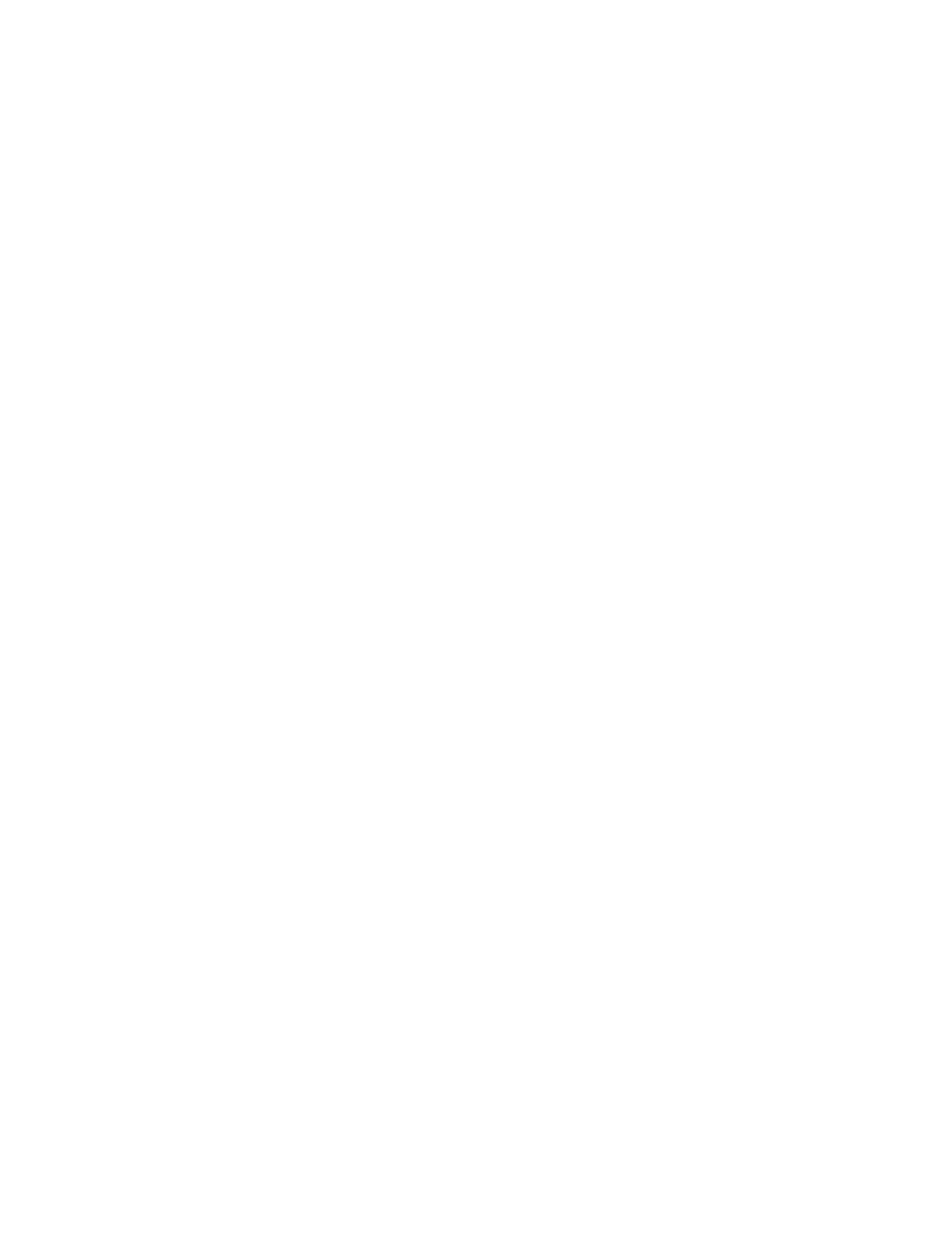
i.LON SmartServer 2.0 Programming Tools User’s Guide
227
parameter is set to IOCTL_SIO_HW_OPTS_SET.
This method returns the 0 upon success, and it returns -1 upon failure. A failure could occur if
another FPM is using the interface, or if the interface is not properly connected to the RS-232 port.
EXAMPLE
The following example demonstrates a rs232_ioctl()method that returns the current
configuration used by the RS-232 interface.
int options = rs232_ioctl(fd, IOCTL_SIO_HW_OPTS_GET, 0);
rs232_read()
You can use the rs232_read() method in the OnTimer() routine to read data from the RS-232
interface.
Note: Use the ReadBytes() function shown below to read data from the RS-232 interface. Do not
use the rs232_read() function directly to read data from the RS-232 receive buffer because it will
cause your FPM Driver to remain in an infinite loop until receive (Rx) data is received from the RS-
232 interface.
SYNTAX
int rs232_read(int fd, unsigned char * buf, int length);
The fd parameter specifies the file handle returned when the RS-232 interface was opened with
the rs232_open() method.
The buf parameter specifies a pointer to the memory area to where the data read from the RS-232
interface is to be stored. The memory area must have enough space to store the data or else the
SmartServer may fail as a result of a call to this method.
The length parameter specifies the maximum number of bytes that are to be read.
This method returns the number of bytes read upon success, and it returns -1 upon failure. A
failure could occur if another FPM is using the interface, or if the interface is not properly
connected to the RS-232 port.
EXAMPLE
rs232_read(fd, someBuffer, 1);
Note: If there is no data to be read from the RS-232 interface, the call to the rs232_read()
method will block and wait until new data arrives on the interface. To avoid blocking, you must
call both the select() and ioctl( fd, FIONREAD, ... ) methods before calling the
rs232_read()
method in order to set a timeout and therefore avoid blocking.
The following code demonstrates how to do this. The ReadBytes()and
BytesReadyForRead()
methods in this example are based on the ones included in the sample
RS-232 driver (Rs232Driver.cpp) provided in the
LonWorks\iLON\Development\eclipse\workspace.fpm folder:
//define buffer size in header file
#define MAX_RXBUFLEN 512
Byte rxBuf [MAX_RXBUFLEN];
…
void CRs232Driver::OnTimer()
{
if
(m_oDisplay_InputTimer.Expired())
{
memset(rxBuf,
0,
MAX_RXBUFLEN);