Initialize(), Fpm application example, Fpm driver example – Echelon i.LON SmartServer 2.0 User Manual
Page 219: Work()
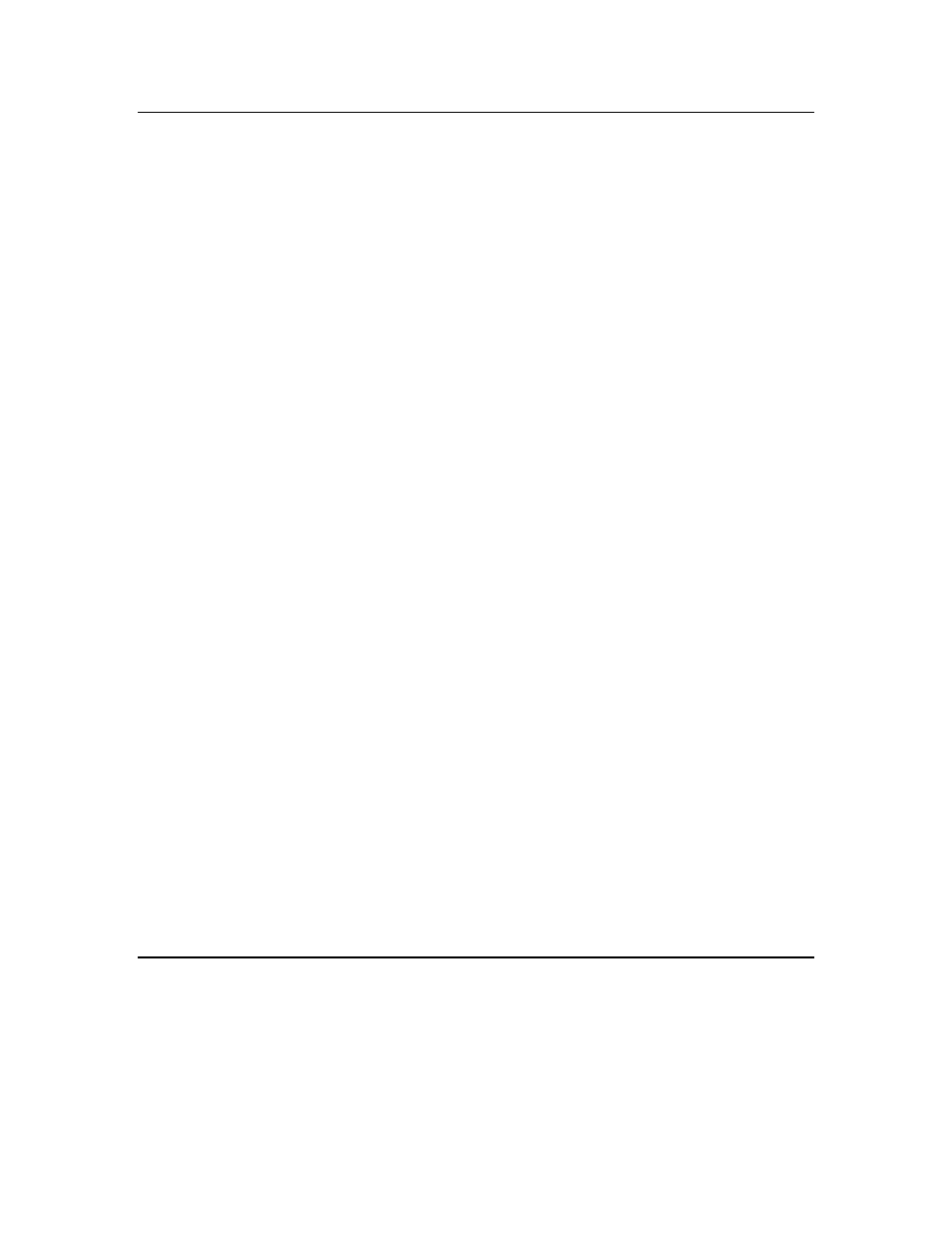
i.LON SmartServer 2.0 Programming Tools User’s Guide
205
Initialize()
The Initialize() routine in the .cpp file is called when your FPM application or driver starts or is
enabled. For an FPM application, you can use the Initialize() routine to write initial data point
values, and start timers. For an FPM driver, you can use the Initialize() routine to open RS-232
or RS-485 connections, start timers, and write data point properties.
• You can start timers using the Start()method of the CFPM_Timer class or the user-defined
START_TIMER()
macro. The Start()method calls back the OnTimer() routine, which
handles timers expiration events. The START_TIMER() macro calls back a custom timer
handler that you must create. See Timer Methods for more information about using the
Start()
method and the START_TIMER() macro.
• You can open the RS-232 and RS-485 interfaces on the SmartServer using the rs232_open()
and rs485_open() methods. For more information on these methods, see RS-232 Interface
Methods and RS-485 Interface Methods later in this chapter.
FPM Application Example
The following example demonstrates code you could use in the Initialize()routine of an FPM
Application. In this example, an initial value is written to a data point and two timers are started.
DECLARE(_0000000000000000_0_::SNVT_temp_f, nviSetPoint,
INPUT_DP)
CFPM_Timer m_oTimer1; //declared in header file
CFPM_Timer m_oTimer2; //declared in header file
void CUFPT_FPM_Application::Initialize()
{
nviSetPoint = 20.28;
m_oTimer1.Start(FPM_TF_REPEAT, 2000);
m_oTimer2.Start(FPM_TF_ONETIME, 0);
}
FPM Driver Example
The following example demonstrates code you could use in the Initialize()routine of an FPM
Driver. In this example, the FPM connects to an RS-232 interface and then starts one timer.
int _rs232_fd = -1;
CFPM_Timer m_oTimer3; //declared in header file
void CUFPT_FPM_Driver::Initialize()
{
_rs232_fd = rs232_open(9600);
m_oTimer3.Start(FPM_TF_REPEAT, 1200);
}
Work()
The Work() routine in the .cpp file is called when the value of a data point declared in an FPM
application or driver changes. The Work() routine establishes functionality between the data points
defined in the FPM to the data points on the SmartServer. When configuring the Work() routine, you
determine which data point was updated using the Changed() method. See Methods for more
information on using this method.