Measurement Computing WaveBook rev.3.0 User Manual
Page 192
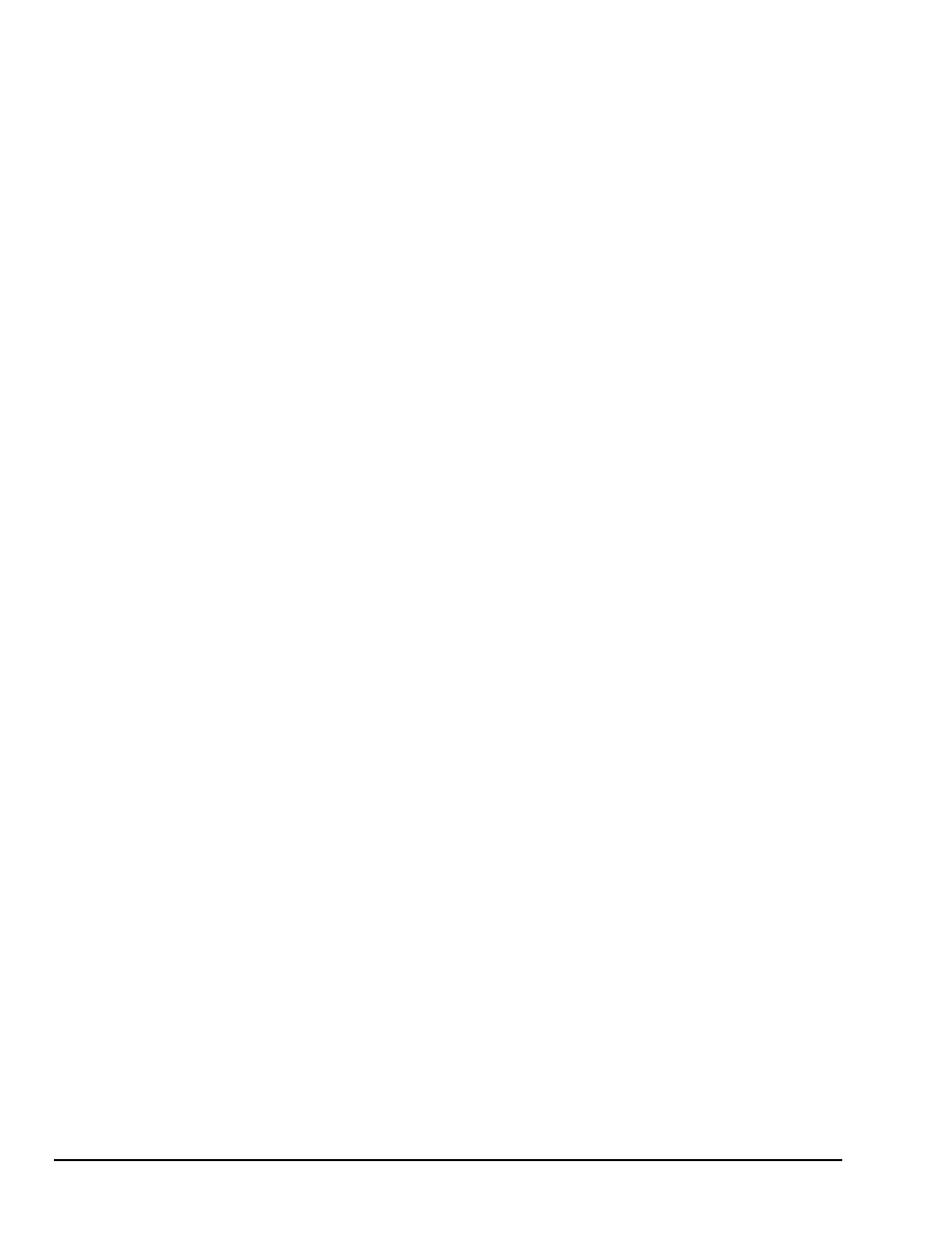
C-18 daqX API - Programming Models,
6-22-99
WaveBook User’s Manual
Set the scan configuration for channels 1 to 8 with a gain of ×1 in unipolar analog mode:
ret& = VBdaqAdcSetMux&(handle&, 1, channels&, DgainX1&,
DafUniPolar&+DafAnalog&)
Set the post-trigger scan frequency:
ret& = VBdaqAdcSetFreq&(handle&, freq!)
Set the trigger source to a software trigger command; the rest of the parameters have no effect on a software
trigger:
ret& = VBdaqAdcSetTrig&(handle&, DatsSoftware&, DatdRisingEdge&, 0,
HYSTERESIS%, 1)
Set the direct-to-disk filename with no pre-write, in append mode; also available is:
ret& = VBdaqAdcSetDiskFile&(handle&, "adcex8.bin", DaomAppendFile&, 0)
Start reading data in the background mode with cycle mode on and updateBlock:
ret& = VBdaqAdcTransferSetBuffer&(handle&, buf%(), BLOCK&, DatmCycleOn& +
DatmUpdateBlock&)
ret& = VBdaqAdcTransferStart&(handle&)
ret& = VBdaqAdcArm&(handle&)
ret& = VBdaqAdcSoftTrig&(handle&)
Monitor the progress of the transfer:
active& = -1
While active& <> 0
ret& = VBdaqAdcTransferGetStat&(handle&, active&, retCount&)
Wend
Print "Acquisition complete:"; retCount&; "scans acquired."
Close the device:
ret& = VBdaqClose&(handle&)
Now we convert the binary file to a text file. There is no simple way to do this, so it is necessary to open
the file and manipulate it by hand.
First, open the binary file:
Open "adcex8.bin" For Input As 1
Next, get a handle for the file; this is one of the windows API calls, CreateFile (it doesn’t actually
create anything, however).
fileHandle& = CreateFile(binFile, GENERIC_READ, &H1, "", CREATE_ALWAYS,
FILE_ATTRIBUTE_NORMAL, "")
Now open the text output file where the converted data will be written:
Open "adcex8.txt" For Output As 2
Next, actually convert the binary data to text:
Do
Convert BLOCK unpacked scans to packed bytes:
scanCount& = BLOCK&
sampleCount& = scanCount& * channels&
wordCount& = sampleCount& * 3 / 4
byteCount& = 2 * wordCount&
Read the packed bytes from the input file, and get the number of bytes actually read. The UBound()and
Lbound()
functions just return the upper and lower bounds of the buffer. Get #1 retrieves data from the
file and stores it in the buf() array.
Dim sz&
sz& = UBound(buf%) - LBound(buf%)
For i& = 0 To sz&
Get #1, i&, buf(i&)
Next i&
byteCount& = sz
Next, convert the data just read into the buffer from packed bytes to unpacked scans:
wordCount& = byteCount& / 2
sampleCount& = wordCount& * 4 / 3
scanCount& = sampleCount& / channels&
Unpack the packed data using the same buffer. This command can be called even if the WaveBook if not
online or connected.
ret& = VBdaqCvtRawDataFormat&(buf%(), DacaUnpack, BLOCK&, channels&,
scanCount&)