Initialization & error handling – Measurement Computing WaveBook rev.3.0 User Manual
Page 177
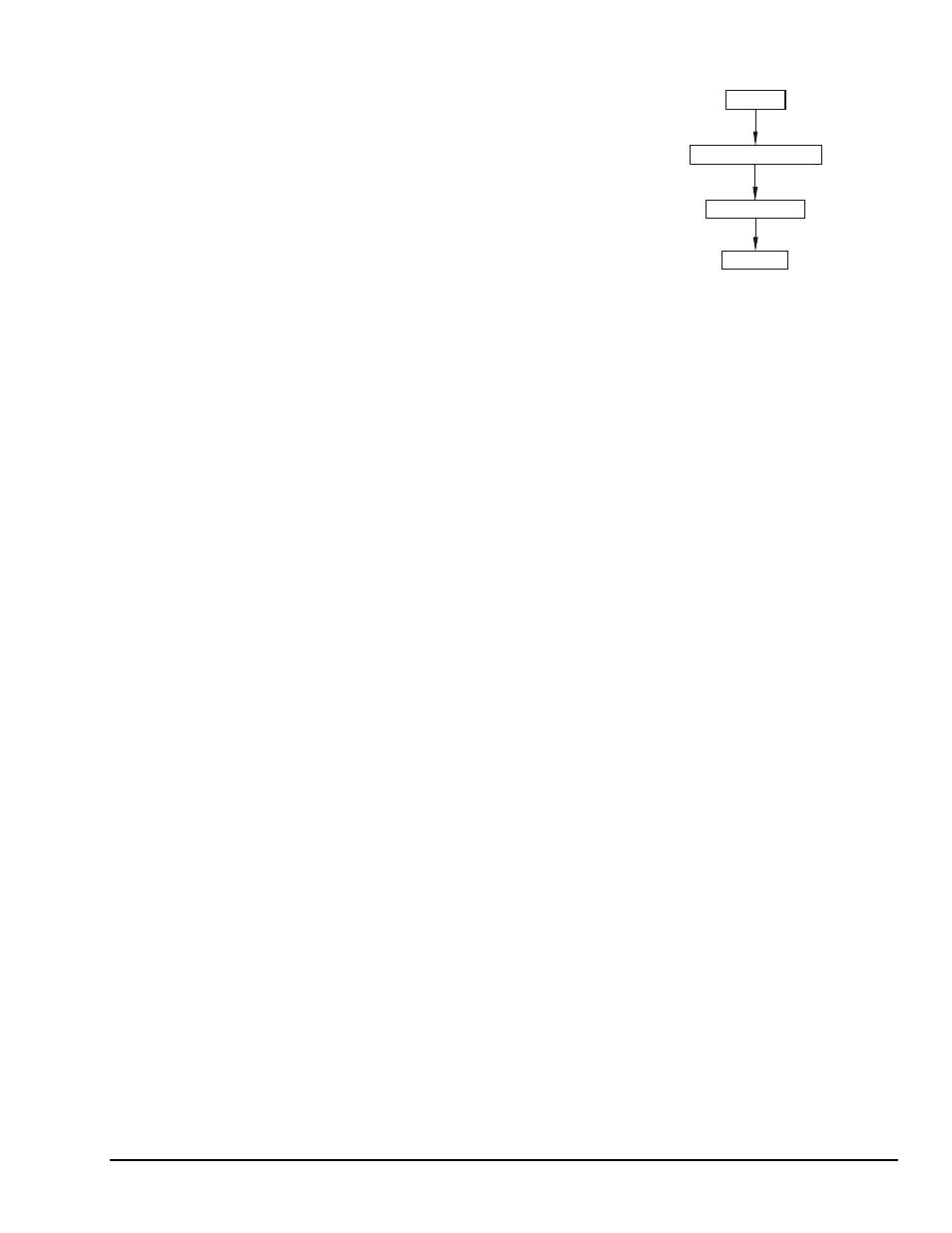
WaveBook User’s Manual,
6-22-99
daqX API - Programming Models C-3
Initialization & Error Handling
This section demonstrates how to initialize the Daq* and use various
methods of error handling. Most of the example programs use similar
coding as detailed here. Functions used include:
•
VBdaqOpen&(daqName$)
•
VBdaqSetErrorHandler&(errHandler&)
•
VBdaqClose&(handle&)
All Visual Basic programs should include the DaqX.bas file into their
project. The DaqX.bas file provides the necessary definitions and
function prototyping for the DaqX driver DLL.
handle& = VBdaqOpen&(“WaveBook0”)
ret& = VBdaqClose&(handle&)
Open a data
acquisition
session.
Setup error
handling
Close the
session.
daqClose
User Code
daqSetErrorHandler
daqOpen
The Daq* device is opened and initialized with daqOpen. daqOpen takes one parameter—the name of
the device to be opened. The device name can be accessed or changed via the Daq* Configuration utility
located in the operating system’s Control Panel. The daqOpen call, if successful, will return a handle to
the opened device (If the device could not be found or opened, daqOpen will return -1). The handle may
then be used by other functions to configure or use the device. When operations with the device are
complete, the device may then be closed with the daqClose function.
The DaqX library has a default error handler defined upon loading. However; to change or disable the error
handler, the daqSetErrorHandler function may be used to setup an error handler for the driver. In the
following example, the error handler is set to 0 (no handler defined) which disables error handling.
ret& = VBdaqSetErrorHandler&(0&)
If there is a Daq* error, the program will continue. The function’s return value (an error number or 0 if no
error) can help you debug a program.
If (VBdaqOpen&(“WaveBook0”) < 0) Then
“Cannot open DaqBook0”
Daq* functions return daqErrno&.
Print “daqErrno& : ”; HEX$(daqErrno&)
End If
The next statement defines an error handling routine that frees us from checking the return value of every
Daq* function call. Although not necessary, this sample program transfers program control to a user-
defined routine when an error is detected. Without a Daq* error handler, Visual Basic will receive and
handle the error, post it on the screen and terminate the program. Visual Basic provides an integer variable
(ERR) that contains the most recent error code. This variable can be used to detect the error source and
take the appropriate action. The function daqSetErrorHandler tells Visual Basic to assign ERR to a
specific value when a Daq*error is encountered. The following line tells Visual Basic to set ERR to 100
when a Daq*error is encountered. (Other languages work similarly; refer to specific language
documentation as needed.)
handle& = VBdaqOpen&(“WaveBook0”)
ret& = VBdaqSetErrorHandler&(handle&, 100)
On Error GoTo ErrorHandler
The On Error GoTo command in Visual Basic allows a user-defined error handler to be provided, rather
than the standard error handler that Visual Basic uses automatically. The program uses On Error GoTo
to transfer program control to the label ErrorHandler if an error is encountered.
Daq* errors will send the program into the error handling routine. This is the error handler. Program
control is sent here on error.
ErrorHandler:
errorString$ = "ERROR in ADC1"
errorString$ = errorString$ & Chr(10) & "BASIC Error :" + Str$(Err)
If Err = 100 Then errorString$ = errorString$ & Chr(10) & "DaqBook Error
: " + Hex$(daqErrno&)
MsgBox errorString$, , "Error!"
End Sub