Echelon i.LON SmartServer 2.0 User Manual
Page 368
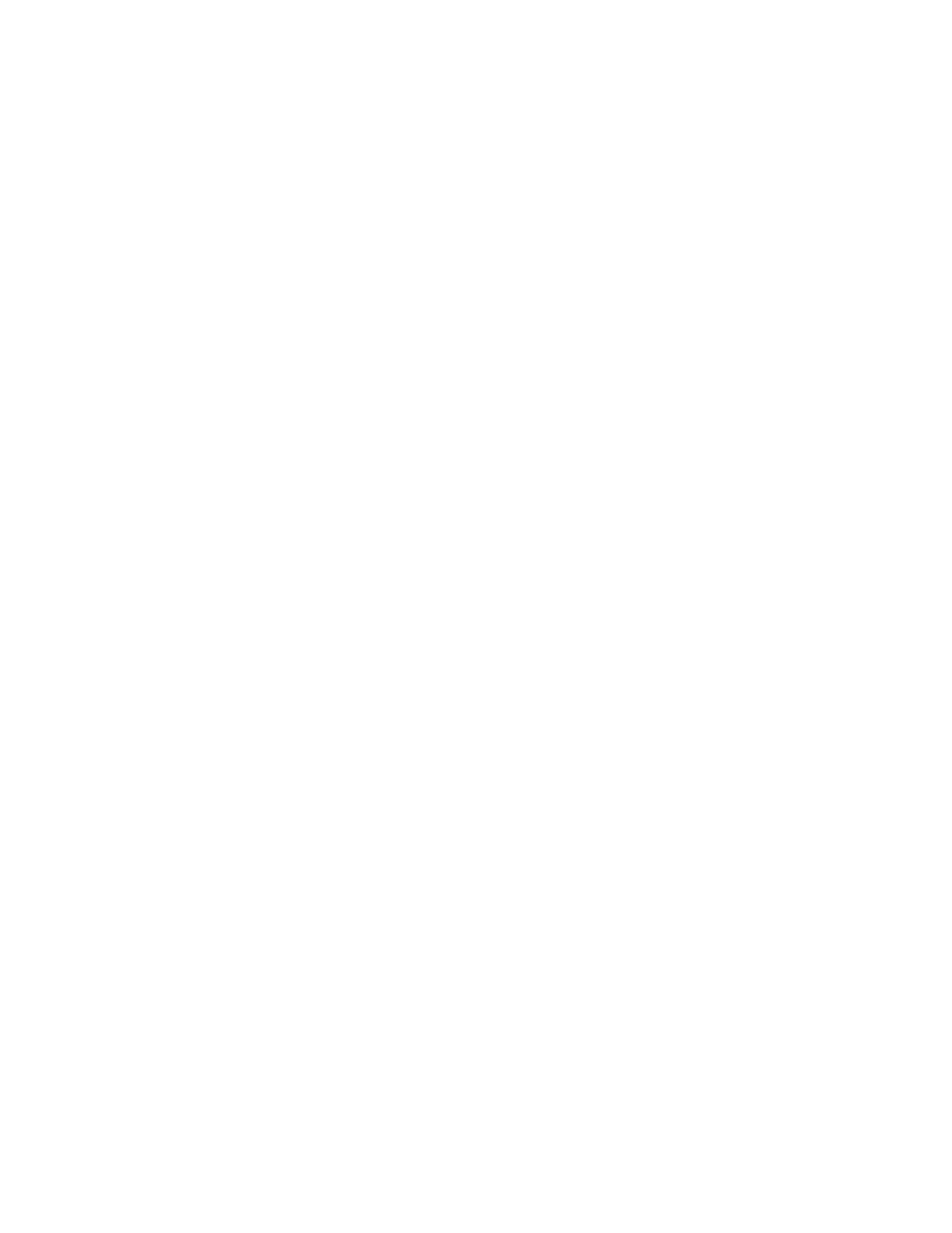
i.LON SmartServer 2.0 Programmer’s Reference
21-16
class
Program
{
//Function required for converting device Neuron IDs and program IDs to a byte[]
static
public
byte
[] HexStringToArray(
string
str)
{
int
nLen = str.Length / 2;
byte
[] arr =
new
byte
[nLen];
for
(
int
i = 0; i < nLen; i++)
{
string
strByte = str.Substring(i * 2, 2);
arr[i] =
Byte
.Parse(strByte, System.Globalization.
NumberStyles
.HexNumber);
}
return
arr;
}
static
void
Main(
string
[] args)
{
iLON_SoapCalls
.BindClientToSmartServer();
iLON_SmartServer.
iLON100portTypeClient
SmartServer =
iLON_SoapCalls
._iLON;
try
{
// -------------- CREATING LONWORKS DEVICES --------------
//Create a new LON_Device_Cfg Item
iLON_SmartServer.
LON_Device_Cfg
my_LON_Device1 =
new
iLON_SmartServer.
LON_Device_Cfg
();
iLON_SmartServer.
LON_Device_Cfg
my_LON_Device2 =
new
iLON_SmartServer.
LON_Device_Cfg
();
//Create an ItemCfgColl to store the LON Devices we just created
iLON_SmartServer.
Item_CfgColl
ItemCfgColl =
new
iLON_SmartServer.
Item_CfgColl
();
ItemCfgColl.Item =
new
iLON_SmartServer.
Item_Cfg
[2];
ItemCfgColl.Item[0] = my_LON_Device1;
ItemCfgColl.Item[1] = my_LON_Device2;
//=====CREATING AND INSTALLING LON DEVICE #1==================
// specify properties of new LON Device #1
my_LON_Device1.UCPTname =
"Net/LON/DIO-1"
;
my_LON_Device1.UCPTlocal = 0;
my_LON_Device1.UCPTuniqueId = HexStringToArray(
"00a145791500"
);
my_LON_Device1.UCPTprogramId = HexStringToArray(
"80000105288a0403"
);
my_LON_Device1.UCPTurlTemplate =
"/root/lonWorks/Import/Echelon/LonPoint/Version3/dio-10v3.XIF"
;
my_LON_Device1.UCPTcommissionStatus =
new
iLON_SmartServer.
E_LonString
();
my_LON_Device1.UCPTcommissionStatus.Value =
"COMMISSIONED"
;
my_LON_Device1.UCPTapplicationStatus =
new
iLON_SmartServer.
E_LonString
();
my_LON_Device1.UCPTapplicationStatus.Value =
"APP_RUNNING"
;
//create a command array to store device commands to be sent
my_LON_Device1.Command =
new
iLON_SmartServer.
LON_Device_CfgCommand
[3];
//commission device
my_LON_Device1.Command[0] =
new
ConsoleApplication_LON_Device.iLON_SmartServer.
LON_Device_CfgCommand
();
my_LON_Device1.Command[0].UCPTcommand =
new
iLON_SmartServer.
LON_Device_eCommand
();
my_LON_Device1.Command[0].UCPTcommand = iLON_SmartServer.
LON_Device_eCommand
.ChangeCommissionStatus;
my_LON_Device1.Command[0].UCPTstatus =
new
ConsoleApplication_LON_Device.iLON_SmartServer.
E_LonString
();
my_LON_Device1.Command[0].UCPTstatus.LonFormat =
"UCPTstatus"
;
my_LON_Device1.Command[0].UCPTstatus.Value =
"STATUS_REQUEST"
;
//run device application
my_LON_Device1.Command[1] =
new
iLON_SmartServer.
LON_Device_CfgCommand
();
my_LON_Device1.Command[1].UCPTcommand =
new
iLON_SmartServer.
LON_Device_eCommand
();
my_LON_Device1.Command[1].UCPTcommand = iLON_SmartServer.
LON_Device_eCommand
.ChangeApplicationStatus;
my_LON_Device1.Command[1].UCPTstatus =
new
ConsoleApplication_LON_Device.iLON_SmartServer.
E_LonString
();
my_LON_Device1.Command[1].UCPTstatus.LonFormat =
"UCPTstatus"
;