Echelon i.LON SmartServer 2.0 User Manual
Page 339
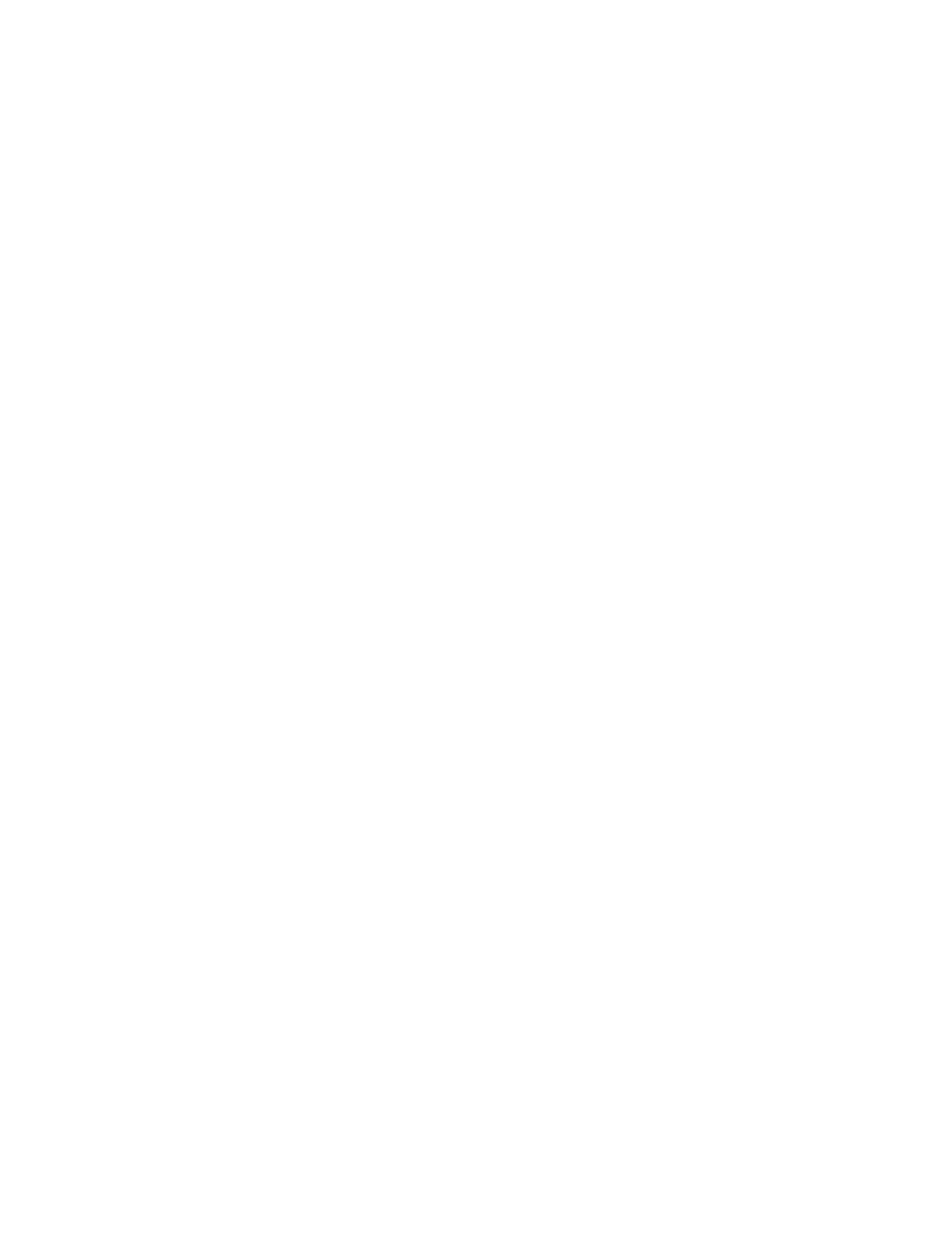
i.LON SmartServer 2.0 Programmer’s Reference
20-17
after you have completed section 20.2.1, Referencing and Inheriting from the WSDL Using .NET 2.0
Framework, and section 20.2.2, Instantiating the Web Service Client in Visual C# .NET 2.0.
using
System;
using
System.Collections.Generic;
using
System.Linq;
using
System.Text;
namespace
SmartServerConsoleExample
{
class
Program
{
// your SmartServer's IpAddress
public
static
string
_iLonEndpointIpAddress =
"<SmartServer IP Address>"
;
static
void
Main(
string
[] args)
{
iLON_SoapCalls
.BindClientToSmartServer(_iLonEndpointIpAddress);
iLON_SmartServer.
iLON100
SmartServer =
iLON_SoapCalls
._iLON;
// -------------- READ DATA POINT VALUE --------------
try
{
// instantiate the member object
iLON_SmartServer.
Item_Coll
itemColl =
new
iLON_SmartServer.
Item_Coll
();
itemColl.Item =
new
iLON_SmartServer.
Item
[1];
itemColl.Item[0] =
new
iLON_SmartServer.
Dp_Data
();
// set the DP name
itemColl.Item[0].UCPTname =
"Net/LON/iLON App/Digital Output 1/nviClaValue_1"
;
// set maxAge to get the updated DP value in case it has been cached for more than 10
// seconds on the Data Server (see section 4.3.4.1 for more information)
((iLON_SmartServer.
Dp_Data
)(itemColl.Item[0])).UCPTmaxAge = 10;
((iLON_SmartServer.
Dp_Data
)(itemColl.Item[0])).UCPTmaxAgeSpecified =
true
;
//call the Read Function
iLON_SmartServer.
Item_DataColl
dataColl = SmartServer.Read(itemColl);
if
(dataColl.Item ==
null
)
{
// sanity check. this should not happen
Console
.Out.WriteLine(
"No items were returned"
);
}
else
if
(dataColl.Item[0].fault !=
null
)
{
// error
Console
.Out.WriteLine(
"An error occurred. Fault code = "
+
dataColl.Item[0].fault.faultcode +
". Fault text = %s."
+
dataColl.Item[0].fault.faultstring);
}
else
{
// success
Console
.Out.WriteLine(
"Read is successful"
);
Console
.Out.WriteLine(((iLON_SmartServer.
Dp_Data
)dataColl.Item[0]).UCPTname +
" = "
+
((iLON_SmartServer.
Dp_Data
)dataColl.Item[0]).UCPTvalue[0].Value +
"\n"
);
}
// -------------- WRITE DATA POINT VALUE --------------
// reset the DP priority (see section 4.3.6 for more information)
iLON_SmartServer.
Item_Coll
itemCollInvoke =
new
iLON_SmartServer.
Item_Coll
();
itemCollInvoke.Item =
new
iLON_SmartServer.
Item
[1];
itemCollInvoke.Item[0] =
new
iLON_SmartServer.
Dp_ResetPrio_Invoke
();
((iLON_SmartServer.
Dp_ResetPrio_Invoke
)(itemCollInvoke.Item[0])).UCPTname =