5 string expressions – Campbell Scientific CR9000X Measurement and Control System User Manual
Page 166
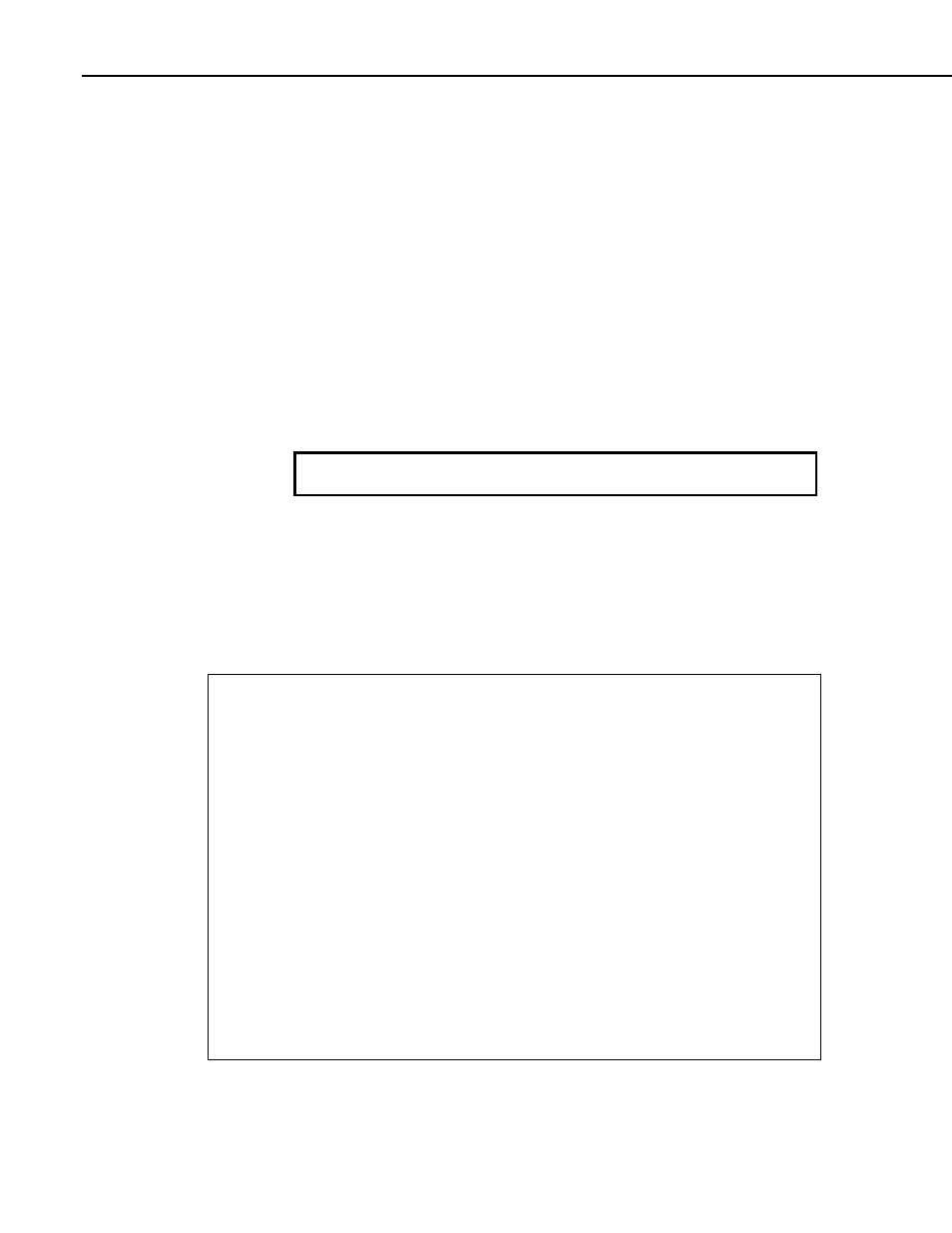
Section 4. CRBasic – Native Language Programming
The following commands and logical operators are used to construct logical
expressions.
IF
AND
OR
NOT
XOR
IIF
Conditional tests can require the CR9000X to evaluate an expression and take
one path if the expression is true and another if the expression is false. For
example:
If X>=5 then Y=0
will set the variable Y to 0 if X is greater than or equal to 5. The CR9000X can
also evaluate expressions linked with multiple ands or ors:
If X>=5 and Z=2 then Y=0
will only set Y=0 if both X>=5 and Z=2 are true.
If X>=5 or Z=2 then Y=0
will set Y=0 if either X>=5 or Z=2 is true.
See Section 8 Processing and Math Functions for more information on If,
Not, And, Or, Xor, & IIF.
4.2.11.5 String Expressions
CRBASIC allows the addition or concatenation of string variables to variables
of all types using & and + operators. To ensure consistent results, use “&”
when concatenating strings. Use “+” when concatenating strings to other
variable types. Example 4.2.11-5 demonstrates CRBASIC code for
concatenating strings and integers.
EXAMPLE 4.2.11-5 CRBASIC Code: String and Variable Concatenation
'Declare Variables
Dim Wrd(8) As String * 10
Public Phrase(2) As String * 80
Public PhraseNum(2) As Long
'Declare Data Table
DataTable (Test,1,-1)
DataInterval (0,15,Sec,10)
Sample (2,Phrase,String)
'Write phrases to data table "Test"
EndTable
BeginProg
'
Program
Scan (1,Sec,0,0)
'Assign strings to String variables
Wrd(1) = " " : Wrd(2) = "Good" : Wrd(3) = "morning" : Wrd(4) = "Don't"
Wrd(5) = "do" : Wrd(6) = "that" : Wrd(7) = "," : Wrd(8) = "Dave"
'Assign integers to Long variables
PhraseNum(1) = 1:PhraseNum(2) = 2
'Concatenate string "1 Good morning, Dave
"
Phrase(1) = PhraseNum(1)+Wrd(1)&Wrd(2)&Wrd(1)&Wrd(3)&Wrd(7)&Wrd(1)&Wrd(8)
'Concatenate string "2 Don't do that, Dave"
Phrase(2) = PhraseNum(2)+Wrd(1)&Wrd(4)&Wrd(1)&Wrd(5)&Wrd(1)&Wrd(6)&Wrd(7)&Wrd(1)&Wrd(8)
CallTable Test
NextScan
EndProg
4-38