1 floating point arithmetic, 2 mathematical operations – Campbell Scientific CR9000X Measurement and Control System User Manual
Page 163
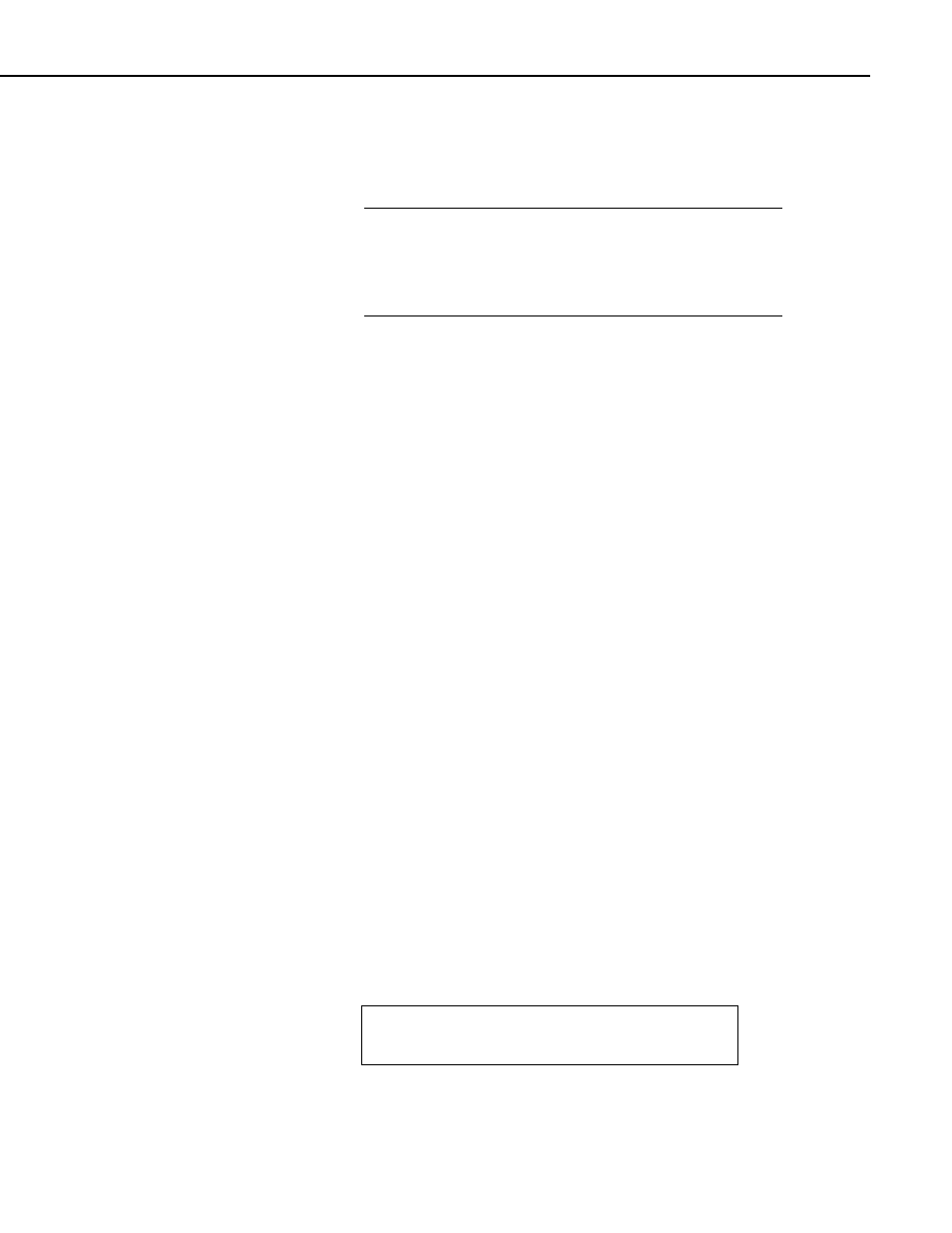
Section 4. CRBasic – Native Language Programming
4.2.11.1 Floating Point Arithmetic
Variables and calculations are performed internally in single precision IEEE4
byte floating point with some operations calculated in double precision.
Single precision float has 24 bits of mantissa. Double precision
has a 32-bit extension of the mantissa, resulting in 56 bits of
precision. Instructions that use double precision are AddPrecise,
Average, AvgRun, AvgSpa, CovSpa, MovePrecise, RMSSpa,
StdDev, StdDevSpa, and Totalize.
NOTE
Floating point arithmetic is common in many electronic computational systems,
but it has pitfalls high-level programmers should be aware of. Several sources
discuss floating point arithmetic thoroughly. One readily available source is the
topic “Floating Point” at Wikipedia.org. In summary, CR9000X programmers
should consider at least the following:
• Floating point numbers do not perfectly mimic real numbers.
• Floating point arithmetic does not perfectly mimic true arithmetic.
• Avoid use of equality in conditional statements. Use >= and <= instead.
For example, use “If X => Y, then do” rather than using, “If X = Y, then
do”.
• When programming extended cyclical summation of non-integers, use the
AddPrecise() instruction. Otherwise, as the size of the sum increases,
fractional addends will have ever decreasing effect on the magnitude of the
sum, because normal floating point numbers are limited to about 7 digits of
resolution.
4.2.11.2 Mathematical Operations
Mathematical operations are written out much as they are algebraically. For
example, to convert Celsius temperature to Fahrenheit, the syntax is:
TempF = TempC * 1.8 + 32
With the CR9000X there may be 5 or 50 temperature (or other) measurements.
Rather than have 50 different names, a variable array with one name and 50
elements may be used. A thermocouple temperature might be declared simply
with the Public instruction:
Public TCTemp(50).
With an array of 50 elements the names of the individual temperatures are
TCTemp(1), TCTemp(2), TCTemp(3), ... TCTemp(50). The array notation
allows compact code to perform operations on all the variables. Example
4.2.11-1 shows example code to convert twenty temperatures in a variable array
from C to F:
EXAMPLE 4.2.11-1. CRBasic Code: Use of variable arrays .
For I=1 to 50
TCTemp(I)=TCTemp(I)*1.8+32
Next I
4-35