Measurement Computing Data Acquisition Systems rev.10.4 User Manual
Page 34
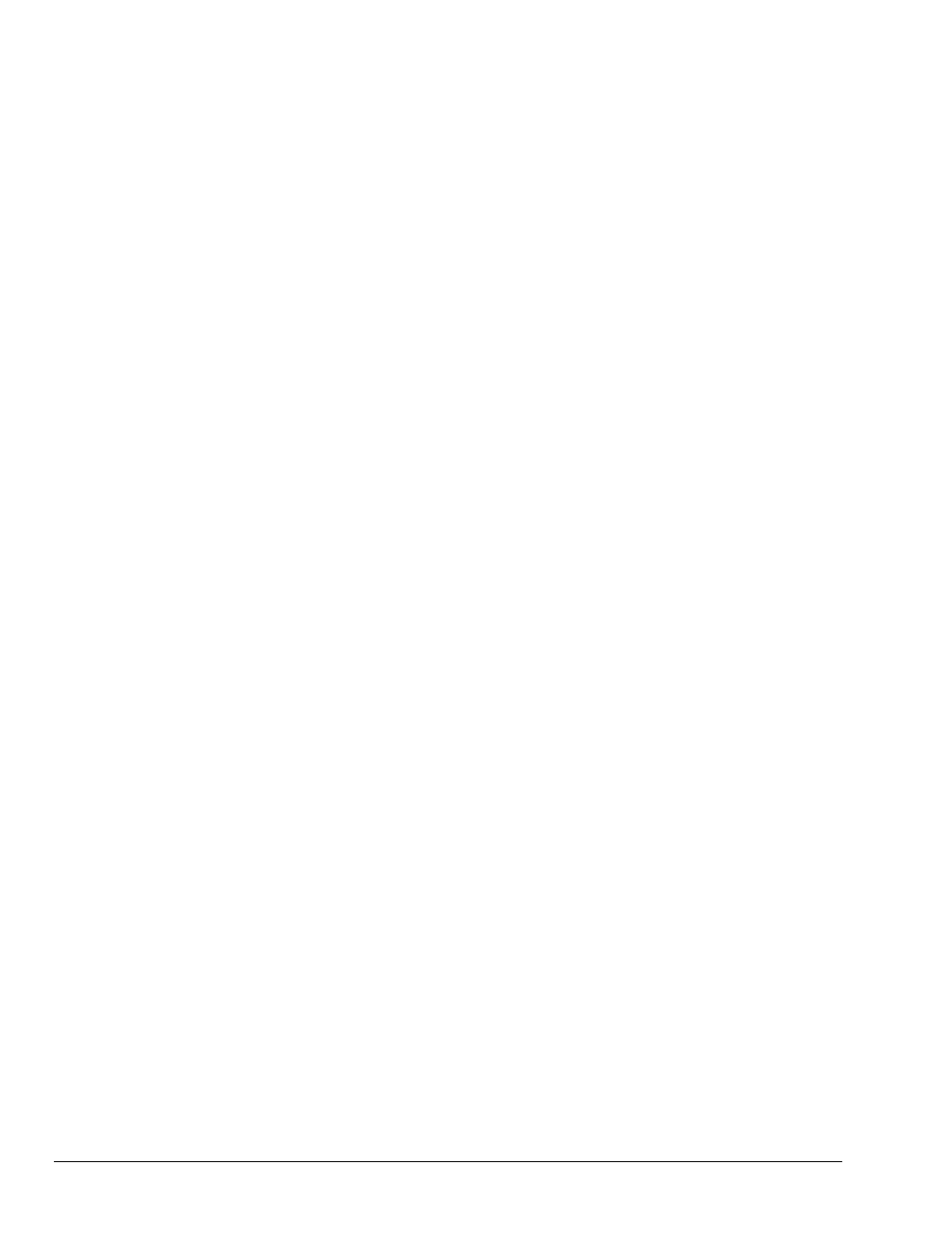
2-24 API Programming, General Models
988594
Programmer’s
Manual
Public Sub ZeroComp()
' Performs zero compensation on ADCs readings
Const ScanLength& = 6 'Total channels per scan
Const ScanCount& = 5 'Number of scans to acquire
Dim chan&(ScanLength), gain&(ScanLength)
Dim flag&(ScanLength), buf%(ScanLength * ScanCount)
Dim ret&, daqHandle&
daqHandle& = VBdaqOpen&("daqbook0")
' Channel zero must be shorted to ground
' Use DafClearLSNibble flag to clear 4 least significant
' bits when using 12-bit A/D converters
chan&(0) = 0: gain&(0) = DgainX1&: flag&(0) = DafBipolar& +
DafClearLSNibble&
chan&(1) = 0: gain&(1) = DgainX2&: flag&(1) = DafBipolar& +
DafClearLSNibble&
chan&(2) = 1: gain&(2) = DgainX1&: flag&(2) = DafBipolar& +
DafClearLSNibble&
chan&(3) = 2: gain&(3) = DgainX2&: flag&(3) = DafBipolar& +
DafClearLSNibble&
chan&(4) = 3: gain&(4) = DgainX2&: flag&(4) = DafBipolar& +
DafClearLSNibble&
chan&(5) = 4: gain&(5) = DgainX2&: flag&(5) = DafBipolar& +
DafClearLSNibble&
ret& = VBdaqAdcSetScan&(daqHandle&, chan&(), gain&(), flag&(), ScanLength)
ret& = VBdaqAdcSetTrig&(daqHandle&, DatsImmediate&, 1, 0, 0, 0)
ret& = VBdaqAdcSetAcq&(daqHandle&, DaamNShot&, 0, ScanCount)
ret& = VBdaqAdcSetClockSource&(daqHandle&, DacsAdcClock&)
ret& = VBdaqAdcSetFreq&(daqHandle&, 100!)ret& =
VBdaqAdcTransferSetBuffer&(daqHandle&, buf%(), ScanCount, DatmCycleOff& +
DatmUpdateSingle&)
ret& = VBdaqAdcTransferStart&(daqHandle&)
ret& = VBdaqAdcArm&(daqHandle&)
ret& = VBdaqWaitForEvent&(daqHandle&, DteAdcDone&)
' Print the first scan of unconverted data
Print "Channel zero shorted to ground"
Print "Channel 0 at X1 gain: "; IntToUint(buf%(0))
Print "Channel 0 at X2 gain: "; IntToUint(buf%(1))
Print
Print "Before zero compensation"
Print "Channel 1 at X1 gain: "; IntToUint(buf%(2))
Print "Channel 2 at X2 gain: "; IntToUint(buf%(3))
Print "Channel 3 at X2 gain: "; IntToUint(buf%(4))
Print "Channel 4 at X2 gain: "; IntToUint(buf%(5))
Print
' Perform zero compensation on readings sampled at x1 gain.
' 1 reading at position 2. Zero reading at position 0.
ret& = VBdaqZeroSetupConvert&(ScanLength, 0, 2, 1, buf%(), ScanCount)
' Perform zero compensation on readings sampled at x2 gain.
' 3 readings at position 3. Zero reading at position 1.
ret& = VBdaqZeroSetupConvert&(ScanLength, 1, 3, 3, buf%(), ScanCount)
' Print the first scan of converted data
Print "After zero compensation"
Print "Channel 1 at X1 gain: "; IntToUint(buf%(2))
Print "Channel 2 at X2 gain: "; IntToUint(buf%(3))
Print "Channel 3 at X2 gain: "; IntToUint(buf%(4))
Print "Channel 4 at X2 gain: "; IntToUint(buf%(5))
Print
' Close the device
ret& = VBdaqClose&(daqHandle&)
End Sub
Function IntToUint(intval As Integer) As Long
' Converts 16-bit signed integer to unsigned long integer
If 0 <= intval Then