Global variables – Contemporary Control Systems BASview User Manual
Page 67
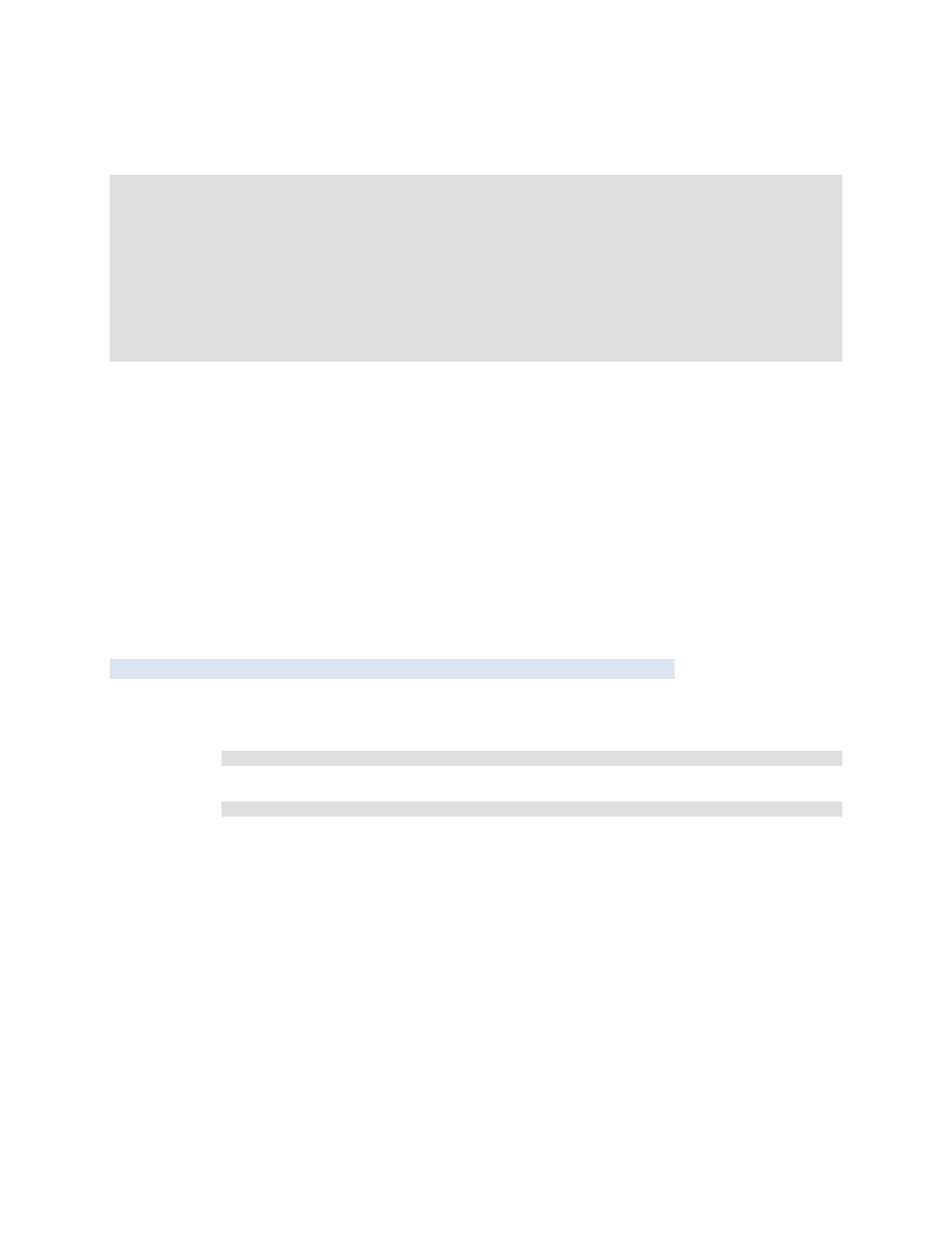
TD110500-0MC
67
The solution is to use the built in timer. Each program has its own timer that is continuously incremented
in the background. It always returns a value equal to the number of seconds the program has run (since it
was saved or since reboot)
— or since it was last cleared. Here is a safer example of the above program:
if timer() > 60*5:
#Have 5 minutes passed?
if getValue(@mySwitch)>0:
#Is the switch being pressed?
isOverridden = getVariable("isOV")
#get stored value
if isOverridden:
#if it is already overridden
clearTimer()
#reset timer to 0
release(@myLights)
#release myLights
releaseSchedule(@mySched)
#release schedule
setVariable("isOV",0)
#set isOV to 0
else:
#if it is not overridden
clearTimer()
#reset timer to 0
setValue(@myLights,1,3600)
#override myLights
overrideSchedule(@mySched,1,3600)
#override schedule
setVariable("isOV",1)
#set isOV to 1
In the above example, the timer is checked to see if it has been more than 5 minutes since the override
state changed. If so, the program continues like in the previous example. If 5 minutes have not passed,
the program exits and the switch is never examined. The key to making this example work is to clear the
timer every time the override state changes so it will stay that way for at least 5 minutes and not short-
cycle the points.
Unfortunately, the program is still not perfect. If the user hits the override switch to override for 1 hour,
then 4 minutes later decides he is done and wants to leave, pressing the switch has no effect and the
override will remain active for the full hour. This is an example of the thought necessary for writing a solid
program. Every possibility must be taken in to account
— or the results may not be what is expected.
There really is no "best" solution for hitting the switch after 4 minutes. The timer check could be lowered
to 2 minutes, but it will still ignore it if the user presses it after 1 minute 50 seconds. This is not likely to
happen, and worst case the lights stay on 58 minutes longer than required. In this particular example it is
not a major problem if the switch press is missed so you may decide that this program is sufficient.
Global Variables
Global variables can be used to share values between programs. They work exactly like saved variables
except that only 100 global variables are allowed and they are shared amongst all programs. If a
program sets a global variable called "bias" to 50, all other programs can read and
modifty
that value.
setGlobal("bias", 12.5)
#store the value of bias globally
A different program can then use:
myBias=getGlobal("bias")
#get the value and store in myBias
Calling getGlobal with a variable name that has not been defined will always return zero.
If a global variable is no longer needed, use removeGlobal("myVariable") to remove it from the list of
global variables.