Programming reference, Overview, Basic rules – Contemporary Control Systems BASview User Manual
Page 64
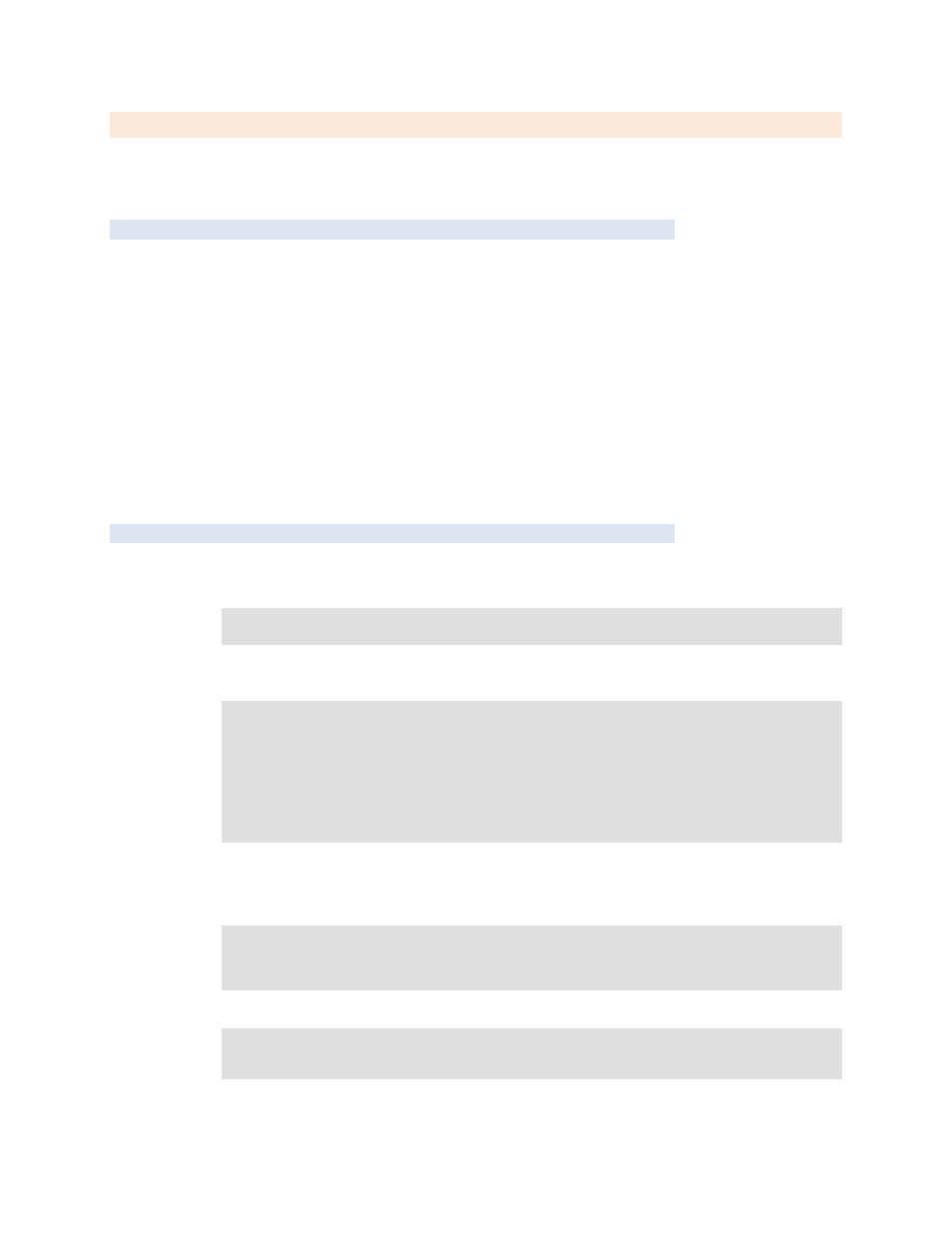
TD110500-0MC
64
Programming Reference
The following is a reference guide on writing Python programs for the BASview. For more on setup and
configuration of programming nodes
— and an explanation of "reference names" see the
Overview
Programs for the BASview are written using a modified version of
Python is a very easy to learn language, but can scale up to more complicated tasks when necessary.
The BASview itself makes extensive use of Python internally.
The following reference is designed to allow a new user to write simple programs. It is recommended that
users new to programming also view at least one tutorial on the Python language. Several of them may
be found on the Internet vi
just the basics of structure and indentation,
if/elif/
else blocks and using simple functions.
The Python language used in the BASview has the following differences from normal Python:
It is based on version 2.4 of Python.
The "import" keyword has been disabled for security reasons.
A double underscore "__" is not allowed anywhere in a program.
Basic Rules
The following are some basic rules for a Python program.
Any text on a line after a # symbol is considered a comment and is ignored
Example:
bias = bias + 0.5
#This is a comment and ignored.
Python uses indentation (spaces or tabs) to define structure, rather than brackets or begin/end
keywords.
Example:
if setpoint > 65:
#note the required colon for if, elif and else
bias = 0.5
#part of the "if" condition
elif setpoint > 75:
#elif means "else if"
bias = 0.7
#part of the "elif" condition
else:
bias = 0.2
#do this if the other conditions are false
deadband = 2
#still part of the "else" condition
output = output + bias
#not part of the if/elif/else block
Any number of spaces may be used for indentation, but it must be consistent for each block. It is
recommended that a number be chosen and used in all programs (2 or 4 are popular choices).
Local variables do not need to be declared and can be of any data type (which can be changed).
Example:
myVariable = 50
#myVariable is created as an integer
myVariable = 6.5
#then changed to a floating point number
myVariable = "test"
#then to a string
All variables, functions and keywords are case-sensitive.
Example:
myVariable = myVariable +1
#increment myVariable
output = MyVariable
#Bug! "MyVariable" is undefined.