Iterators, Set operations, Searching and counting – HP Integrity NonStop H-Series User Manual
Page 88
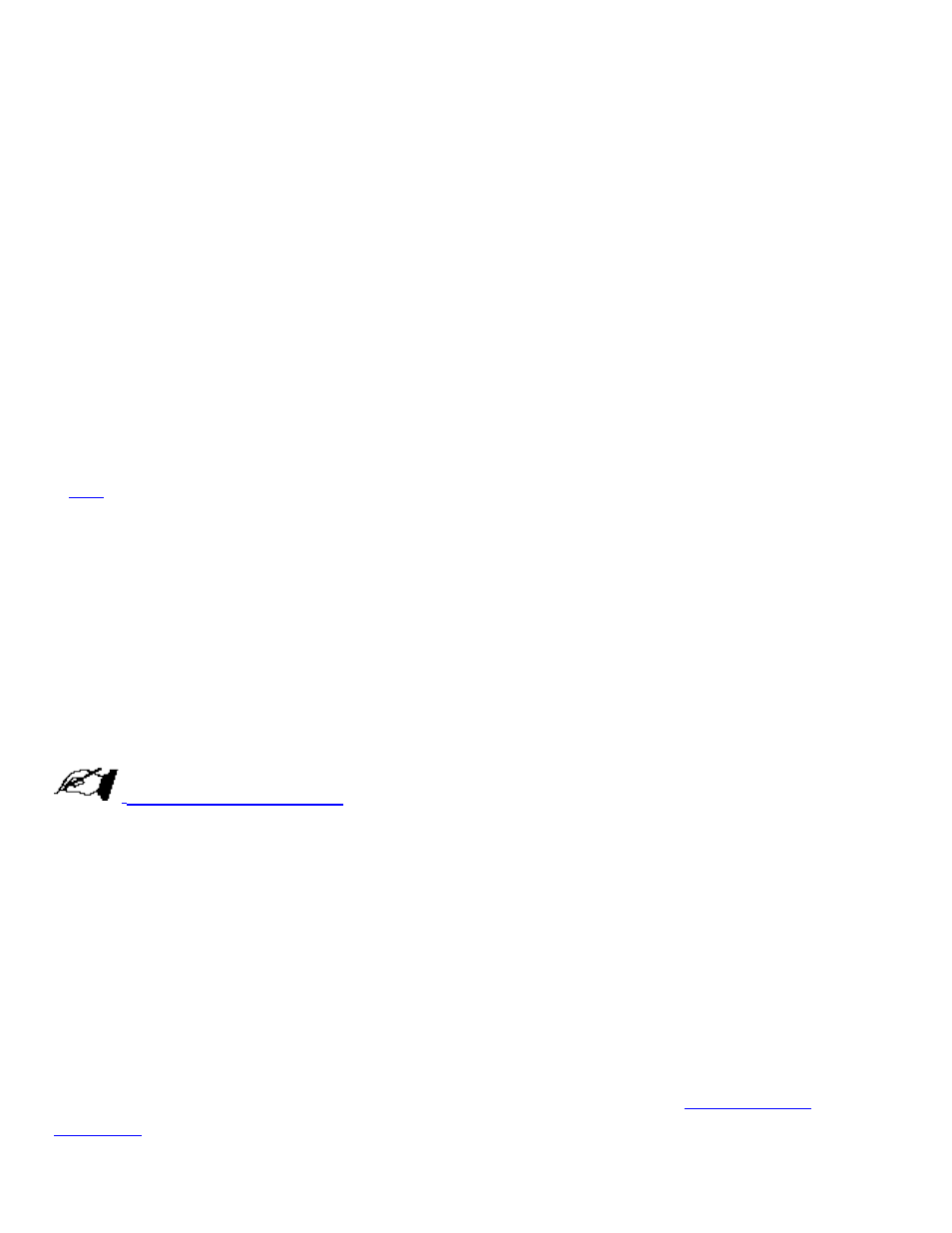
Searching and Counting
The member function size() will yield the number of elements held by a container. The member
function empty() will return a boolean true value if the container is empty, and is generally faster
than testing the size against zero.
The member function find() takes an element value, and returns an iterator denoting the location of
the value in the set if it is present, or a value matching the end-of-set (the value yielded by the
function end()) if it is not. If a multiset contains more than one matching element, the value returned
can be any appropriate value.
set
if (five != set_three.end())
cout << "set contains a five" << endl;
The member functions lower_bound() and upper_bound() are most useful with multisets, as with
sets they simply mimic the function find(). The member function lower_bound() yields the first
entry that matches the argument key, while the member function upper_bound() returns the first
value past the last entry matching the argument. Finally, the member function equal_range() returns
a
pair
of iterators, holding the lower and upper bounds.
The member function count() returns the number of elements that match the argument. For a set this
value is either zero or one, whereas for a multiset it can be any nonnegative value. Since a non-zero
integer value is treated as true, the count() function can be used to test for inclusion of an element, if
all that is desired is to determine whether or not the element is present in the set. The alternative,
using find(), requires testing the result returned by find() against the end-of-collection iterator.
if (set_three.count(5))
cout << "set contains a five" << endl;
Iterators
No Iterator Invalidation
The member functions begin() and end() produce iterators for both sets and multisets. The iterators
produced by these functions are constant to ensure that the ordering relation for the set is not
inadvertently or intentionally destroyed by assigning a new value to a set element. Elements are
generated by the iterators in sequence, ordered by the comparison operator provided when the set
was declared. The member functions rbegin() and rend() produce iterators that yield the elements in
reverse order.
Set Operations
The traditional set operations of subset test, set union, set intersection, and set difference are not
provided as member functions, but are instead implemented as generic algorithms that will work
with any ordered structure. These functions are described in more detail in
. The following summary describes how these functions can be used with the set and
multiset container classes.