HP Integrity NonStop H-Series User Manual
Page 89
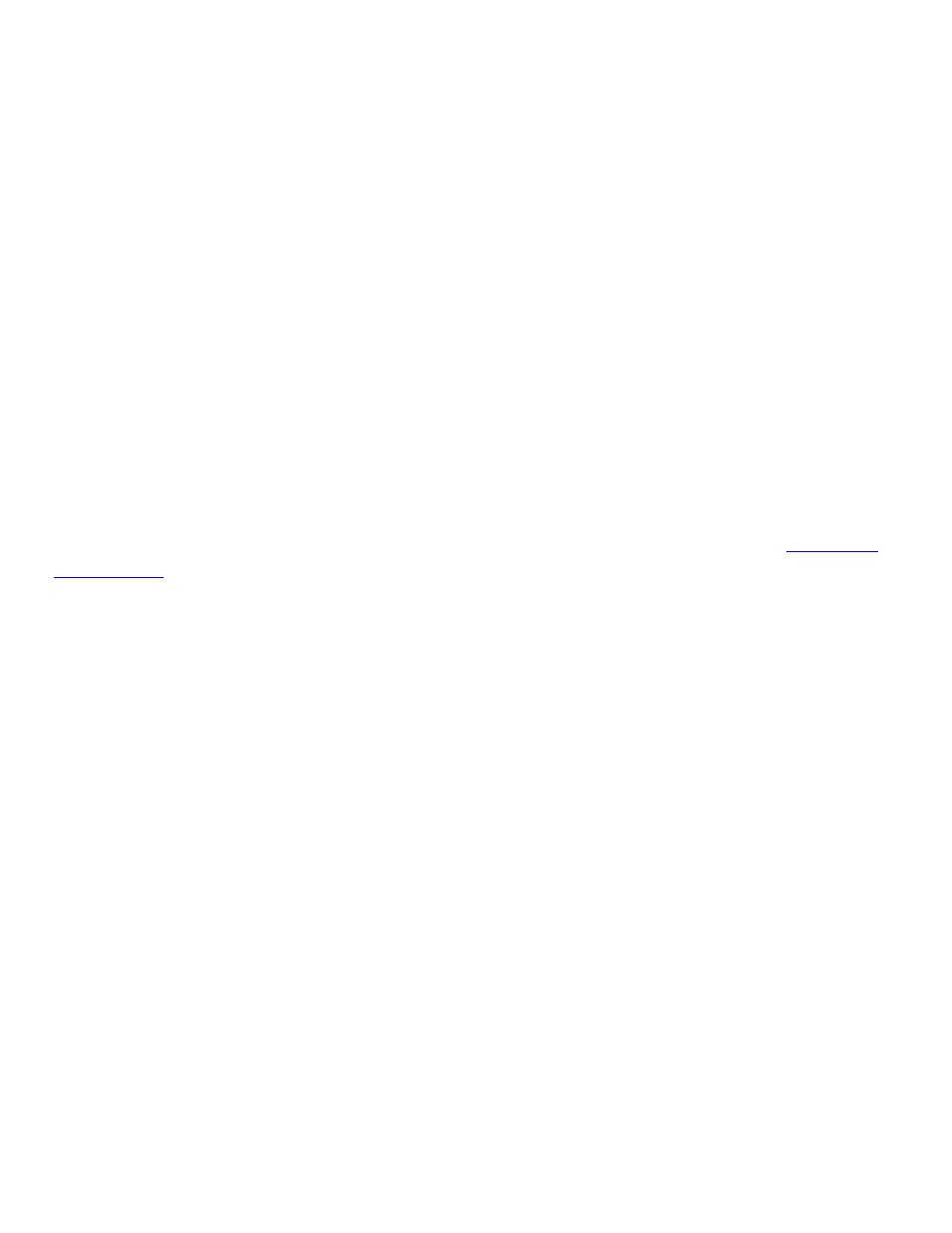
Subset test
The function includes() can be used to determine if one set is a subset of another; that is, if all
elements from the first are contained in the second. In the case of multisets the number of matching
elements in the second set must exceed the number of elements in the first. The four arguments are
a pair of iterators representing the (presumably) smaller set, and a pair of iterators representing the
(potentially) larger set.
if (includes(set_one.begin(), set_one.end(),
set_two.begin(), set_two.end()))
cout << "set_one is a subset of set_two" << endl;
The less than operator (operator <) will be used for the comparison of elements, regardless of the
operator used in the declaration of the set. Where this is inappropriate, an alternative version of the
includes() function is provided. This form takes a fifth argument, which is the comparison function
used to order the elements in the two sets.
Set Union or Intersection
The function set_union() can be used to construct a union of two sets. The two sets are specified by
iterator pairs, and the union is copied into an output iterator that is supplied as a fifth argument. To
form the result as a set, an insert iterator must be used to form the output iterator. (See
for a discussion of insert iterators.) If the desired outcome is a union of one set with
another, then a temporary set can be constructed, and the results swapped with the argument set
prior to deletion of the temporary set.
// union two sets, copying result into a vector
vector
set_union(set_one.begin(), set_one.end(),
set_two.begin(), set_two.end(), v_one.begin());
// form union in place
set
set_union(set_one.begin(), set_one.end(),
set_two.begin(), set_two.end(),
inserter(temp_set, temp_set.begin()));
set_one.swap(temp_set); // temp_set will be deleted
The function set_intersection() is similar, and forms the intersection of the two sets.
As with the includes() function, the less than operator (operator <) is used to compare elements in
the two argument sets, regardless of the operator provided in the declaration of the sets. Should this
be inappropriate, alternative versions of both the set_union() or set_intersection() functions permit
the comparison operator used to form the set to be given as a sixth argument.
The operation of taking the union of two multisets should be distinguished from the operation of
merging two sets. Imagine that one argument set contains three instances of the element 7, and the
second set contains two instances of the same value. The union will contain only three such values,