Iteration, Test for inclusion – HP Integrity NonStop H-Series User Manual
Page 60
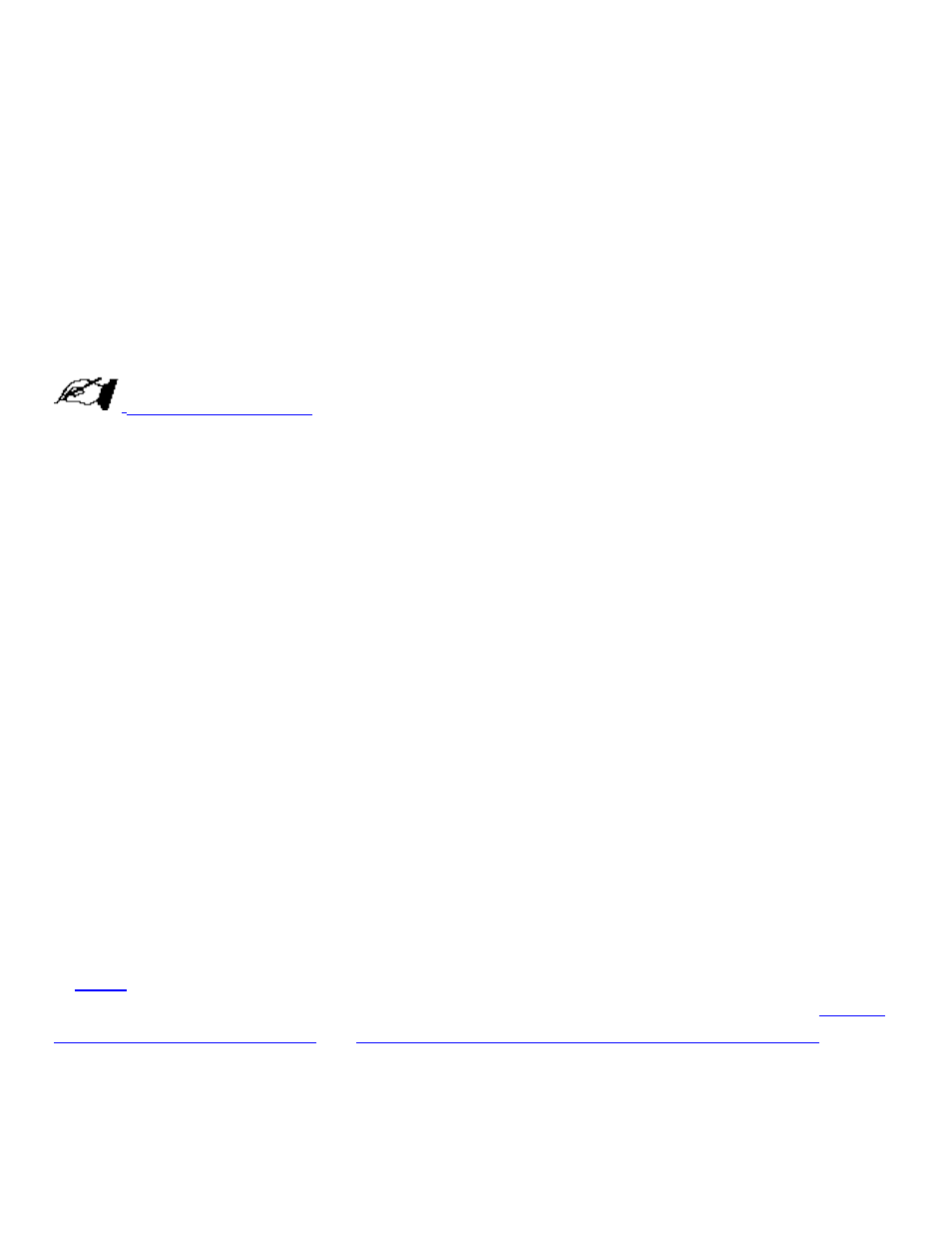
individual insertions, because with a single call at most one allocation will be performed.
// find the location of the 7
vector
find(vec_five.begin(), vec_five.end(), 7);
// then insert the 12 before the 7
vec_five.insert(where, 12);
vec_five.insert(where, 6, 14); // insert six copies of 14
The most general form of the insert() member function takes a position and a pair of iterators that
denote a subsequence from another container. The range of values described by the sequence is
inserted into the vector. Again, because at most a single allocation is performed, using this function
is preferable to using a sequence of individual insertions.
vec_five.insert (where, vec_three.begin(), vec_three.end());
Iterator Invalidation
In addition to the pop_back() member function, which removes elements from the end of a vector, a
function exists that removes elements from the middle of a vector, using an iterator to denote the
location. The member function that performs this task is erase(). There are two forms; the first takes
a single iterator and removes an individual value, while the second takes a pair of iterators and
removes all values in the given range. The size of the vector is reduced, but the capacity is
unchanged. If the container type defines a destructor, the destructor will be invoked on the
eliminated values.
vec_five.erase(where);
// erase from the 12 to the end
where = find(vec_five.begin(), vec_five.end(), 12);
vec_five.erase(where, vec_five.end());
Iteration
The member functions begin() and end() yield random access iterators for the container. Again, we
note that the iterators yielded by these operations can become invalidated after insertions or
removals of elements. The member functions rbegin() and rend() return similar iterators, however
these access the underlying elements in reverse order. Constant iterators are returned if the original
container is declared as constant, or if the target of the assignment or parameter is constant.
Test for Inclusion
A
vector
does not directly provide any method that can be used to determine if a specific value is
contained in the collection. However, the generic algorithms find() or count() (Chapter 13,
Element Satisfying a Condition
Count the Number of Elements that Satisfy a Condition
) can be
used for this purpose. The following statement, for example, tests to see whether an integer vector
contains the element 17.
int num = 0;
count (vec_five.begin(), vec_five.end(), 17, num);