Removing elements – HP Integrity NonStop H-Series User Manual
Page 71
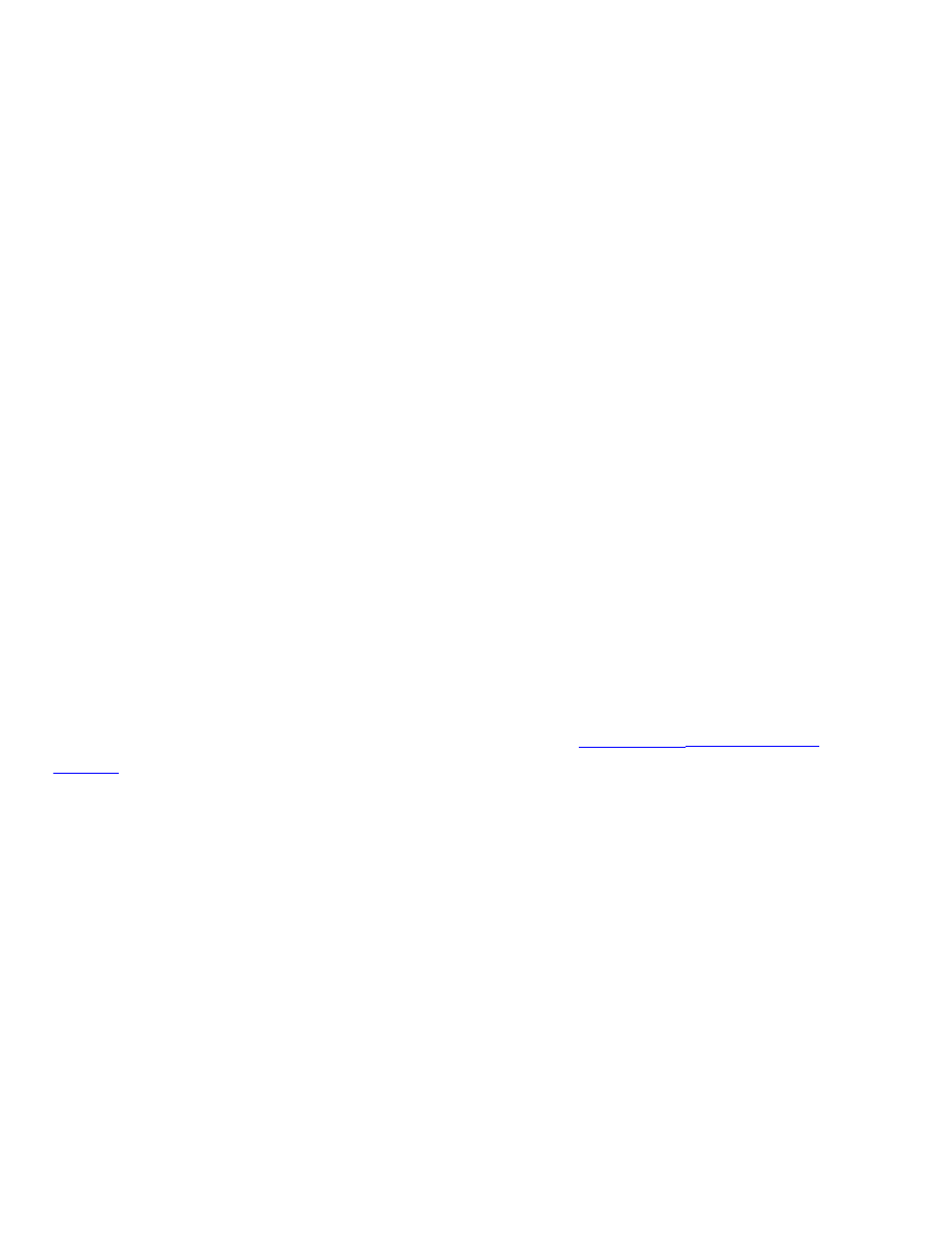
list_eleven.swap(list_twelve);
Removing Elements
Just as there are a number of different ways to insert an element into a list, there are a variety of ways
to remove values from a list. The most common operations used to remove a value are pop_front() or
pop_back(), which delete the single element from the front or the back of the list, respectively. These
member functions simply remove the given element, and do not themselves yield any useful result. If
a destructor is defined for the element type it will be invoked as the element is removed. To look at
the values before deletion, use the member functions front() or back().
The erase() operation can be used to remove a value denoted by an iterator. For a list, the argument
iterator, and any other iterators that denote the same location, become invalid after the removal, but
iterators denoting other locations are unaffected. We can also use erase() to remove an entire
subsequence, denoted by a pair of iterators. The values beginning at the initial iterator and up to, but
not including, the final iterator are removed from the list. Erasing elements from the middle of a list
is an efficient operation, unlike erasing elements from the middle of a vector or a deque.
list_nine.erase (location);
// erase values between the first occurrence of 5
// and the following occurrence of 7
list
location = find(list_nine.begin(), list_nine.end(), 5);
list
find(location, list_nine.end(), 7);
list_nine.erase (location, location2);
The remove() member function removes all occurrences of a given value from a list. A variation,
remove_if(), removes all values that satisfy a given predicate. An alternative to the use of either of
these is to use the remove() or remove_if() generic algorithms (
). The generic algorithms do not reduce the size of the list, instead they move the elements to
be retained to the front of the list, leave the remainder of the list unchanged, and return an iterator
denoting the location of the first unmodified element. This value can be used in conjunction with the
erase() member function to remove the remaining values.
list_nine.remove(4); // remove all fours
list_nine.remove_if(divisibleByThree); //remove any div by 3
// the following is equivalent to the above
list
remove_if(list_nine.begin(), list_nine.end(),
divisibleByThree);
list_nine.erase(location3, list_nine.end());
The operation unique() will erase all but the first element from every consecutive group of equal
elements in a list. The list need not be ordered. An alternative version takes a binary function, and
compares adjacent elements using the function, removing the second value in those situations were
the function yields a true value. As with remove_if(), not all compilers support the second form of