HP Integrity NonStop H-Series User Manual
Page 146
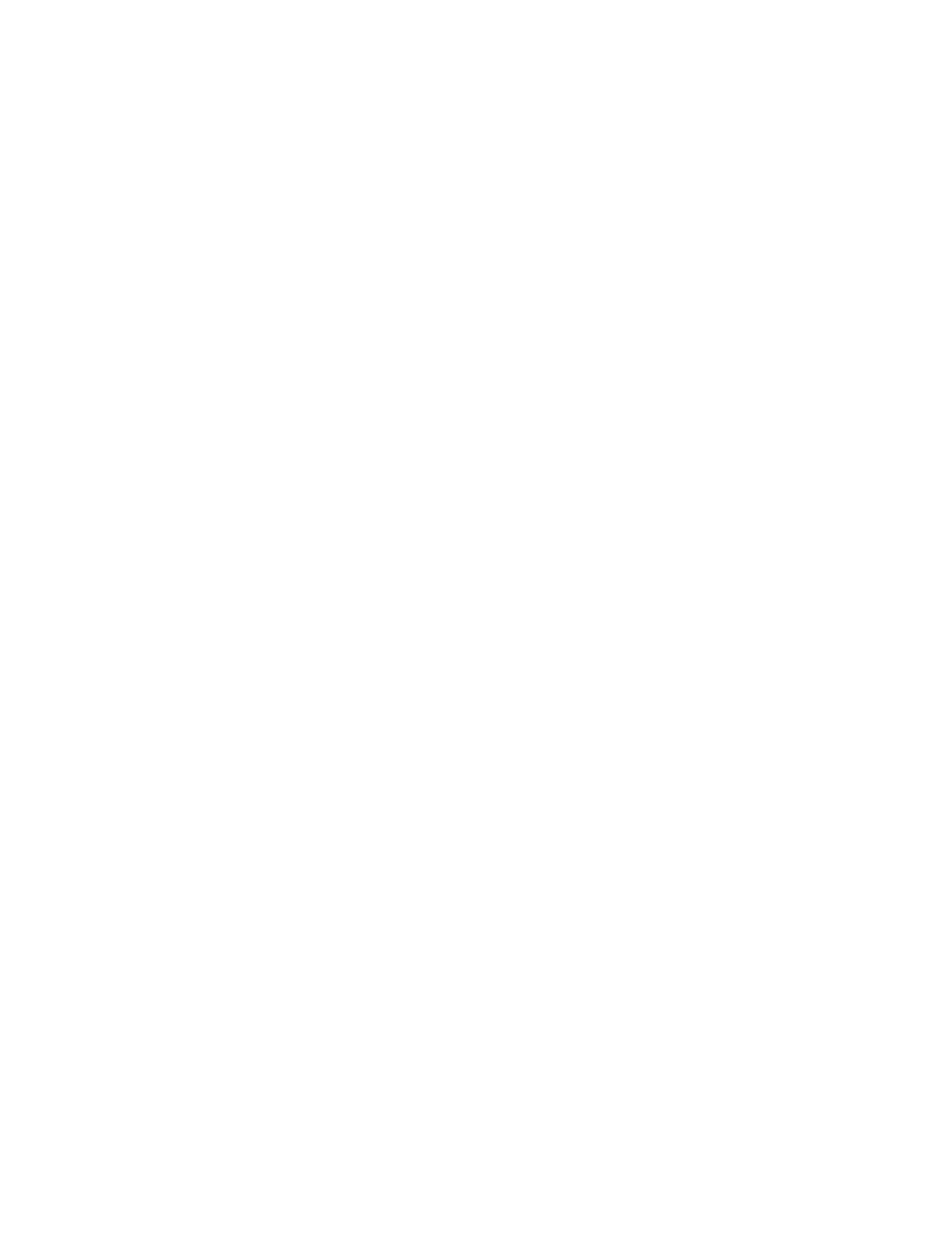
Adding elements into a sequence
●
Copying a sequence from input or to output
●
Converting a sequence from one form into another
●
These are illustrated in the following sample program.
void copy_example()
// illustrate the use of the copy algorithm
{
char * source = "reprise";
char * surpass = "surpass";
char buffer[120], * bufferp = buffer;
// example 1, a simple copy
copy (source, source + strlen(source) + 1, bufferp);
// example 2, self copies
copy (bufferp + 2, bufferp + strlen(buffer) + 1, bufferp);
int buflen = strlen(buffer) + 1;
copy_backward (bufferp, bufferp + buflen, bufferp + buflen + 3);
copy (surpass, surpass + 3, bufferp);
// example 3, copy to output
copy (bufferp, bufferp + strlen(buffer),
ostream_iterator
cout << endl;
// example 4, use copy to convert type
list
copy (bufferp, bufferp + strlen(buffer),
inserter(char_list, char_list.end()));
char * big = "big ";
copy (big, big + 4, inserter(char_list, char_list.begin()));
char buffer2 [120], * buffer2p = buffer2;
* copy (char_list.begin(), char_list.end(), buffer2p) = '\0';
cout << buffer2 << endl;
}
The first call on copy(), in example 1, simply copies the string pointed to by the variable source into
a buffer, resulting in the buffer containing the text "reprise". Note that the ending position for the
copy is one past the terminating null character, thus ensuring the null character is included in the
copy operation.
The copy() operation is specifically designed to permit self-copies, i.e., copies of a sequence onto
itself, as long as the destination iterator does not fall within the range formed by the source iterators.
This is illustrated by example 2. Here the copy begins at position 2 of the buffer and extends to the
end, copying characters into the beginning of the buffer. This results in the buffer holding the value