Reverse iterators – HP Integrity NonStop H-Series User Manual
Page 31
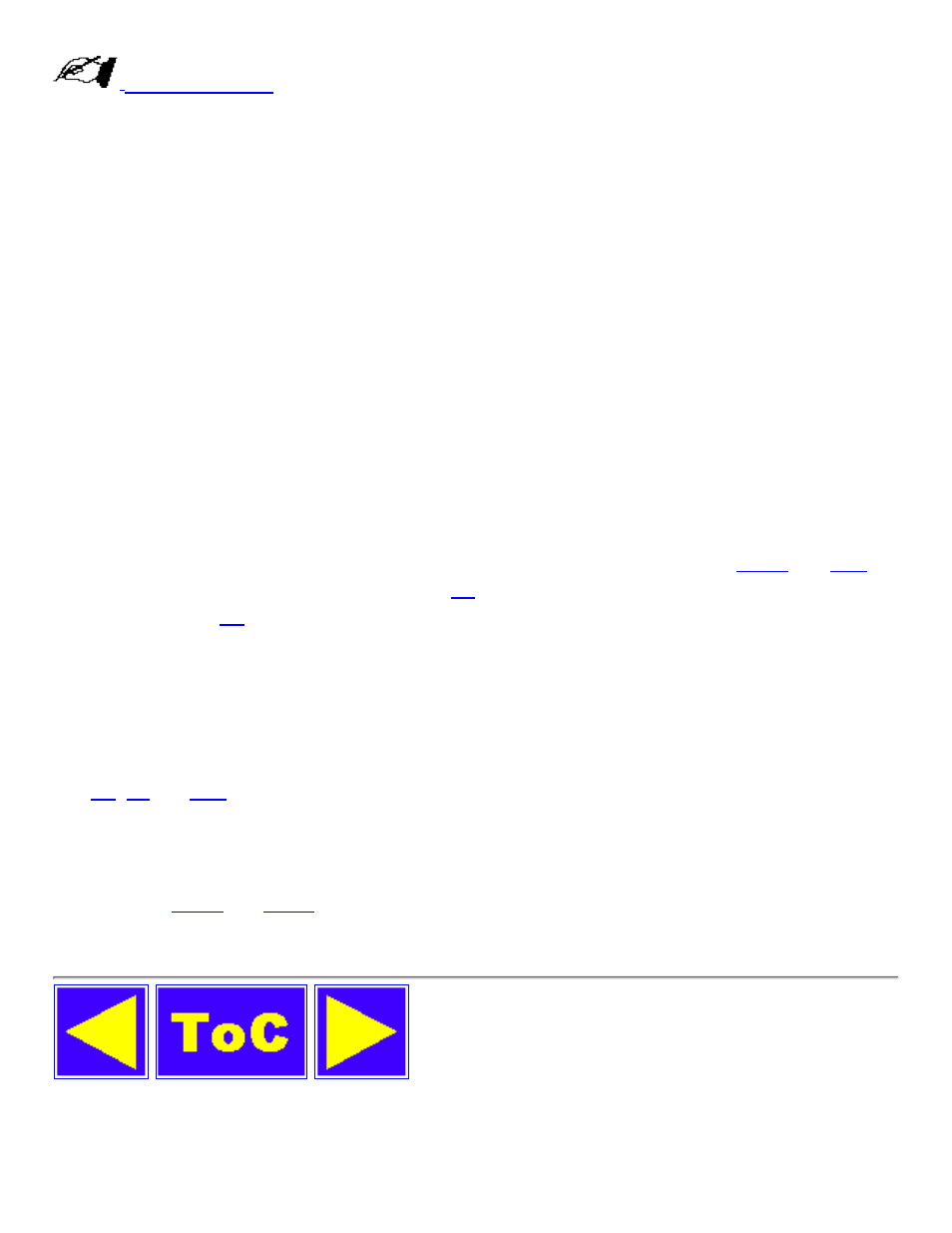
randomInteger()
The program will cycle as long as first is denoting a position that occurs earlier in the sequence than
the one denoted by last. Only random access iterators can be compared using relational operators; all
other iterators can be compared only for equality or inequality. On each cycle through the loop, the
expression last - first yields the number of elements between the two limits. The function
randomInteger() is assumed to generate a random number between 0 and the argument. Using the
standard random number generator, this function could be written as follows:
unsigned int randomInteger (unsigned int n)
// return random integer greater than
// or equal to 0 and less than n
{
return rand() % n;
}
This random value is added to the iterator first, resulting in an iterator to a randomly selected value in
the container. This value is then swapped with the element denoted by the iterator first.
Reverse Iterators
An iterator naturally imposes an order on an underlying container of values. For a
vector
or a
map
the
order is given by increasing index values. For a
set
it is the increasing order of the elements held in
the container. For a
list
the order is explicitly derived from the way values are inserted.
A reverse iterator will yield values in exactly the reverse order of those given by the standard
iterators. That is, for a vector or a list, a reverse iterator will generate the last element first, and the
first element last. For a set it will generate the largest element first, and the smallest element last.
Strictly speaking, reverse iterators are not themselves a new category of iterator. Rather, there are
reverse bidirectional iterators, reverse random access iterators, and so on.
The
list
,
set
and
map
data types provide a pair of member functions that produce reverse bidirectional
iterators. The functions rbegin() and rend() generate iterators that cycle through the underlying
container in reverse order. Increments to such iterators move backward, and decrements move
forward through the sequence.
Similarly, the
vector
and
deque
data types provide functions (also named rbegin() and rend()) that
produce reverse random access iterators. Subscript and addition operators, as well as increments to
such iterators move backward within the sequence.