HP Integrity NonStop H-Series User Manual
Page 70
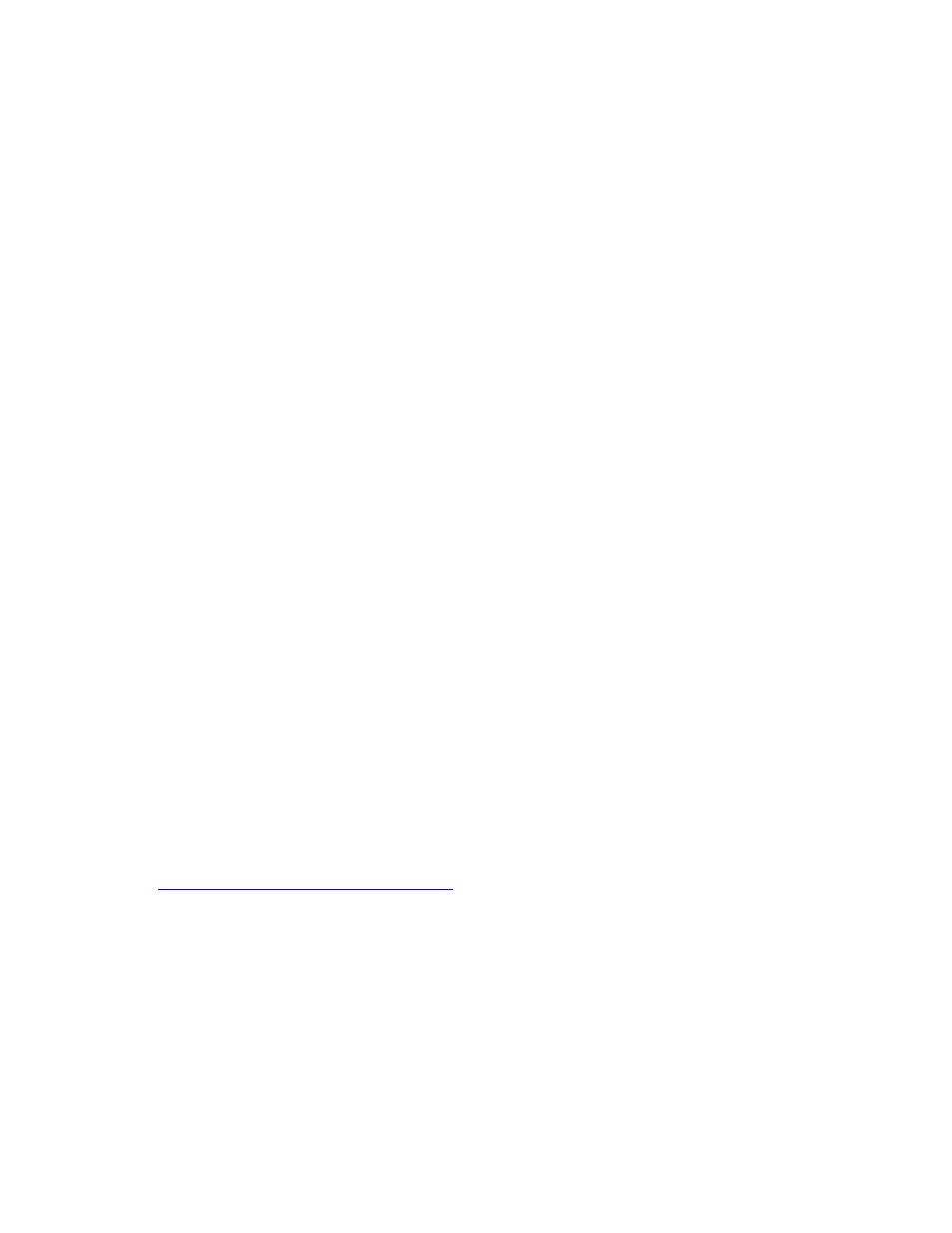
an iterator denoting the location of the inserted value. This result value was ignored in the invocations
shown above.
// find the location of the first occurrence of the
// value 5 in list
list
find(list_nine.begin(), list_nine.end(), 5);
// and insert an 11 immediate before it
location = list_nine.insert(location, 11);
It is also possible to insert a fixed number of copies of an argument value. This form of insert() does
not yield the location of the values.
line_nine.insert (location, 5, 12); // insert five twelves
Finally, an entire sequence denoted by an iterator pair can be inserted into a list. Again, no useful
value is returned as a result of the insert().
// insert entire contents of list_ten into list_nine
list_nine.insert (location, list_ten.begin(), list_ten.end());
There are a variety of ways to splice one list into another. A splice differs from an insertion in that the
item is simultaneously added to the receiver list and removed from the argument list. For this reason,
a splice can be performed very efficiently, and should be used whenever appropriate. As with an
insertion, the member function splice() uses an iterator to indicate the location in the receiver list
where the splice should be made. The argument is either an entire list, a single element in a list
(denoted by an iterator), or a subsequence of a list (denoted by a pair of iterators).
// splice the last element of list ten
list_nine.splice (location, list_ten, list_ten.end());
// splice all of list ten
list_nine.splice (location, list_ten);
// splice list 9 back into list 10
list_ten.splice (list_ten.begin(), list_nine,
list_nine.begin(), location);
Two ordered lists can be combined into one using the merge() operation. Values from the argument
list are merged into the ordered list, leaving the argument list empty. The merge is stable; that is,
elements retain their relative ordering from the original lists. As with the generic algorithm of the
same name (
), two forms are supported. The second form uses the
binary function supplied as argument to order values. Not all compilers support the second form. If
the second form is desired and not supported, the more general generic algorithm can be used,
although this is slightly less efficient.
// merge with explicit compare function
list_eleven.merge(list_six, widgetCompare);
//the following is similar to the above
list
merge (list_eleven.begin(), list_eleven.end(),
list_six.begin(), list_six.end(),
inserter(list_twelve, list_twelve.begin()), widgetCompare);