Removal of elements from a set, Searching and counting – HP Integrity NonStop H-Series User Manual
Page 87
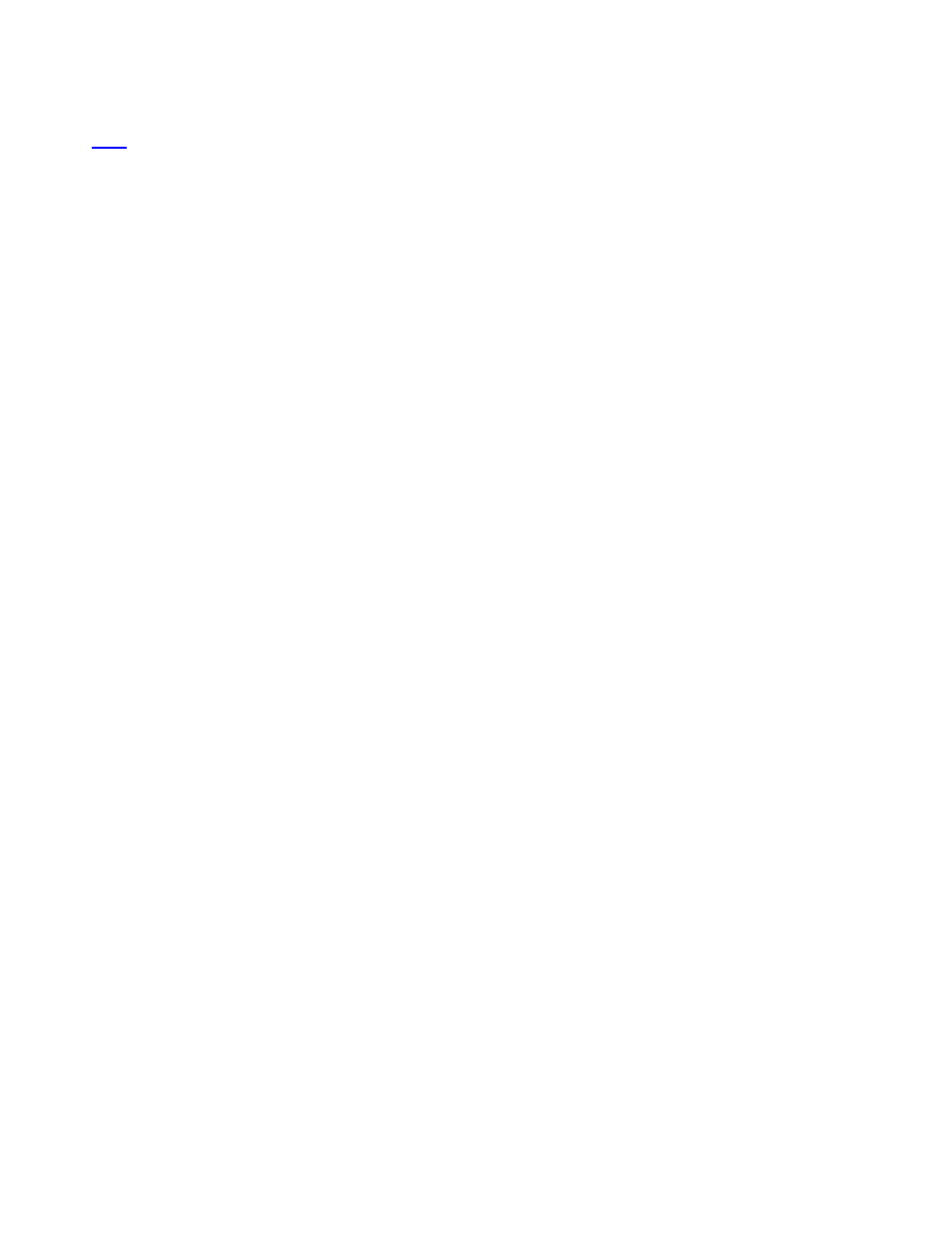
Insertions of several elements from another container can also be performed using an iterator pair:
set_one.insert (set_three.begin(), set_three.end());
The
pair
data structure is a tuple of values. The first value is accessed through the field name first,
while the second is, naturally, named second. A function named make_pair() simplifies the task of
producing an instance of class pair.
template
struct pair {
T1 first;
T2 second;
pair (const T1 & x, const T2 & y) : first(x), second(y) { }
};
template
inline pair
{ return pair
In determining the equivalence of keys, for example, to determine if the key portion of a new
element matches any existing key, the comparison function for keys is used, and not the equivalence
(==) operator. Two keys are deemed equivalent if the comparison function used to order key values
yields false in both directions. That is, if Compare(key1, key2) is false, and if Compare(key2, key1)
is false, then key1 and key2 are considered equivalent.
Removal of Elements from a Set
Values are removed from a set using the member function erase(). The argument can be either a
specific value, an iterator that denotes a single value, or a pair of iterators that denote a range of
values. When the first form is used on a multiset, all arguments matching the argument value are
removed, and the return value indicates the number of elements that have been erased.
// erase element equal to 4
set_three.erase(4);
// erase element five
set
set_three.erase(five);
// erase all values between seven and eleven
set
set
set_three.erase (seven, eleven);
If the underlying element type provides a destructor, then the destructor will be invoked prior to
removing the element from the collection.