Set operations, Conversions – HP Integrity NonStop H-Series User Manual
Page 94
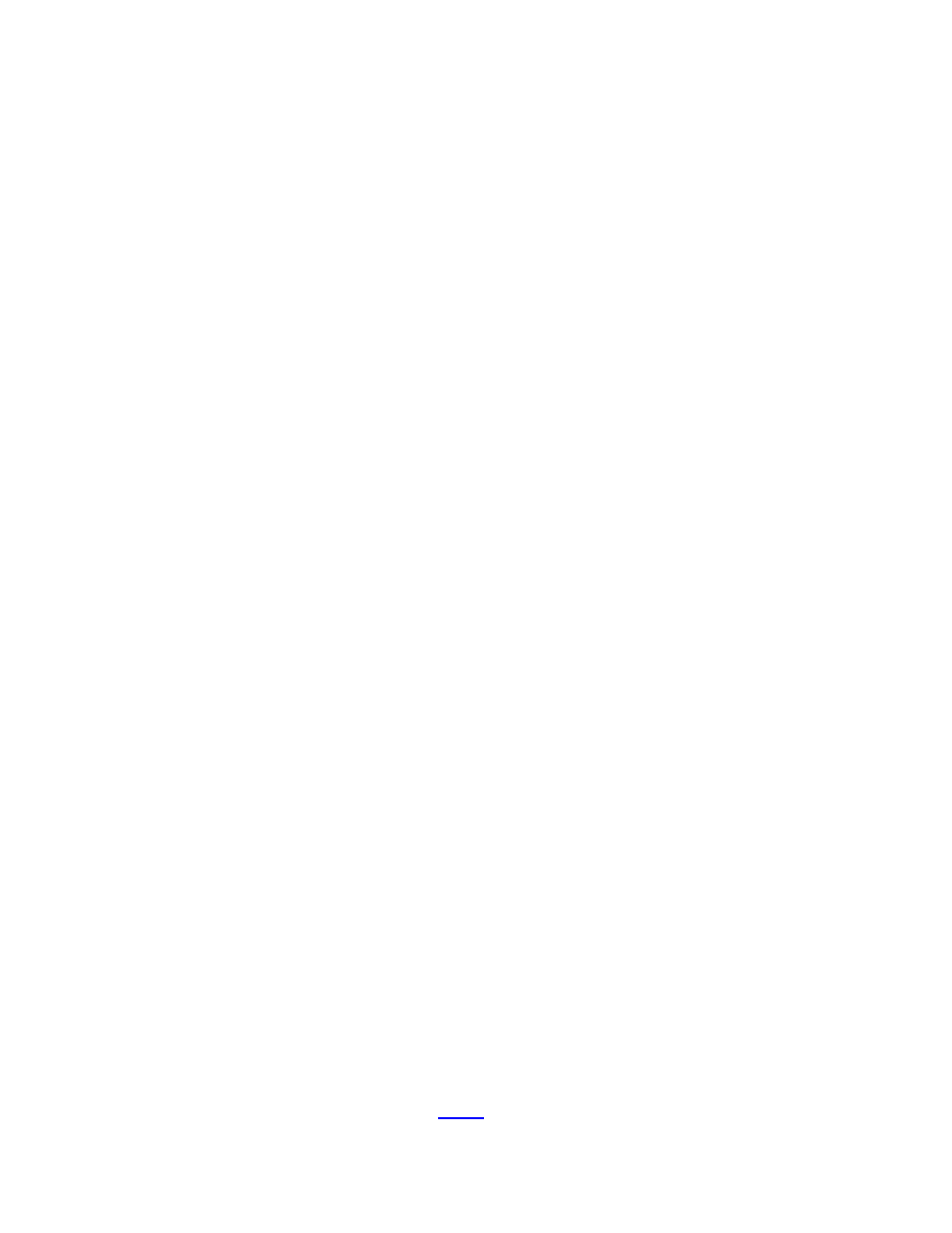
bset_one[3] = 1;
if (bset_one.test(4))
cout << "bit position 4 is set" << endl;
if (bset_one.any())
cout << "some bit position is set" << endl;
if (bset_one.none()) cout << "no bit position is set" << endl;
The function set() can be used to set a specific bit. bset_one.set(i) is equivalent to bset_one[i] =
true. Invoking the function without any arguments sets all bit positions to true. The function
reset() is similar, and sets the indicated positions to false (sets all positions to false if invoked
with no argument). The function flip() flips either the indicated position, or all positions if no
argument is provided. The function flip() is also provided as a member function for the
individual bit references.
bset_one.flip(); // flip the entire set
bset_one.flip(12); // flip only bit 12
bset_one[12].flip(); // reflip bit 12
The member function size() returns the size of the bitset, while the member function count()
yields the number of bits that are set.
Set operations
Set operations on bitsets are implemented using the bit-wise operators, in a manner analogous to
the way in which the same operators act on integer arguments.
The negation operator (operator ~) applied to a bitset returns a new bitset containing the inverse
of elements in the argument set.
The intersection of two bitsets is formed using the and operator (operator &). The assignment
form of the operator can be used. In the assignment form, the target becomes the disjunction of
the two sets.
bset_three = bset_two & bset_four;
bset_five &= bset_three;
The union of two sets is formed in a similar manner using the or operator (operator |). The
exclusive-or is formed using the bit-wise exclusive or operator (operator ^).
The left and right shift operators (operator << and >>) can be used to shift a bitset left or right, in
a manner analogous to the use of these operators on integer arguments. If a bit is shifted left by
an integer value n, then the new bit position i is the value of the former i-n. Zeros are shifted into
the new positions.
Conversions
The member function to_ulong() converts a
bitset
into an unsigned long. It is an error to perform
this operation on a bitset containing more elements than will fit into this representation.