Rotate elements around a midpoint, Chapter 13, And partitions – HP Integrity NonStop H-Series User Manual
Page 159
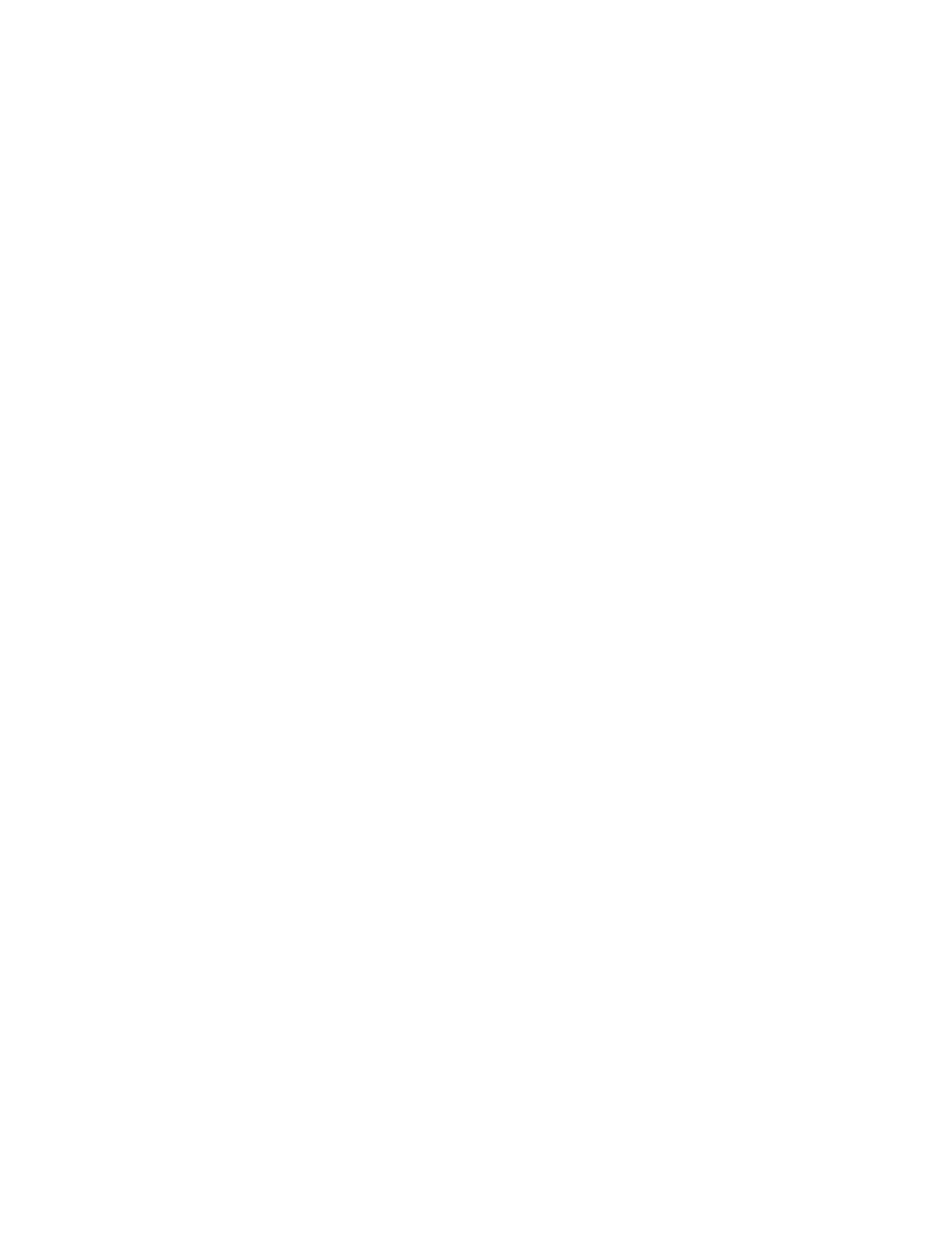
replace_if (numbers.begin(), numbers.end(), isEven, 9);
// illustrate copy versions of replace
int aList[] = {2, 1, 4, 3, 2, 5};
int bList[6], cList[6], j;
replace_copy (aList, aList+6, &bList[0], 2, 7);
replace_copy_if (bList, bList+6, &cList[0],
bind2nd(greater
}
The example program also illustrates the use of the replace_copy algorithms. First, an array
containing the values 2 1 4 3 2 5 is created. This is modified by replacing the 2 values with 7,
resulting in the array 7 1 4 3 7 5. Next, all values larger than 3 are replaced with the value 8,
resulting in the array values 8 1 8 3 8 8. In the latter case the bind2nd() adaptor is used, to modify
the binary greater-than function by binding the 2nd argument to the constant value 3, thereby
creating the unary function x > 3.
Rotate Elements Around a Midpoint
A rotation of a sequence divides the sequence into two sections, then swaps the order of the
sections, maintaining the relative ordering of the elements within the two sections. Suppose, for
example, that we have the values 1 to 10 in sequence.
1 2 3 4 5 6 7 8 9 10
If we were to rotate around the element 7, the values 7 to 10 would be moved to the beginning,
while the elements 1 to 6 would be moved to the end. This would result in the following sequence.
7 8 9 10 1 2 3 4 5 6
When you invoke the algorithm rotate(), the starting point, midpoint, and past-the-end location are
all denoted by forward iterators:
void rotate (ForwardIterator first, ForwardIterator middle,
ForwardIterator last);
The prefix portion, the set of elements following the start and not including the midpoint, is
swapped with the suffix, the set of elements between the midpoint and the past-the-end location.
Note, as in the illustration presented earlier, that these two segments need not be the same length.
void rotate_example()
// illustrate the use of the rotate algorithm
{
// create the list 1 2 3 ... 10
list
generate_n(inserter(iList, iList.begin()), 10, iotaGen(1));
// find the location of the seven
list
find(iList.begin(), iList.end(), 7);