Locate maximum or minimum element – HP Integrity NonStop H-Series User Manual
Page 153
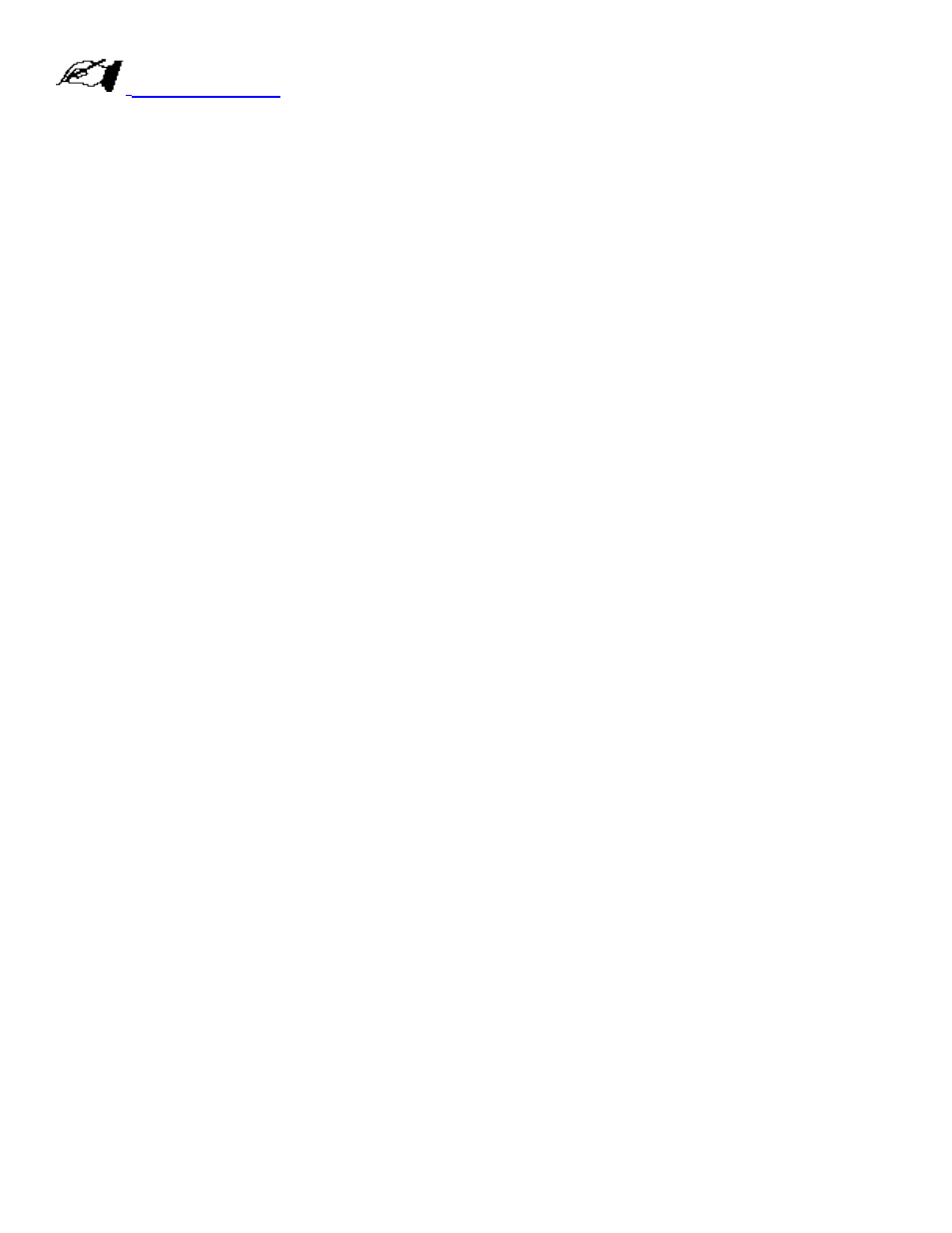
Speed of Search
Suppose, for example, that we wish to discover the location of the string "ration" in the string
"dreams and aspirations". The solution to this problem is shown in the example program. If no
appropriate match is found, the value returned is the past-the-end iterator for the first sequence.
void search_example ()
// illustrate the use of the search algorithm
{
char * base = "dreams and aspirations";
char * text = "ration";
char * where = search(base, base + strlen(base),
text, text + strlen(text));
if (*where != '\0')
cout << "substring position: " << where - base << endl;
else
cout << "substring does not occur in text" << endl;
}
Note that this algorithm, unlike many that manipulate two sequences, uses a starting and ending
iterator pair for both sequences, not just the first sequence.
Like the algorithms equal() and mismatch(), an alternative version of search() takes an optional
binary predicate that is used to compare elements from the two sequences.
Locate Maximum or Minimum Element
The functions max() and min() can be used to find the maximum and minimum of a pair of
values. These can optionally take a third argument that defines the comparison function to use
in place of the less-than operator (operator <). The arguments are values, not iterators:
template
const T& max(const T& a, const T& b [, Compare ] );
template
const T& min(const T& a, const T& b [, Compare ] );
The maximum and minimum functions are generalized to entire sequences by the generic
algorithms max_element() and min_element(). For these functions the arguments are input
iterators.
ForwardIterator max_element (ForwardIterator first,
ForwardIterator last [, Compare ] );
ForwardIterator min_element (ForwardIterator first,
ForwardIterator last [, Compare ] );