Partial sums – HP Integrity NonStop H-Series User Manual
Page 174
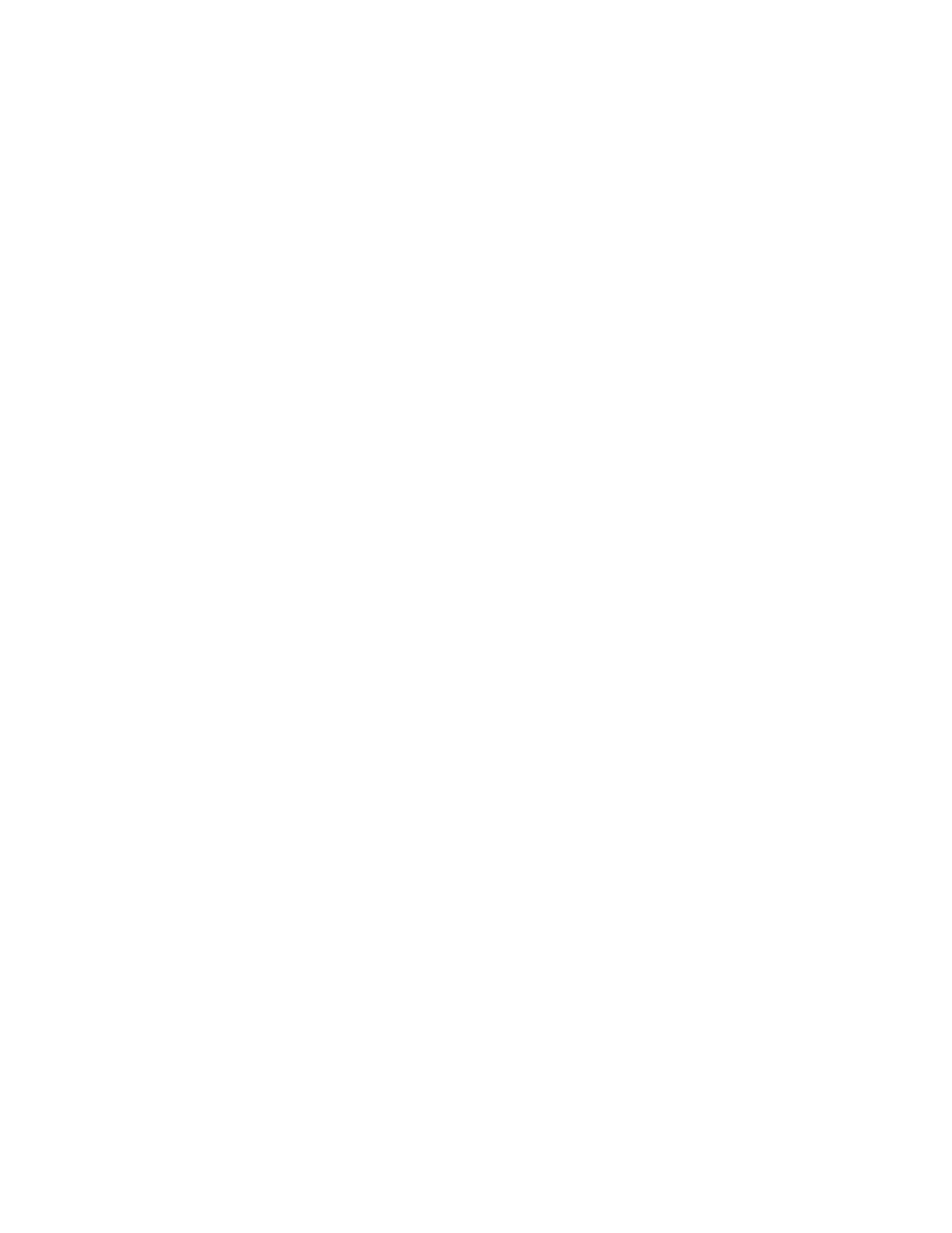
int square(int n) { return n * n; }
void transform_example ()
// illustrate the use of the transform algorithm
{
// generate a list of value 1 to 6
list
generate_n (inserter(aList, aList.begin()), 6, iotaGen(1));
// transform elements by squaring, copy into vector
vector
transform (aList.begin(), aList.end(), aVec.begin(), square);
// transform vector again, in place, yielding 4th powers
transform (aVec.begin(), aVec.end(), aVec.begin(), square);
// transform in parallel, yielding cubes
vector
transform (aVec.begin(), aVec.end(), aList.begin(),
cubes.begin(), divides
}
Partial Sums
A partial sum of a sequence is a new sequence in which every element is formed by adding the
values of all prior elements. For example, the partial sum of the vector 1 3 2 4 5 is the new vector
1 4 6 10 15. The element 4 is formed from the sum 1 + 3, the element 6 from the sum 1 + 3 + 2,
and so on. Although the term "sum" is used in describing the operation, the binary function can, in
fact, be any arbitrary function. The example program illustrates this by computing partial
products. The arguments to the partial sum function are described as follows:
OutputIterator partial_sum
(InputIterator first, InputIterator last,
OutputIterator result [, BinaryFunction] );
By using the same value for both the input iterator and the result the partial sum can be changed
into an in-place transformation.
void partial_sum_example ()
// illustrate the use of the partial sum algorithm
{
// generate values 1 to 5
vector
generate (aVec.begin(), aVec.end(), iotaGen(1));
// output partial sums
partial_sum (aVec.begin(), aVec.end(),
ostream_iterator