HP Integrity NonStop H-Series User Manual
Page 35
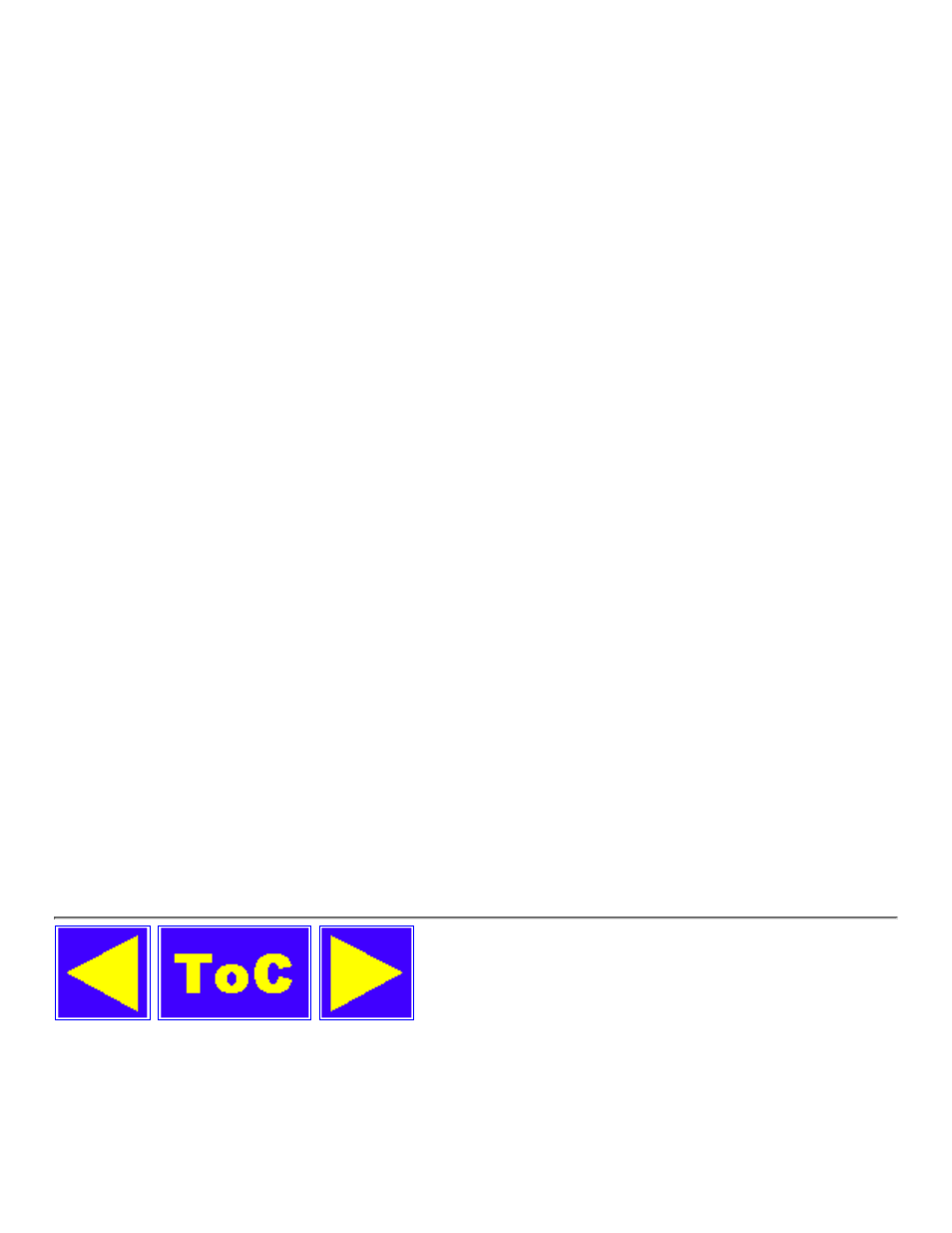
The following simple program illustrates the use of all three forms of insert iterators. First, the
values 3, 2 and 1 are inserted into the front of an initially empty list. Note that as it is inserted, each
value becomes the new front, so that the resultant list is ordered 1, 2, 3. Next, the values 7, 8 and 9
are inserted into the end of the list. Finally, the find() operation is used to locate an iterator that
denotes the 7 value, and the numbers 4, 5 and 6 are inserted immediately prior. The result is the list
of numbers from 1 to 9 in order.
void main() {
int threeToOne [ ] = {3, 2, 1};
int fourToSix [ ] = {4, 5, 6};
int sevenToNine [ ] = {7, 8, 9};
list
// first insert into the front
// note that each value becomes new front
copy (threeToOne, threeToOne+3, front_inserter(aList));
// then insert into the back
copy (sevenToNine, sevenToNine+3, back_inserter(aList));
// find the seven, and insert into middle
list
copy (fourToSix, fourToSix+3, inserter(aList, seven));
// copy result to output
copy (aList.begin(), aList.end(),
ostream_iterator
cout << endl;
}
Observe that there is an important and subtle difference between the iterators created by
inserter(aList, aList.begin()) and front_inserter(aList). The call on inserter(aList, aList.begin())
copies values in sequence, adding each one to the front of a list, whereas front_inserter(aList) copies
values making each value the new front. The result is that front_inserter(aList) reverses the order of
the original sequence, while inserter(aList, aList.begin()) retains the original order.