HP Integrity NonStop H-Series User Manual
Page 166
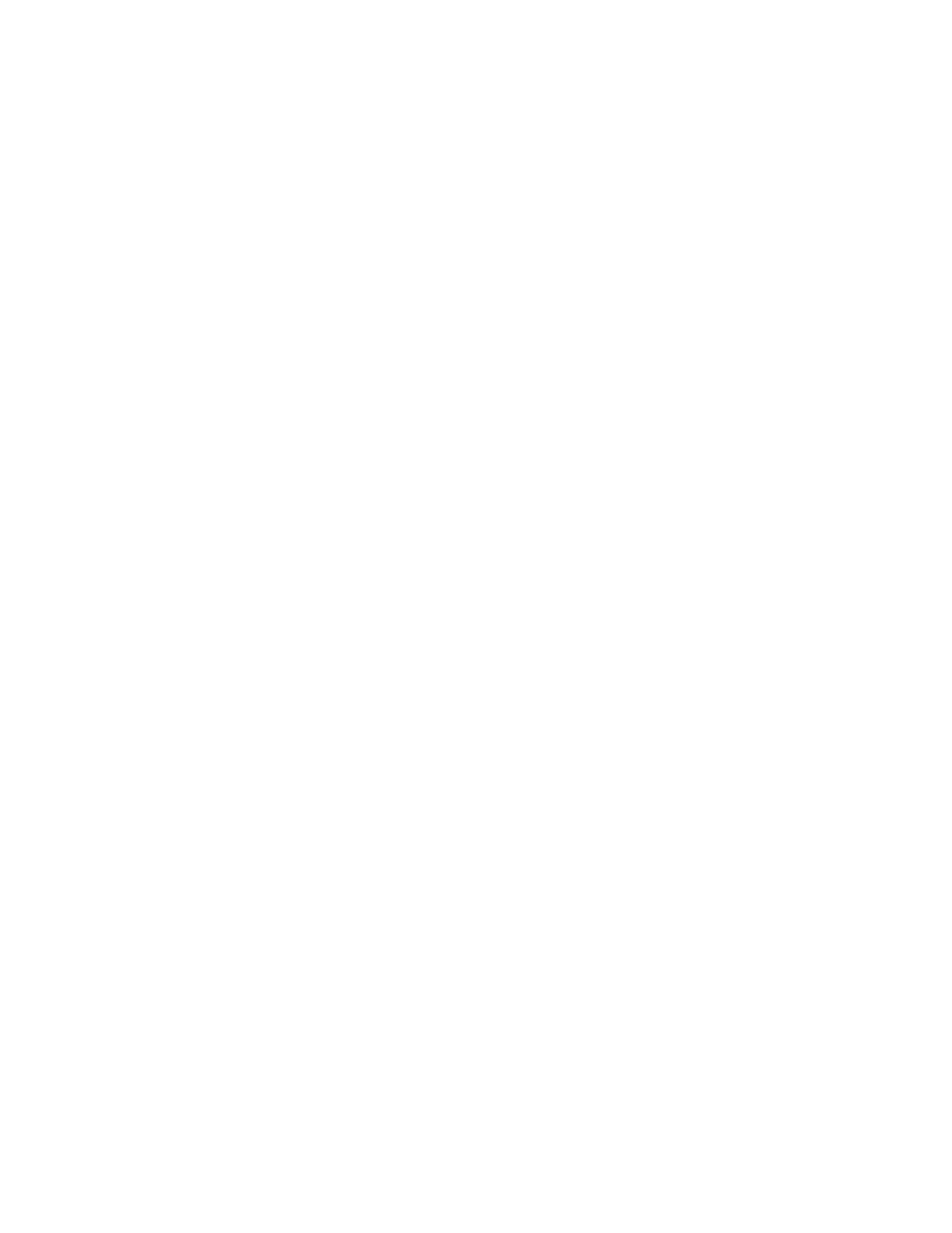
ForwardIterator remove
(ForwardIterator first, ForwardIterator last, const T &);
ForwardIterator remove_if
(ForwardIterator first, ForwardIterator last, Predicate);
The algorithm remove() copies values to the front of the sequence, overwriting the location of
the removed elements. All elements not removed remain in their relative order. Once all values
have been examined, the remainder of the sequence is left unchanged. The iterator returned as
the result of the operation provides the end of the new sequence. For example, eliminating the
element 2 from the sequence 1 2 4 3 2 results in the sequence 1 4 3 3 2, with the iterator
returned as the result pointing at the second 3. This value can be used as argument to erase() in
order to eliminate the remaining elements (the 3 and the 2), as illustrated in the example
program.
A copy version of the algorithms copies values to an output sequence, rather than making
transformations in place.
OutputIterator remove_copy
(InputIterator first, InputIterator last,
OutputIterator result, const T &);
OutputIterator remove_copy_if
(InputIterator first, InputIterator last,
OutputIterator result, Predicate);
The use of remove() is shown in the following program.
void remove_example ()
// illustrate the use of the remove algorithm
{
// create a list of numbers
int data[] = {1, 2, 4, 3, 1, 4, 2};
list
copy (data, data+7, inserter(aList, aList.begin()));
// remove 2's, copy into new list
list
remove_copy (aList.begin(), aList.end(),
back_inserter(newList), 2);
// remove 2's in place
list
where = remove (aList.begin(), aList.end(), 2);
aList.erase(where, aList.end());
// remove all even values
where = remove_if (aList.begin(), aList.end(), isEven);