HP Integrity NonStop H-Series User Manual
Page 103
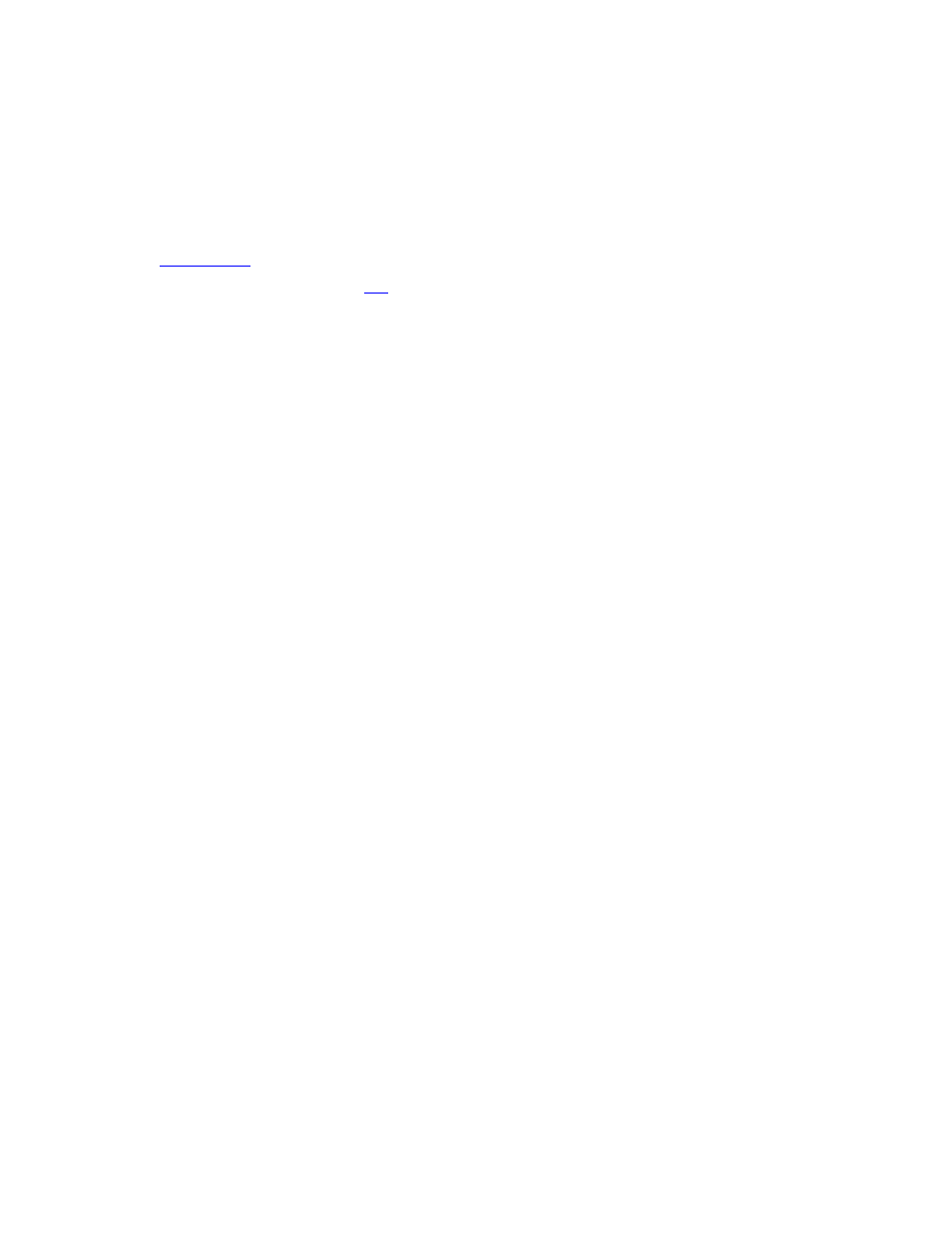
Simple operations on our database are directly implemented by map commands. Adding an element
to the database is simply an insert, removing an element is an erase, and updating is a combination of
the two. To print all the entries in the database we can use the for_each() algorithm, and apply the
following simple utility routine to each entry:
void printEntry(const entry_type & entry)
{ cout << entry.first << ":" << entry.second << endl; }
We will use a pair of slightly more complex operations to illustrate how a few of the algorithms
described in
can be used with maps. Suppose we wanted to display all the phone numbers
with a certain three digit initial prefix
[1]
. We will use the find_if() function (which is different from
the find() member function in class map) to locate the first entry. Starting from this location,
subsequent calls on find_if() will uncover each successive entry.
void telephoneDirectory::displayPrefix(int prefix)
{
cout << "Listing for prefix " << prefix << endl;
friendMap::iterator where;
where =
find_if (database.begin(), database.end(),
checkPrefix(prefix));
while (where != database.end()) {
printEntry(*where);
where = find_if (++where, database.end(),
checkPrefix(prefix));
}
cout << "end of prefix listing" << endl;
}
For the predicate to this operation, we require a boolean function that takes only a single argument
(the pair representing a database entry), and tells us whether or not it is in the given prefix. There is
no obvious candidate function, and in any case the test prefix is not being passed as an argument to
the comparison function. The solution to this problem is to employ a technique that is commonly
used with the standard library, defining the predicate function as an instance of a class, and storing
the test predicate as an instance variable in the class, initialized when the class is constructed. The
desired function is then defined as the function call operator for the class:
int prefix(const entry_type & entry)
{ return entry.second / 10000; }
class checkPrefix {
public:
checkPrefix (int p) : testPrefix(p) { }
int testPrefix;
bool operator () (const entry_type & entry)
{ return prefix(entry) == testPrefix; }
};
Our final example will be to display the directory sorted by prefix. It is not possible to alter the order
of the maps themselves. So instead, we create a new map with the element types reversed, then copy