Example program - bank teller simulation – HP Integrity NonStop H-Series User Manual
Page 116
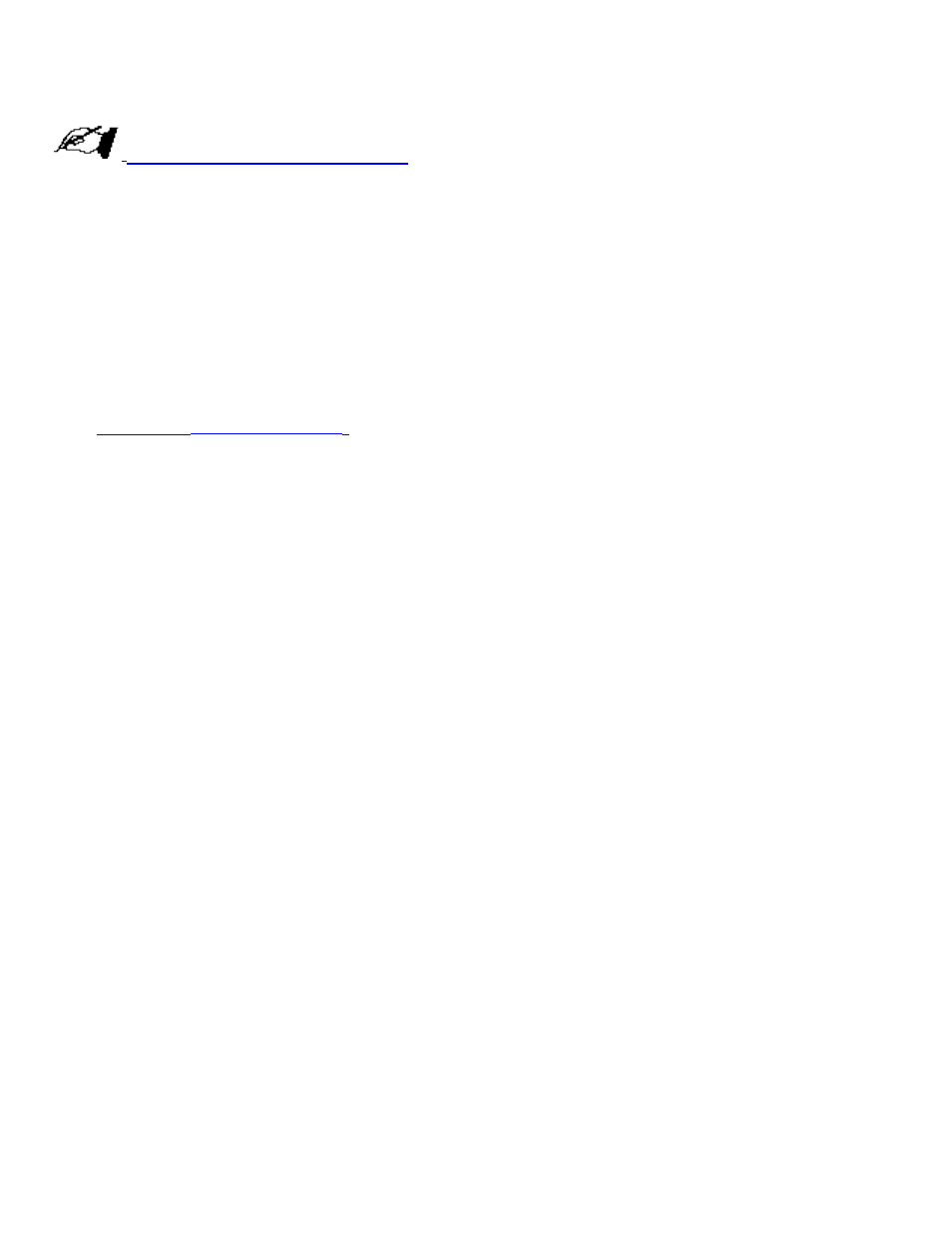
Example Program - Bank Teller Simulation
Obtaining the Sample Program
Queues are often found in businesses, such as supermarkets or banks. Suppose you are the manager
of a bank, and you need to determine how many tellers to have working during certain hours. You
decide to create a computer simulation, basing your simulation on certain observed behavior. For
example, you note that during peak hours there is a ninety percent chance that a customer will arrive
every minute.
We create a simulation by first defining objects to represent both customers and tellers. For
customers, the information we wish to know is the average amount of time they spend waiting in
line. Thus, customer objects simply maintain two integer data fields: the time they arrive in line, and
the time they will spend at the counter. The latter is a value randomly selected between 2 and 8.
(See
for a discussion of the randomInteger() function.)
class Customer {
public:
Customer (int at = 0) : arrival_Time(at),
processTime(2 + randomInteger(6)) {}
int arrival_Time;
int processTime;
bool done() // are we done with our transaction?
{ return --processTime < 0; }
operator < (const Customer & c) // order by arrival time
{ return arrival_Time < c.arrival_Time; }
operator == (const Customer & c) // no two customers are alike
{ return false; }
};
Because objects can only be stored in standard library containers if they can be compared for
equality and ordering, it is necessary to define the < and == operators for customers. Customers can
also tell us when they are done with their transactions.
Tellers are either busy servicing customers, or they are free. Thus, each teller value holds two data
fields; a customer, and a boolean flag. Tellers define a member function to answer whether they are
free or not, as well as a member function that is invoked when they start servicing a customer.
class Teller {
public:
Teller() { free = true; }
bool isFree() // are we free to service new customer?
{ if (free) return true;
if (customer.done())