Output iterators – HP Integrity NonStop H-Series User Manual
Page 28
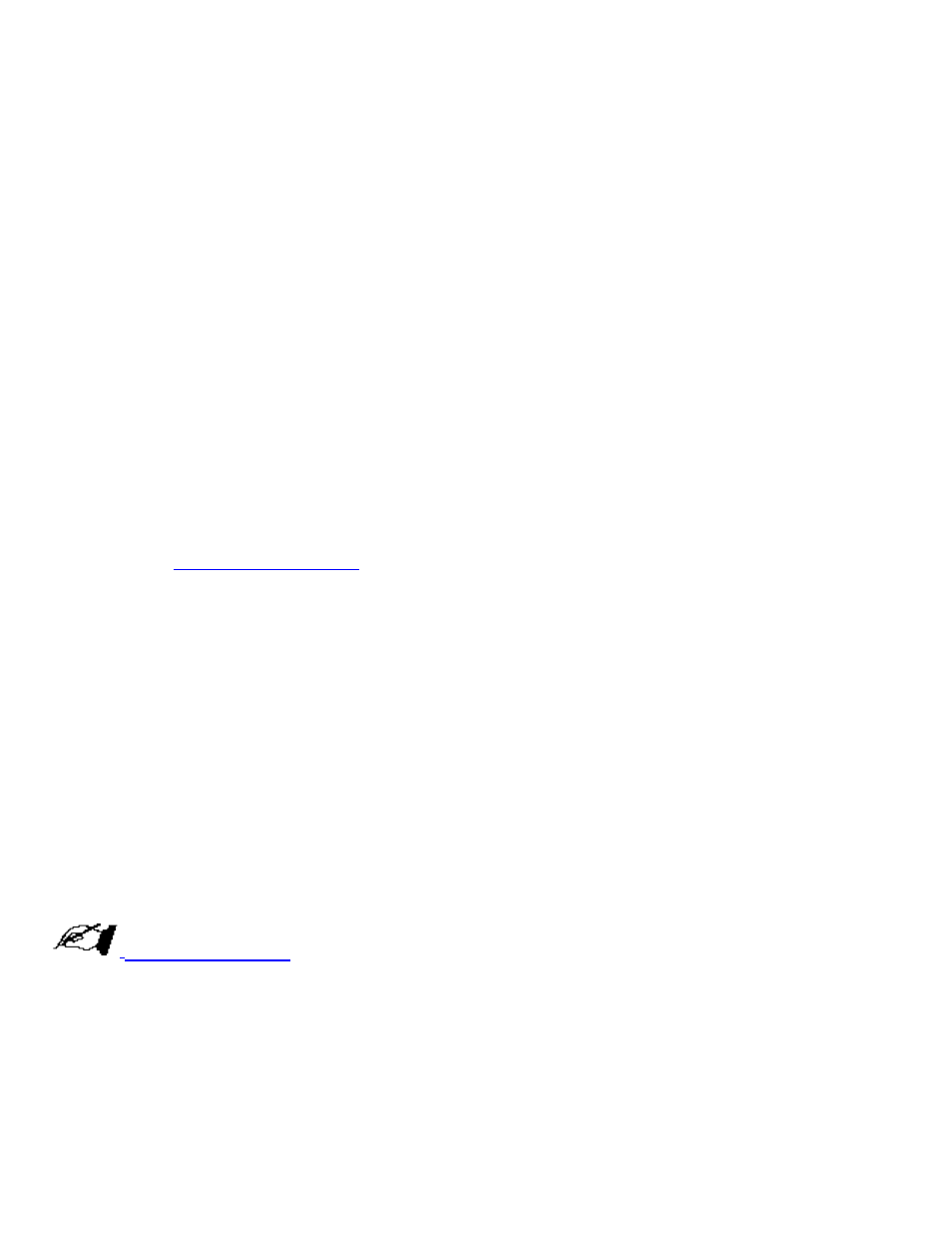
int * where = find(data, data+100, 7);
Note that constant pointers, pointers which do not permit the underlying array to be modified, can be
created by simply placing the keyword const in a declaration.
const int * first = data;
const int * last = data + 100;
// can't modify location returned by the following
const int * where = find(first, last, 7);
Container iterators. All of the iterators constructed for the various containers provided by the
standard library are at least as general as input iterators. The iterator for the first element in a
collection is always constructed by the member function begin(), while the iterator that denotes the
"past-the-end" location is generated by the member function end(). For example, the following
searches for the value 7 in a list of integers:
list
Each container that supports iterators provides a type within the class declaration with the name
iterator. Using this, iterators can uniformly be declared in the fashion shown. If the container being
accessed is constant, or if the description const_iterator is used, then the iterator is a constant iterator.
Input stream iterators. The standard library provides a mechanism to operate on an input stream
using an input iterator. This ability is provided by the class istream_iterator, and will be described in
more detail in
Output Iterators
An output iterator has the opposite function from an input iterator. Output iterators can be used to
assign values in a sequence, but cannot be used to access values. For example, we can use an output
iterator in a generic algorithm that copies values from one sequence into another:
template
OutputIterator copy
(InputIterator first, InputIterator last, OutputIterator result)
{
while (first != last)
*result++ = *first++;
return result;
}
Parallel Sequences
Two ranges are being manipulated here; the range of source values specified by a pair of input
iterators, and the destination range. The latter, however, is specified by only a single argument. It is
assumed that the destination is large enough to include all values, and errors will ensue if this is not
the case.
As illustrated by this algorithm, an output iterator can modify the element to which it points, by being
used as the target for an assignment. Output iterators can use the dereference operator only in this
fashion, and cannot be used to return or access the elements they denote.