Iterators, Insertion, removal and replacement, Copy and substring – HP Integrity NonStop H-Series User Manual
Page 134: Character access
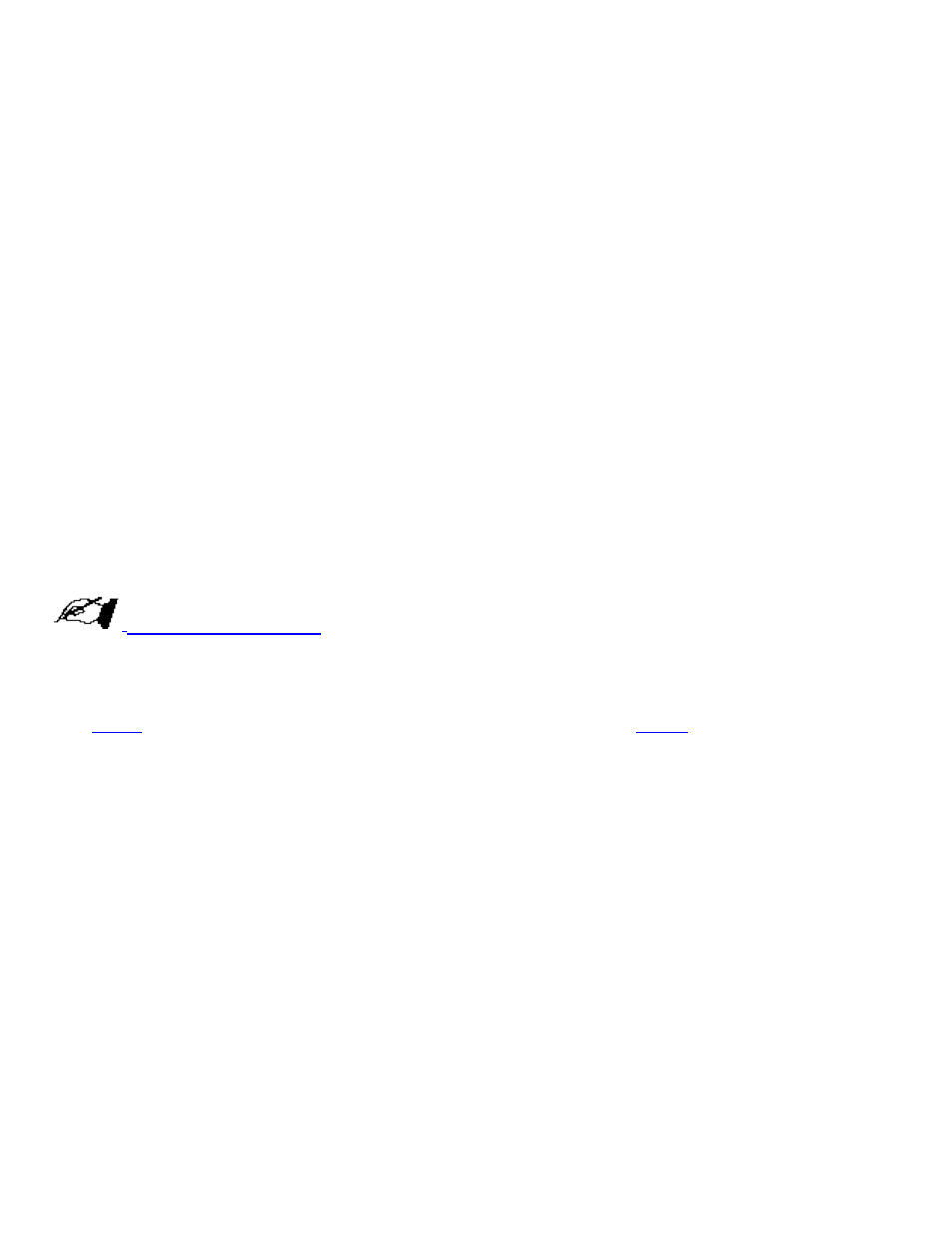
Character Access
An individual character from a string can be accessed or assigned using the subscript operator. The
member function at() is a synonym for this operation.
cout << s4[2] << endl; // output position 2 of s4
s4[2] = 'x'; // change position 2
cout << s4.at(2) << endl; // output updated value
The member function c_str() returns a pointer to a null terminated character array, whose elements
are the same as those contained in the string. This lets you use strings with functions that require a
pointer to a conventional C-style character array. The resulting pointer is declared as constant,
which means that you cannot use c_str() to modify the string. In addition, the value returned by
c_str() might not be valid after any operation that may cause reallocation (such as append() or
insert()). The member function data() returns a pointer to the underlying character buffer.
char d[256];
strcpy(d, s4.c_str()); // copy s4 into array d
Iterators
The member functions begin() and end() return beginning and ending random-access iterators for
the string. The values denoted by the iterators will be individual string elements. The functions
rbegin() and rend() return backwards iterators.
Invalidating Iterators
Insertion, Removal and Replacement
The
string
member functions insert() and remove() are similar to the
vector
functions insert() and
erase(). Like the vector versions, they can take iterators as arguments, and specify the insertion or
removal of the ranges specified by the arguments. The function replace() is a combination of
remove and insert, in effect replacing the specified range with new values.
s2.insert(s2.begin()+2, aList.begin(), aList.end());
s2.remove(s2.begin()+3, s2.begin()+5);
s2.replace(s2.begin()+3, s2.begin()+6, s3.begin(), s3.end());
In addition, the functions also have non-iterator implementations. The insert() member function
takes as argument a position and a string, and inserts the string into the given position. The remove
function takes two integer arguments, a position and a length, and removes the characters specified.
And the replace function takes two similar integer arguments as well as a string and an optional
length, and replaces the indicated range with the string (or an initial portion of a string, if the length
has been explicitly specified).
s3.insert (3, "abc"); //insert abc after position 3
s3.remove (4, 2); // remove positions 4 and 5
s3.replace (4, 2, "pqr"); //replace positions 4 and 5 with pqr