Replace certain elements with fixed value – HP Integrity NonStop H-Series User Manual
Page 158
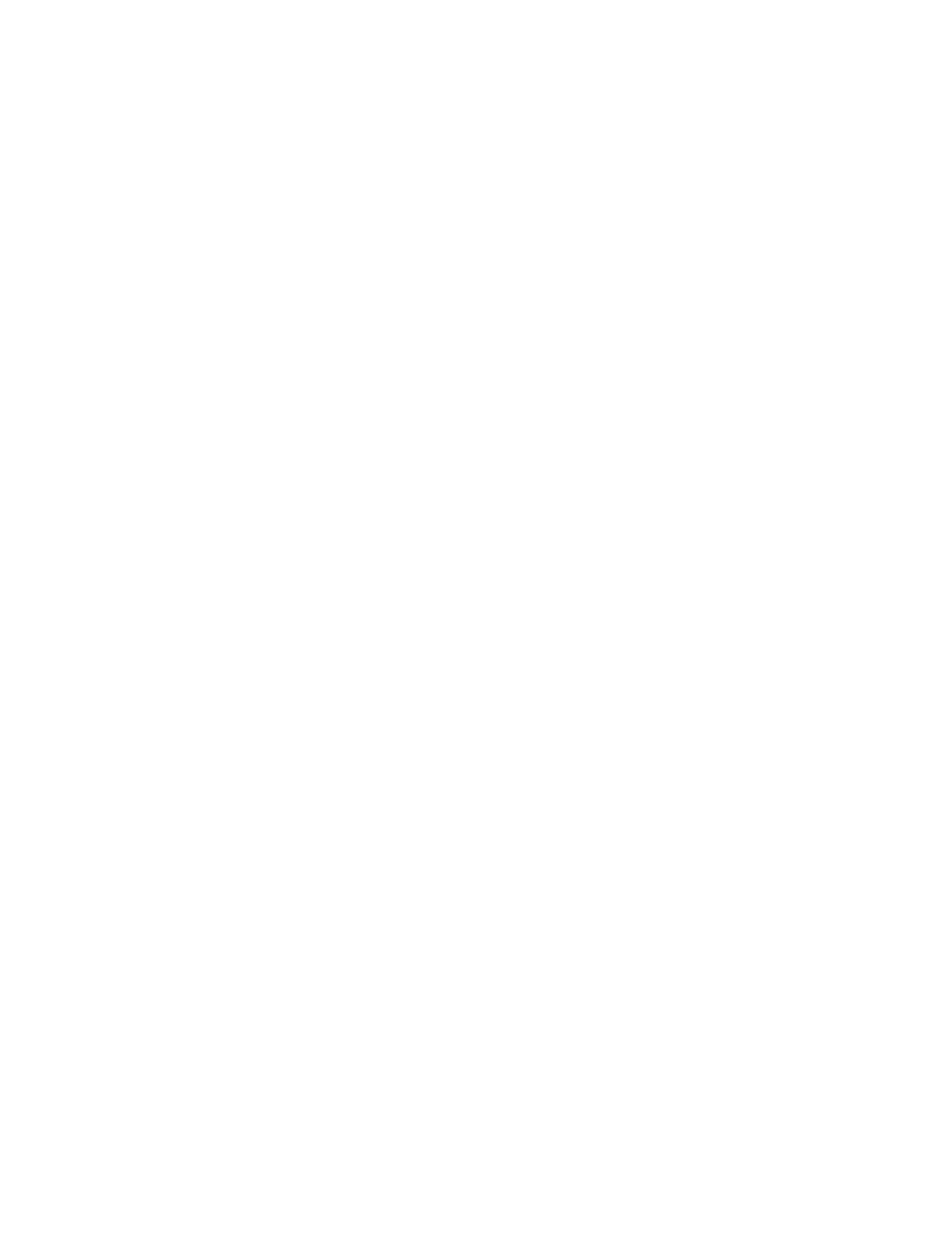
reverse (text, text + strlen(text));
cout << text << endl;
// example 2, reversing a list
list
generate_n (inserter(iList, iList.begin()), 10, iotaGen(2));
reverse (iList.begin(), iList.end());
}
Replace Certain Elements With Fixed Value
The algorithms replace() and replace_if() are used to replace occurrences of certain elements with a
new value. In both cases the new value is the same, no matter how many replacements are
performed. Using the algorithm replace(), all occurrences of a particular test value are replaced with
the new value. In the case of replace_if(), all elements that satisfy a predicate function are replaced
by a new value. The iterator arguments must be forward iterators.
The algorithms replace_copy() and replace_copy_if() are similar to replace() and replace_if(),
however they leave the original sequence intact and place the revised values into a new sequence,
which may be a different type.
void replace (ForwardIterator first, ForwardIterator last,
const T&, const T&);
void replace_if (ForwardIterator first, ForwardIterator last,
Predicate, const T&);
OutputIterator replace_copy (InputIterator, InputIterator,
OutputIterator, const T&, const T&);
OutputIterator replace_copy (InputIterator, InputIterator,
OutputIterator, Predicate, const T&);
In the example program, a vector is initially assigned the values 0 1 2 3 4 5 4 3 2 1 0. A call on
replace() replaces the value 3 with the value 7, resulting in the vector 0 1 2 7 4 5 4 7 2 1 0. The
invocation of replace_if() replaces all even numbers with the value 9, resulting in the vector 9 1 9 7
9 5 9 7 9 1 9.
void replace_example ()
// illustrate the use of the replace algorithm
{
// make vector 0 1 2 3 4 5 4 3 2 1 0
vector
for (int i = 0; i < 11; i++)
numbers[i] = i < 5 ? i : 10 - i;
// replace 3 by 7
replace (numbers.begin(), numbers.end(), 3, 7);
// replace even numbers by 9