Partition a sequence into two groups, Chapter 13, Partition a sequence – HP Integrity NonStop H-Series User Manual
Page 160
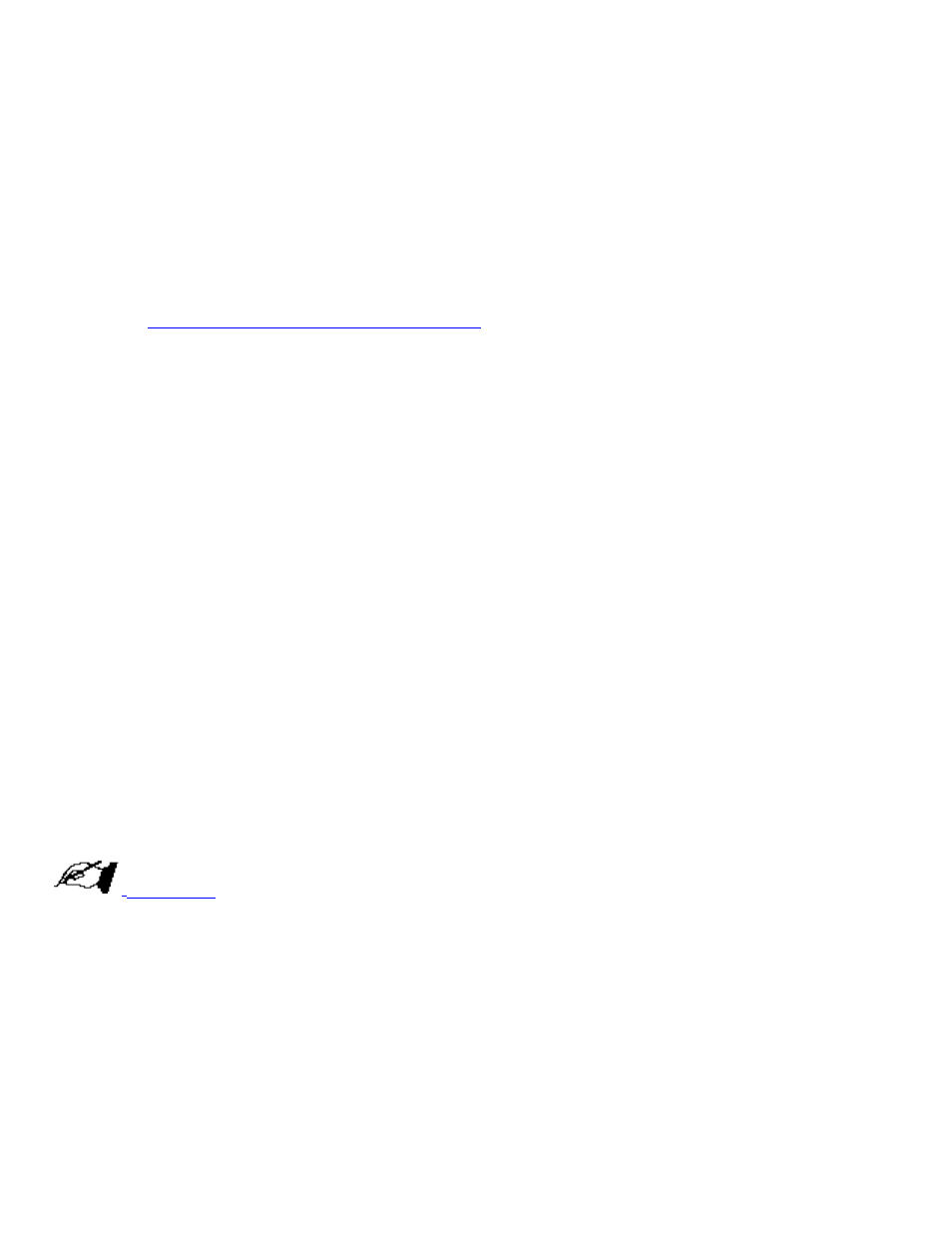
// now rotate around that location
rotate (iList.begin(), middle, iList.end());
// rotate again around the same location
list
rotate_copy (iList.begin(), middle, iList.end(),
inserter(cList, cList.begin()));
}
The example program first creates a list of the integers in order from 1 to 10. Next, the find()
algorithm (
Find an Element Satisfying a Condition
) is used to find the location of the element 7.
This is used as the midpoint for the rotation.
A second form of rotate() copies the elements into a new sequence, rather than rotating the values in
place. This is also shown in the example program, which once again rotates around the middle
position (now containing a 3). The resulting list is 3 4 5 6 7 8 9 10 1 2. The values held in iList
remain unchanged.
Partition a Sequence into Two Groups
A partition is formed by moving all the elements that satisfy a predicate to one end of a sequence,
and all the elements that fail to satisfy the predicate to the other end. Partitioning elements is a
fundamental step in certain sorting algorithms, such as "quicksort."
BidirectionalIterator partition
(BidirectionalIterator, BidirectionalIterator, Predicate);
BidirectionalIterator stable_partition
(BidirectionalIterator, BidirectionalIterator, Predicate);
There are two forms of partition supported in the standard library. The first, provided by the
algorithm partition(), guarantees only that the elements will be divided into two groups. The result
value is an iterator that describes the final midpoint between the two groups; it is one past the end of
the first group.
Partitions
In the example program the initial vector contains the values 1 to 10 in order. The partition moves
the even elements to the front, and the odd elements to the end. This results in the vector holding the
values 10 2 8 4 6 5 7 3 9 1, and the midpoint iterator pointing to the element 5.
void partition_example ()
// illustrate the use of the partition algorithm
{
// first make the vector 1 2 3 ... 10
vector
generate(numbers.begin(), numbers.end(), iotaGen(1));