Generate permutations in sequence, Chapter 13, Generate permutations in – HP Integrity NonStop H-Series User Manual
Page 161: Sequence
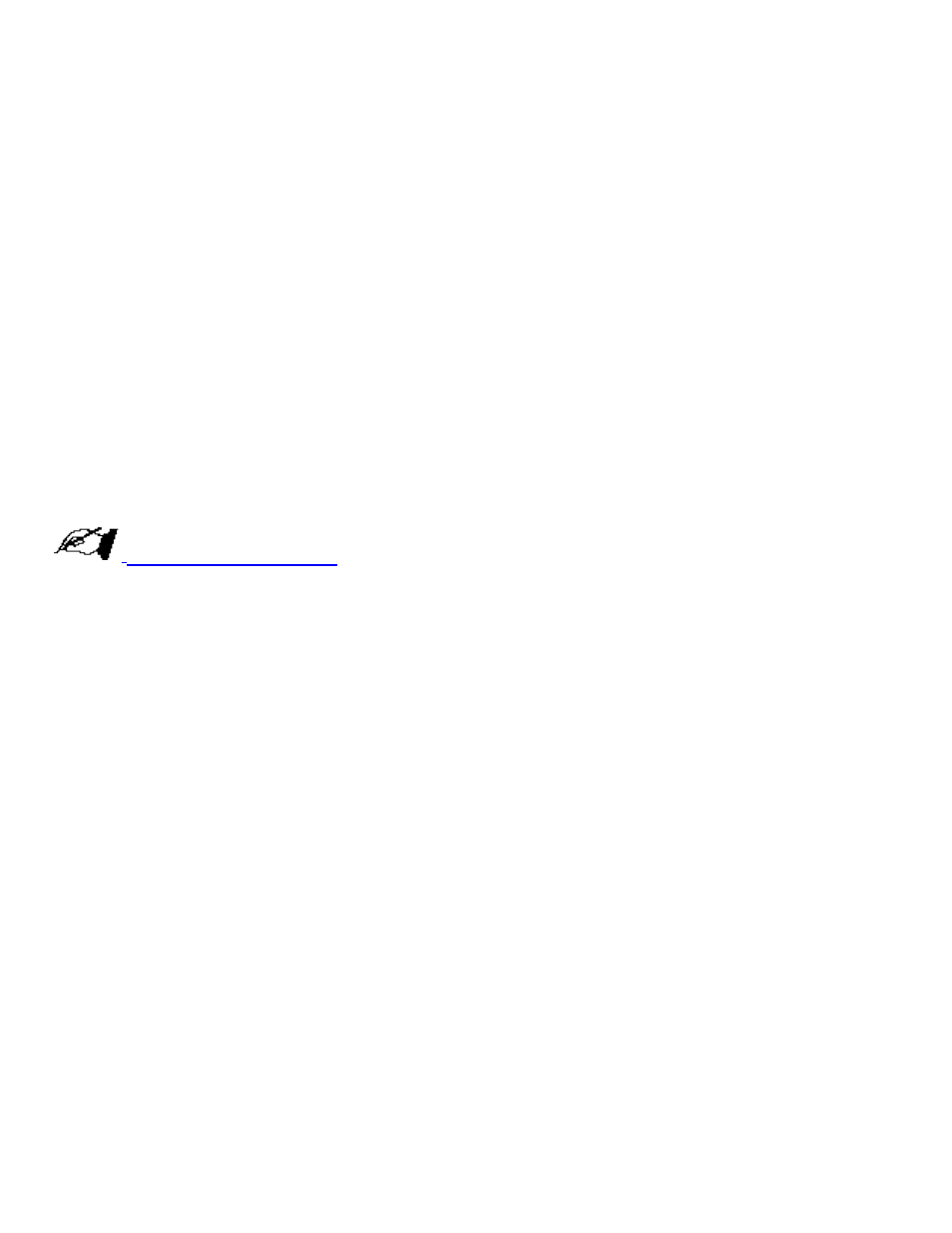
// now put the even values low, odd high
vector
partition(numbers.begin(), numbers.end(), isEven);
cout << "middle location " << result - numbers.begin() << endl;
// now do a stable partition
generate (numbers.begin(), numbers.end(), iotaGen(1));
stable_partition (numbers.begin(), numbers.end(), isEven);
}
The relative order of the elements within a partition in the resulting vector may not be the same as
the values in the original vector. For example, the value 4 preceded the element 8 in the original, yet
in the result it may follow the element 8. A second version of partition, named stable_partition(),
guarantees the ordering of the resulting values. For the sample input shown in the example, the
stable partition would result in the sequence 2 4 6 8 10 1 3 5 7 9. The stable_partition() algorithm is
slightly slower and uses more memory than the partition() algorithm, so when the order of elements
is not important you should use partition().
Generate Permutations in Sequence
Ordering Permutations
A permutation is a rearrangement of values. If values can be compared against each other (such as
integers, characters, or words) then it is possible to systematically construct all permutations of a
sequence. There are 2 permutations of two values, for example, and six permutations of three
values, and 24 permutations of four values.
The permutation generating algorithms have the following definition:
bool next_permutation (BidirectionalIterator first,
BidirectionalIterator last, [ Compare ] );
bool prev_permutation (BidirectionalIterator first,
BidirectionalIterator last, [ Compare ] );
The second example in the sample program illustrates the same idea, only using pointers to
character arrays instead of integers. In this case a different comparison function must be supplied,
since the default operator would simply compare pointer addresses.
bool nameCompare (char * a, char * b) { return strcmp(a, b) <= 0; }
void permutation_example ()
// illustrate the use of the next_permutation algorithm
{
// example 1, permute the values 1 2 3
int start [] = { 1, 2, 3};
do
copy (start, start + 3,
ostream_iterator