Randomly rearrange elements in a sequence – HP Integrity NonStop H-Series User Manual
Page 163
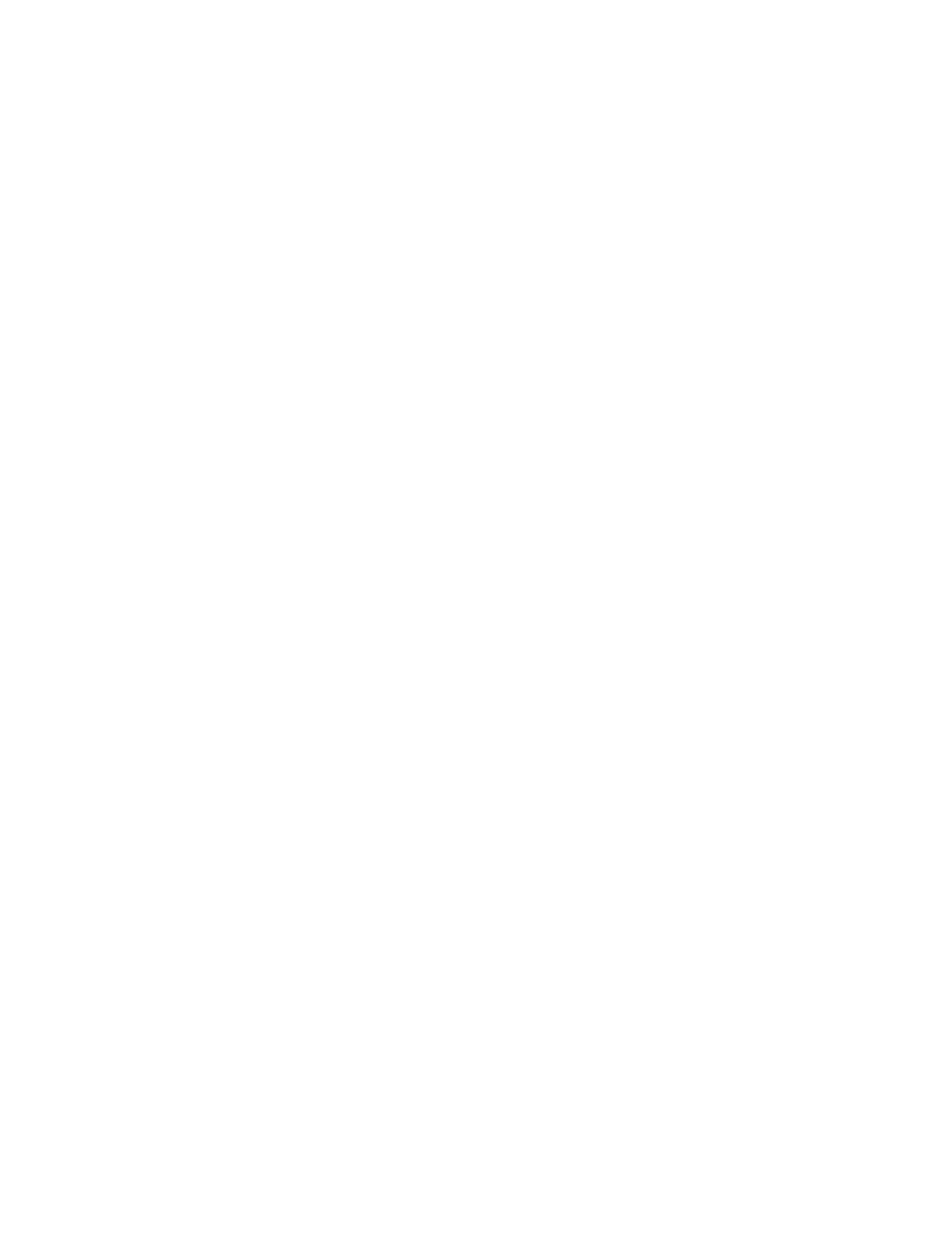
// then find the middle location
vector
find(numbers.begin(), numbers.end(), 1);
// copy them into a list
list
copy (numbers.begin(), numbers.end(),
inserter (numList, numList.begin()));
list
find(numList.begin(), numList.end, 1);
// now merge the lists into one
inplace_merge (numbers.begin(), midvec, numbers.end());
inplace_merge (numList.begin(), midList, numList.end());
}
Randomly Rearrange Elements in a Sequence
The algorithm random_shuffle() randomly rearranges the elements in a sequence. Exactly n swaps
are performed, where n represents the number of elements in the sequence. The results are, of
course, unpredictable. Because the arguments must be random access iterators, this algorithm can
only be used with vectors, deques, or ordinary pointers. It cannot be used with lists, sets, or maps.
void random_shuffle (RandomAccessIterator first,
RandomAccessIterator last [, Generator ] );
An alternative version of the algorithm uses the optional third argument. This value must be a
random number generator. This generator must take as an argument a positive value m and return a
value between 0 and m-1. As with the generate() algorithm, this random number function can be
any type of object that will respond to the function invocation operator.
void random_shuffle_example ()
// illustrate the use of the random_shuffle algorithm
{
// first make the vector containing 1 2 3 ... 10
vector
generate(numbers.begin(), numbers.end(), iotaGen(1));
// then randomly shuffle the elements
random_shuffle (numbers.begin(), numbers.end());
// do it again, with explicit random number generator
struct RandomInteger {
{
operator() (int m) { return rand() % m; }
} random;
random_shuffle (numbers.begin(), numbers.end(), random);