HP Integrity NonStop H-Series User Manual
Page 76
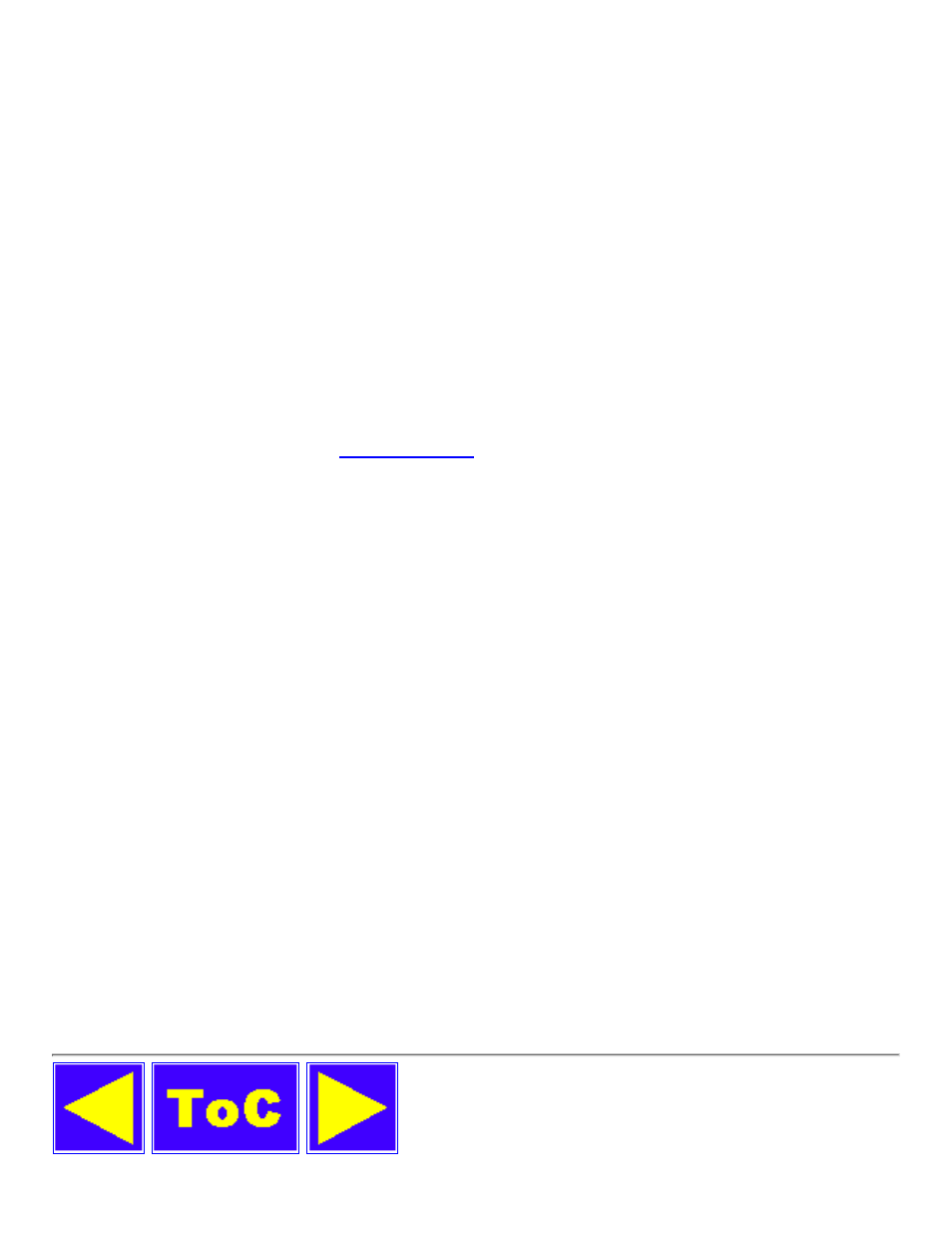
list
find (on_order.begin(), on_order.end(), wid);
if (weneed != on_order.end())
{
cout << "Ship " << Widget(wid)
<< " to fill back order" << endl;
on_order.erase(weneed);
}
else
on_hand.push_front(Widget(wid));
}
When a customer orders a new widget, we scan the list of widgets in stock to determine if the order can
be processed immediately. We can use the function find_if() to search the list. To do so we need a
binary function that takes as its argument a widget and determines whether the widget matches the type
requested. We can do this by taking a general binary widget-testing function, and binding the second
argument to the specific widget type. To use the function bind2nd(), however, requires that the binary
function be an instance of the class
binary_function
. The general widget-testing function is written as
follows:
class WidgetTester : public binary_function
public:
bool operator () (const Widget & wid, int testid) const
{ return wid.id == testid; }
};
The widget order function is then written as follows:
void inventory::order (int wid)
{
cout << "Received order for widget type " << wid << endl;
list
find_if (on_hand.begin(), on_hand.end(),
bind2nd(WidgetTester(), wid));
if (wehave != on_hand.end())
{
cout << "Ship " << *wehave << endl;
on_hand.erase(wehave);
}
else
{
cout << "Back order widget of type " << wid << endl;
on_order.push_front(wid);
}
}