Remove runs of similar values, Chapter 13, Remove overruns of similar values – HP Integrity NonStop H-Series User Manual
Page 167
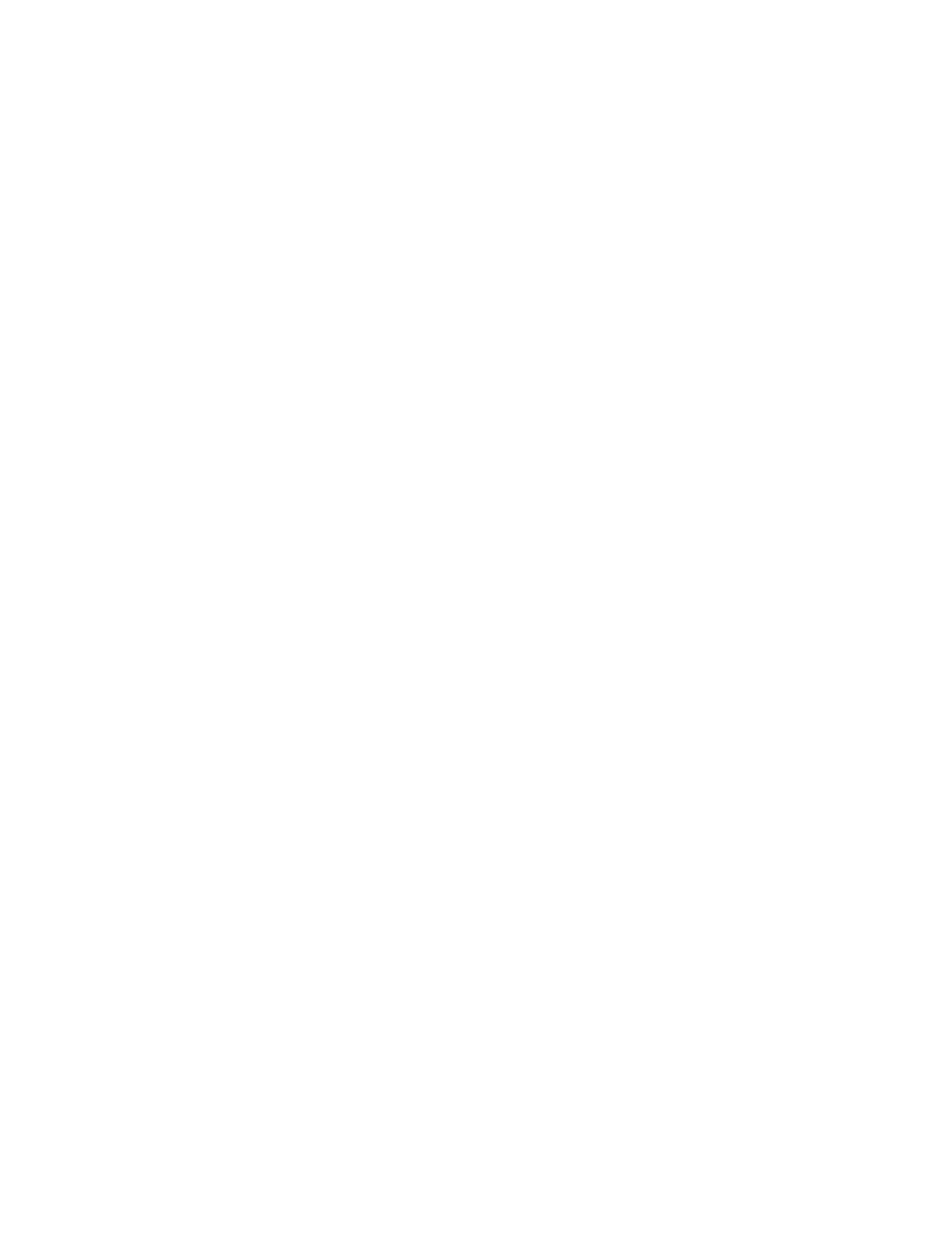
aList.erase(where, aList.end());
}
Remove Runs of Similar Values
The algorithm unique() moves through a linear sequence, eliminating all but the first element
from every consecutive group of equal elements. The argument sequence is described by
forward iterators.
ForwardIterator unique (ForwardIterator first,
ForwardIterator last [, BinaryPredicate ] );
As the algorithm moves through the collection, elements are moved to the front of the sequence,
overwriting the existing elements. Once all unique values have been identified, the remainder of
the sequence is left unchanged. For example, a sequence such as 1 3 3 2 2 2 4 will be changed
into 1 3 2 4 | 2 2 4. We have used a vertical bar to indicate the location returned by the iterator
result value. This location marks the end of the unique sequence, and the beginning of the
left-over elements. With most containers the value returned by the algorithm can be used as an
argument in a subsequent call on erase() to remove the undesired elements from the collection.
This is illustrated in the example program.
A copy version of the algorithm moves the unique values to an output iterator, rather than
making modifications in place. In transforming a list or multiset, an insert iterator can be used
to change the copy operations of the output iterator into insertions.
OutputIterator unique_copy
(InputIterator first, InputIterator last,
OutputIterator result [, BinaryPredicate ] );
These are illustrated in the sample program:
void unique_example ()
// illustrate use of the unique algorithm
{
// first make a list of values
int data[] = {1, 3, 3, 2, 2, 4};
list
set
copy (data, data+6, inserter(aList, aList.begin()));
// copy unique elements into a set
unique_copy (aList.begin(), aList.end(),
inserter(aSet, aSet.begin()));
// copy unique elements in place
list
where = unique(aList.begin(), aList.end());