Find consecutive duplicate elements, Find an element satisfying a condition – HP Integrity NonStop H-Series User Manual
Page 151
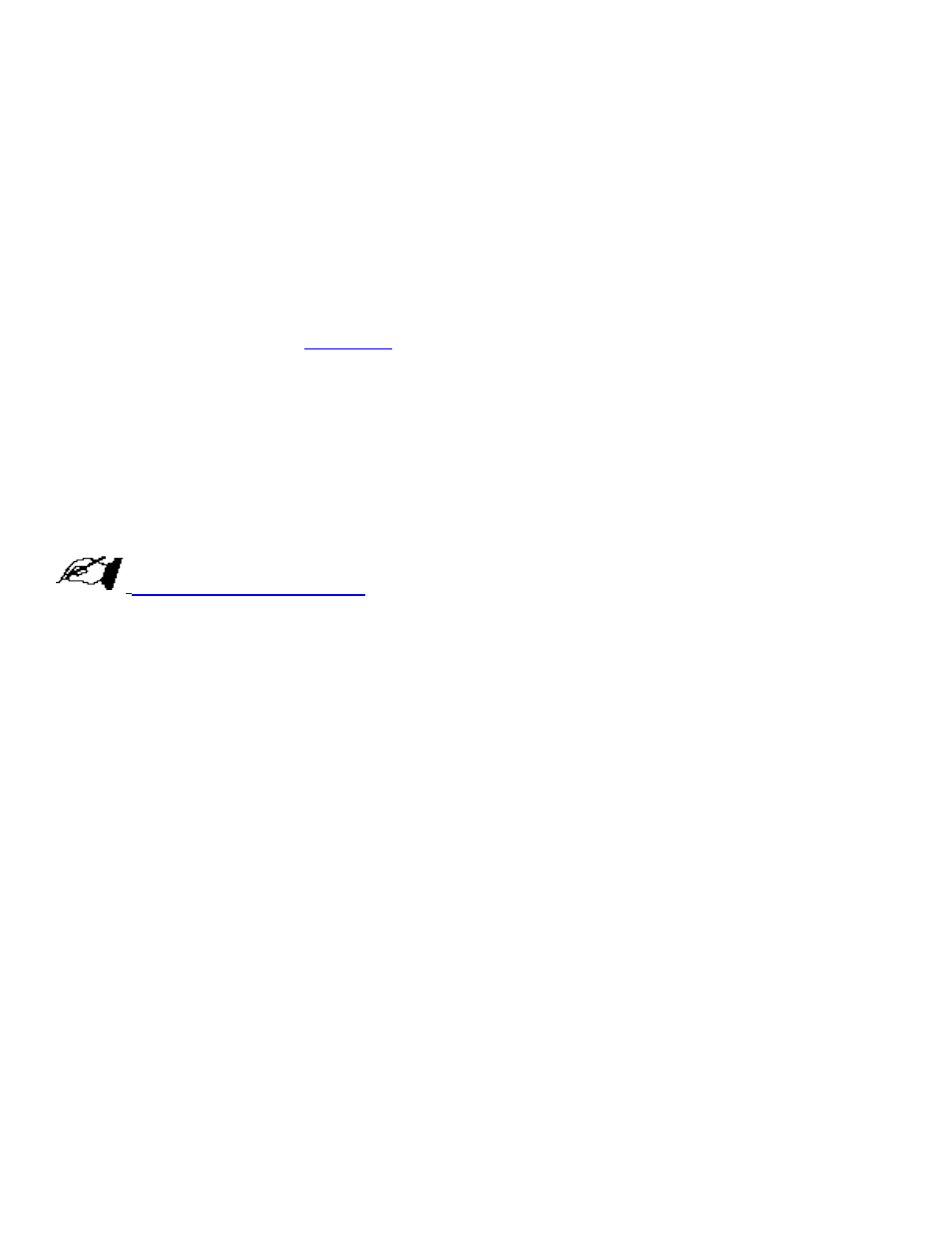
Find an Element Satisfying a Condition
There are two algorithms, find() and find_if(), that are used to find the first element that
satisfies a condition. The declarations of these two algorithms are as follows:
InputIterator find_if (InputIterator first, InputIterator last,
Predicate);
InputIterator find (InputIterator first, InputIterator last,
const T&);
The algorithm find_if() takes as argument a predicate function, which can be any function that
returns a boolean value (see
). The find_if() algorithm returns a new iterator that
designates the first element in the sequence that satisfies the predicate. The second argument,
the past-the-end iterator, is returned if no element is found that matches the requirement.
Because the resulting value is an iterator, the dereference operator (the * operator) must be used
to obtain the matching value. This is illustrated in the example program.
The second form of the algorithm, find(), replaces the predicate function with a specific value,
and returns the first element in the sequence that tests equal to this value, using the appropriate
equality operator (the == operator) for the given data type.
Searching Sets and Maps
The following example program illustrates the use of these algorithms:
void find_test ()
// illustrate the use of the find algorithm
{
int vintageYears[] = {1967, 1972, 1974, 1980, 1995};
int * start = vintageYears;
int * stop = start + 5;
int * where = find_if (start, stop, isLeapYear);
if (where != stop)
cout << "first vintage leap year is " << *where << endl;
else
cout << "no vintage leap years" << endl;
where = find(start, stop, 1995);
if (where != stop)
cout << "1995 is position " << where - start
<< " in sequence" << endl;
else
cout "1995 does not occur in sequence" << endl;
}