HP Integrity NonStop H-Series User Manual
Page 154
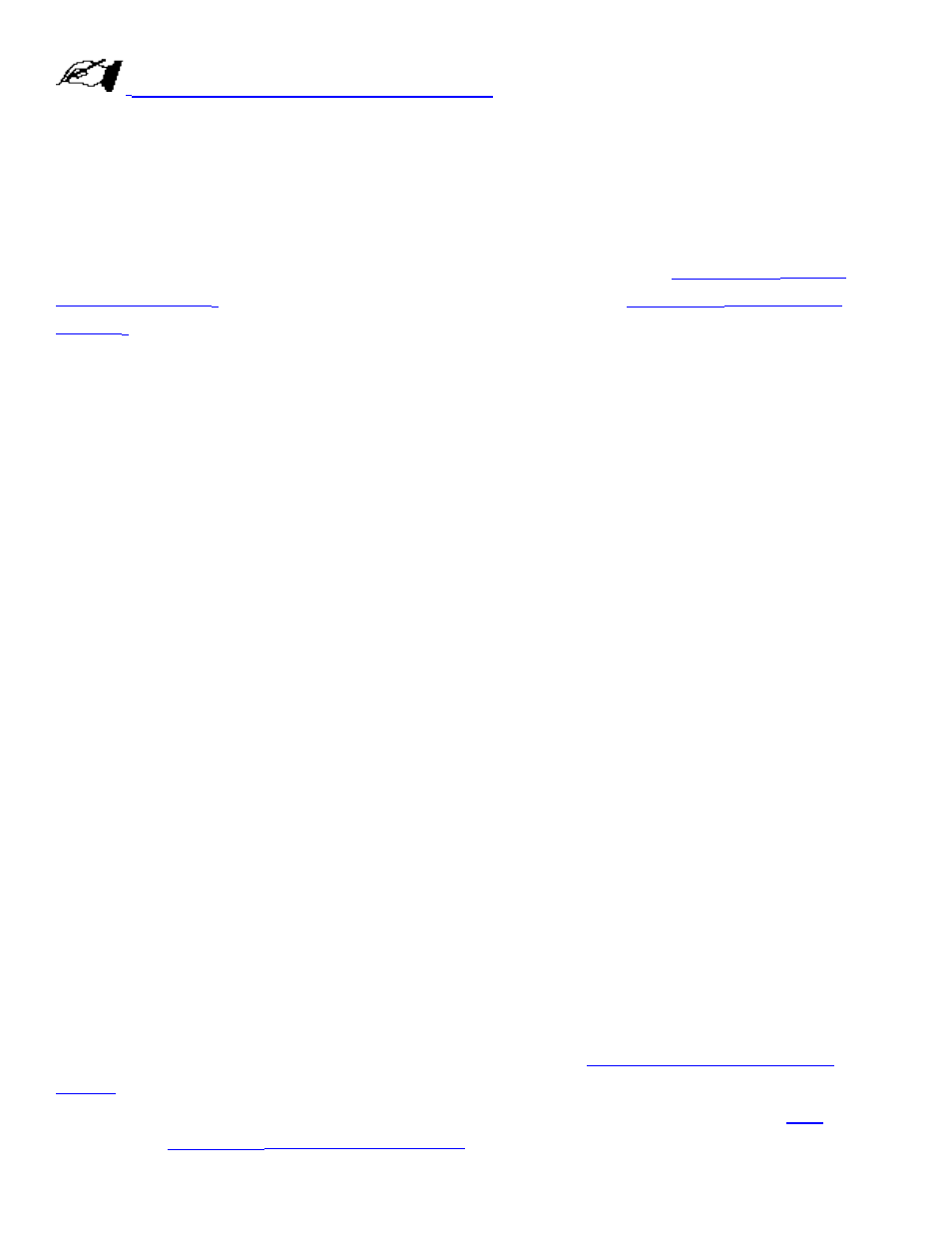
Largest and Smallest Elements of a Set
These algorithms return an iterator that denotes the largest or smallest of the values in a
sequence, respectively. Should more than one value satisfy the requirement, the result yielded is
the first satisfactory value. Both algorithms can optionally take a third argument, which is the
function to be used as the comparison operator in place of the default operator.
The example program illustrates several uses of these algorithms. The function named split()
used to divide a string into words in the string example is described in
. The function randomInteger() is described in
void max_min_example ()
// illustrate use of max_element and min_element algorithms
{
// make a vector of random numbers between 0 and 99
vector
for (int i = 0; i < 25; i++)
numbers[i] = randomInteger(100);
// print the maximum
vector
max_element(numbers.begin(), numbers.end());
cout << "largest value was " << * max << endl;
// example using strings
string text =
"It was the best of times, it was the worst of times.";
list
split (text, " .,!:;", words);
cout << "The smallest word is "
<< * min_element(words.begin(), words.end())
<< " and the largest word is "
<< * max_element(words.begin(), words.end())
<< endl;
}
Locate the First Mismatched Elements in Parallel Sequences
The name mismatch() might lead you to think this algorithm was the inverse of the equal()
algorithm, which determines if two sequences are equal (see
). Instead, the mismatch() algorithm returns a pair of iterators that together indicate the
first positions where two parallel sequences have differing elements. (The structure
pair
is
described in