Random access iterators – HP Integrity NonStop H-Series User Manual
Page 30
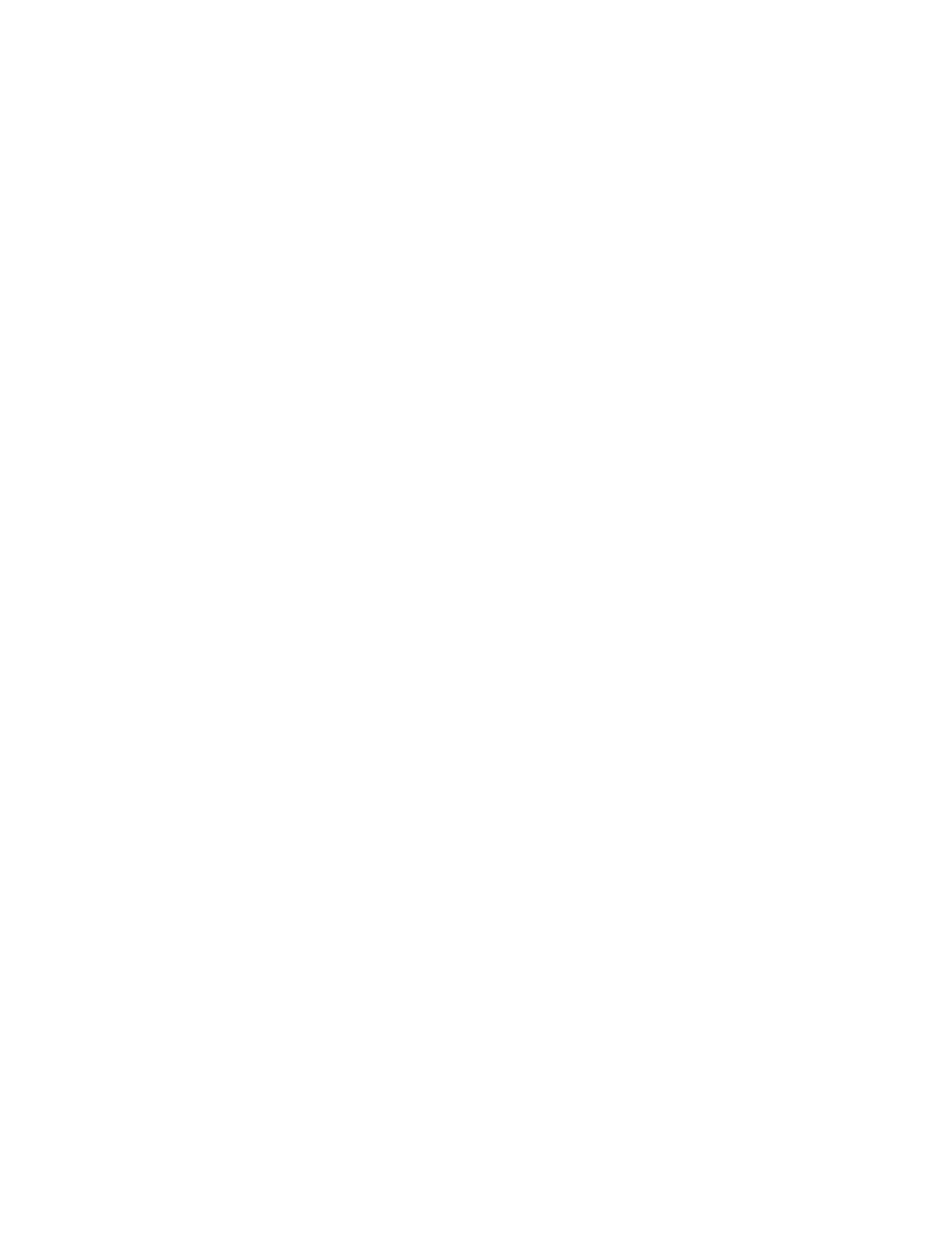
through the elements of a container. For example, we can use bidirectional iterators in a function that
reverses the values of a container, placing the results into a new container.
template
OutputIterator
reverse_copy (BidirectionalIterator first,
BidirectionalIterator last,
OutputIterator result)
{
while (first != last)
*result++ = *--last;
return result;
}
As always, the value initially denoted by the last argument is not considered to be part of the
collection.
The reverse_copy() function could be used, for example, to reverse the values of a linked list, and
place the result into a vector:
list
....
vector
reverse_copy (aList.begin(), aList.end(), aVec.begin() );
Random Access Iterators
Some algorithms require more functionality than the ability to access values in either a forward or
backward direction. Random access iterators permit values to be accessed by subscript, subtracted
one from another (to yield the number of elements between their respective values) or modified by
arithmetic operations, all in a manner similar to conventional pointers.
When using conventional pointers, arithmetic operations can be related to the underlying memory;
that is, x+10 is the memory ten elements after the beginning of x. With iterators the logical meaning
is preserved (x+10 is the tenth element after x), however the physical addresses being described may
be different.
Algorithms that use random access iterators include generic operations such as sorting and binary
search. For example, the following algorithm randomly shuffles the elements of a container. This is
similar to, although simpler than, the function random_shuffle() provided by the standard library.
template
void
mixup (RandomAccessIterator first, RandomAccessIterator last)
{
while (first < last)
{
iter_swap(first, first + randomInteger(last - first));
++first;
}
}