Extent and size-changing operations, Access and iteration, Test for inclusion – HP Integrity NonStop H-Series User Manual
Page 72
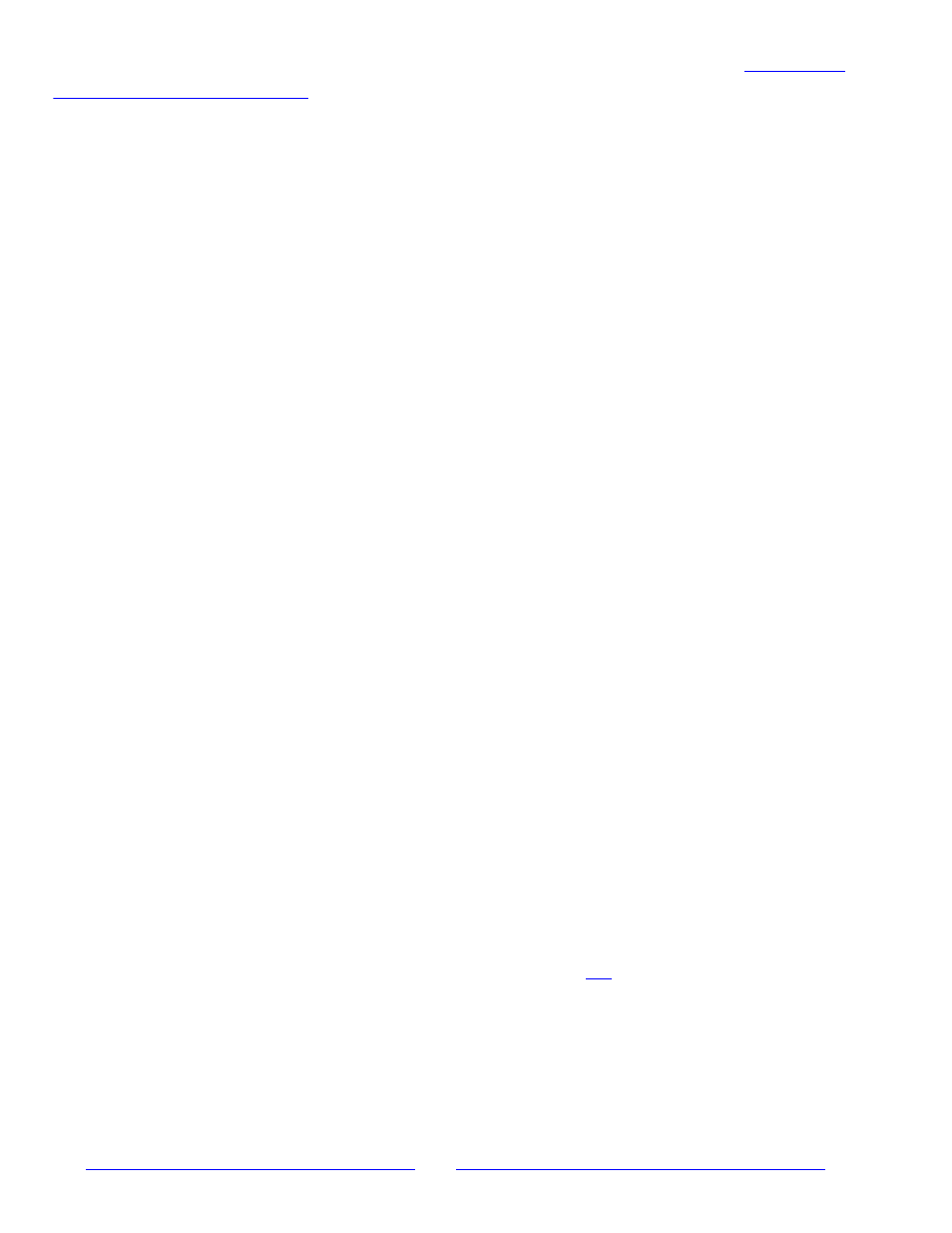
unique(). In this case the more general unique() generic algorithm can be used (see
Remove Overruns of Similar Values
). In the following example the binary function is the greater-than
operator, which will remove all elements smaller than a preceding element.
// remove first from consecutive equal elements
list_nine.unique();
// explicitly give comparison function
list_nine.unique(greater
// the following is equivalent to the above
location3 =
unique(list_nine.begin(), list_nine.end(), greater
list_nine.erase(location3, list_nine.end());
Extent and Size-Changing Operations
The member function size() will return the number of elements being held by a container. The
function empty() will return true if the container is empty, and is more efficient than comparing the
size against the value zero.
cout << "Number of elements: " << list_nine.size () << endl;
if ( list_nine.empty () )
cout << "list is empty " << endl;
else
cout << "list is not empty " << endl;
The member function resize() changes the size of the list to the value specified by the argument.
Values are either added or erased from the end of the collection as necessary. An optional second
argument can be used to provide the initial value for any new elements added to the collection.
// become size 12, adding values of 17 if necessary
list_nine.resize (12, 17);
Access and Iteration
The member functions front() and back() return, but do not remove, the first and last items in the
container, respectively. For a list, access to other elements is possible only by removing elements
(until the desired element becomes the front or back) or through the use of iterators.
There are three types of iterators that can be constructed for lists. The functions begin() and end()
construct iterators that traverse the list in forward order. For the
list
data type begin() and end() create
bidirectional iterators. The alternative functions rbegin() and rend() construct iterators that traverse in
reverse order, moving from the end of the list to the front.
Test for Inclusion
The list data types do not directly provide any method that can be used to determine if a specific
value is contained in the collection. However, either the generic algorithms find() or count() (Chapter
13: