Inserting and removing elements – HP Integrity NonStop H-Series User Manual
Page 59
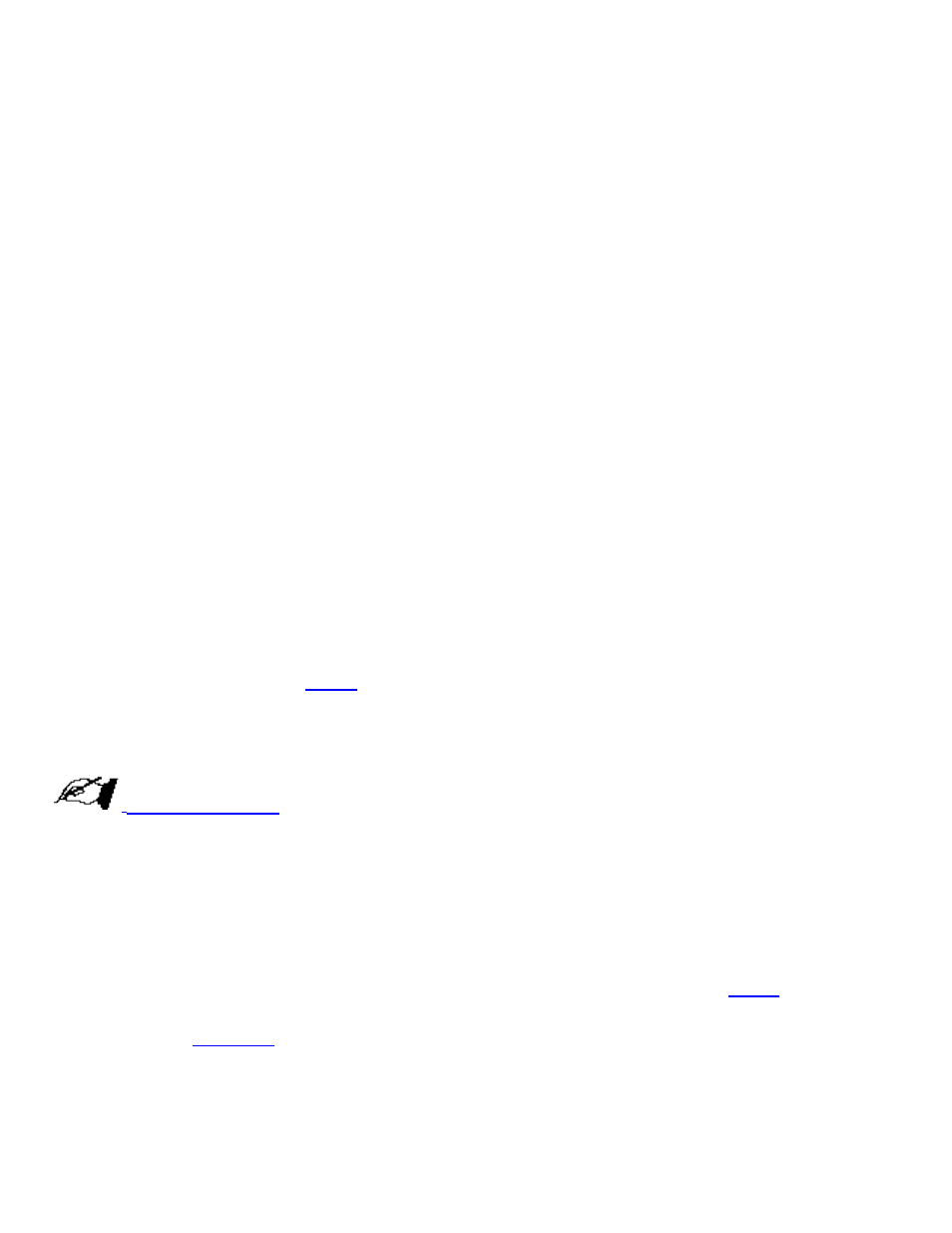
with reserve() is larger than the current capacity, then a reallocation occurs and the argument value
becomes the new capacity. (It may subsequently grow even larger; the value given as the argument
need not be a bound, just a guess.) If the capacity is already in excess of the argument, then no
reallocation takes place. Invoking reserve() does not change the size of the vector, nor the element
values themselves (with the exception that they may potentially be moved should reallocation take
place).
vec_five.reserve(20);
A reallocation invalidates all references, pointers, and iterators referring to elements being held by a
vector.
The member function empty() returns true if the vector currently has a size of zero (regardless of the
capacity of the vector). Using this function is generally more efficient than comparing the result
returned by size() to zero.
cout << "empty is " << vec_five.empty() << endl;
The member function resize() changes the size of the vector to the value specified by the argument.
Values are either added to or erased from the end of the collection as necessary. An optional second
argument can be used to provide the initial value for any new elements added to the collection. If a
destructor is defined for the element type, the destructor will be called for any values that are
removed from the collection.
// become size 12, adding values of 17 if necessary
vec_five.resize (12, 17);
Inserting and Removing Elements
As we noted earlier, the class
vector
differs from an ordinary array in that a vector can, in certain
circumstances, increase or decrease in size. When an insertion causes the number of elements being
held in a vector to exceed the capacity of the current block of memory being used to hold the
values, then a new block is allocated and the elements are copied to the new storage.
Costly Insertions
A new element can be added to the back of a vector using the function push_back(). If there is space
in the current allocation, this operation is very efficient (constant time).
vec_five.push_back(21); // add element 21 to end of collection
The corresponding removal operation is pop_back(), which decreases the size of the vector, but
does not change its capacity. If the container type defines a destructor, the destructor will be called
on the value being eliminated. Again, this operation is very efficient. (The class
deque
permits
values to be added and removed from both the back and the front of the collection. These functions
are described in
, which discusses deques in more detail.)
More general insertion operations can be performed using the insert() member function. The
location of the insertion is described by an iterator; insertion takes place immediately preceding the
location denoted. A fixed number of constant elements can be inserted by a single function call. It is
much more efficient to insert a block of elements in a single call, than to perform a sequence of