Generalized inner product, Chapter 13, Generalized inner – HP Integrity NonStop H-Series User Manual
Page 170: Product
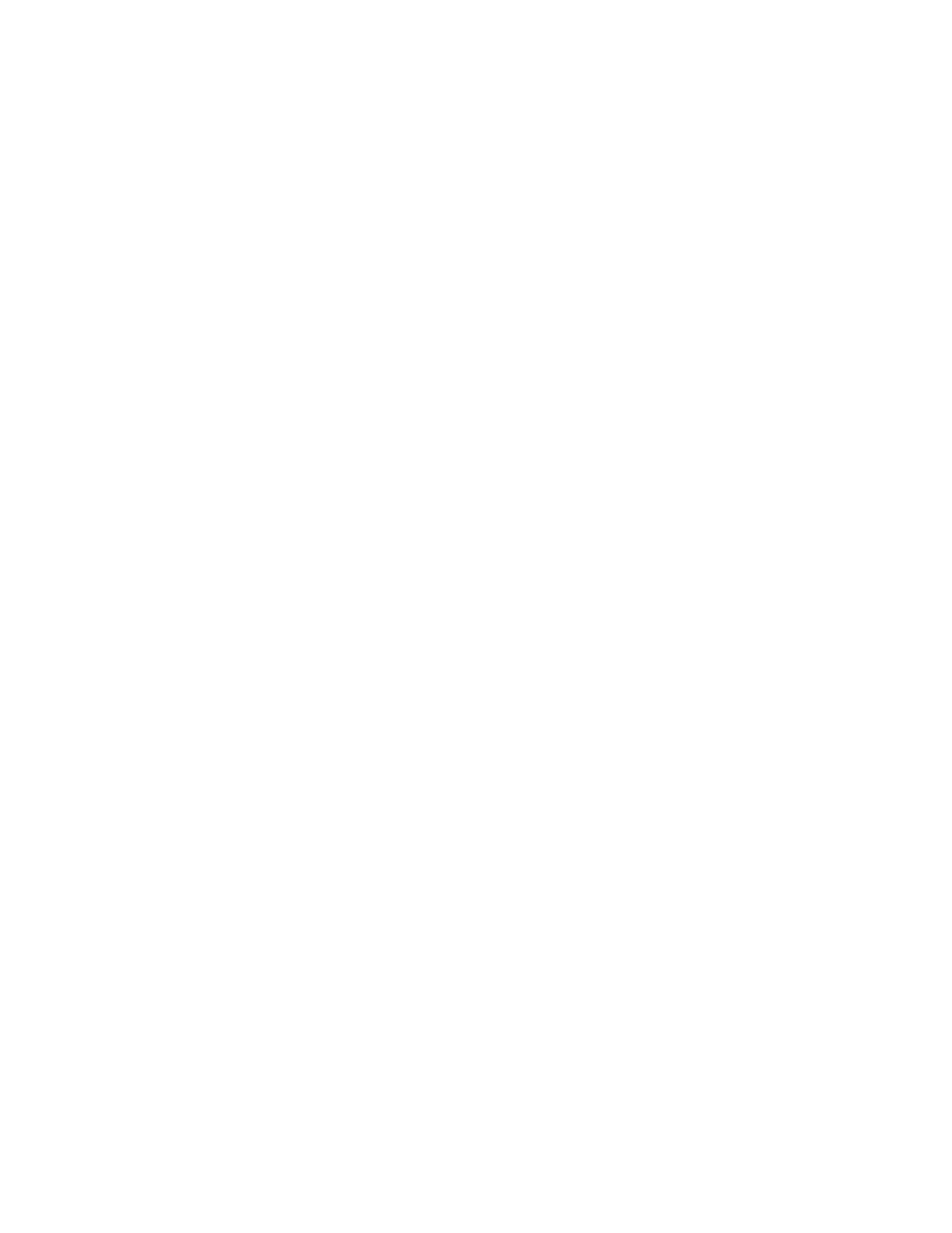
first case the identity is zero, and the default operator + is used. In the second invocation the identity is 1, and the
multiplication operator (named times) is explicitly passed as the fourth argument.
void accumulate_example ()
// illustrate the use of the accumulate algorithm
{
int numbers[] = {1, 2, 3, 4, 5};
// first example, simple accumulation
int sum = accumulate (numbers, numbers + 5, 0);
int product =
accumulate (numbers, numbers + 5, 1, times
cout << "The sum of the first five integers is " << sum << endl;
cout << "The product is " << product << endl;
// second example, with different types for initial value
list
nums = accumulate (numbers, numbers+5, nums, intReplicate);
}
list
// add sequence n to 1 to end of list
{
while (n) nums.push_back(n--);
return nums;
}
Neither the identity value nor the result of the binary function are required to match the container type. This is illustrated in
the example program by the invocation of accumulate() shown in the second example above. Here the identity is an empty
list. The function (shown after the example program) takes as argument a list and an integer value, and repeatedly inserts
values into the list. The values inserted represent a decreasing sequence from the argument down to 1. For the example
input (the same vector as in the first example), the resulting list contains the 15 values 1 2 1 3 2 1 4 3 2 1 5 4 3 2 1.
Generalized Inner Product
Assume we have two sequences of n elements each; a1, a2, ... an and b1, b2, ... bn. The inner product of the sequences is
the sum of the parallel products, that is the value a1 * b1 + a2 * b2 + ... + an * bn. Inner products occur in a number of
scientific calculations. For example, the inner product of a row times a column is the heart of the traditional matrix
multiplication algorithm. A generalized inner product uses the same structure, but permits the addition and multiplication
operators to be replaced by arbitrary binary functions. The standard library includes the following algorithm for computing
an inner product:
ContainerType inner_product
(InputIterator first1, InputIterator last1,
InputIterator first2, ContainerType initialValue
[ , BinaryFunction add, BinaryFunction times ] );
The first three arguments to the inner_product() algorithm define the two input sequences. The second sequence is specified
only by the beginning iterator, and is assumed to contain at least as many elements as the first sequence. The next argument
is an initial value, or identity, used for the summation operator. This is similar to the identity used in the accumulate()
algorithm. In the generalized inner product function the last two arguments are the binary functions that are used in place of
the addition operator, and in place of the multiplication operator, respectively.
In the example program the second invocation illustrates the use of alternative functions as arguments. The multiplication is
replaced by an equality test, while the addition is replaced by a logical or. The result is true if any of the pairs are equal, and
false otherwise. Using an and in place of the or would have resulted in a test which was true only if all pairs were equal; in
effect the same as the equal() algorithm described in the next section.
void inner_product_example ()
// illustrate the use of the inner_product algorithm
{
int a[] = {4, 3, -2};
int b[] = {7, 3, 2};